Fix `async Fn` confirmation for `FnDef`/`FnPtr`/`Closure` types
Fixes three issues:
1. The code in `extract_tupled_inputs_and_output_from_async_callable` was accidentally getting the *future* type and the *output* type (returned by the future) messed up for fnptr/fndef/closure types. :/
2. We have a (class of) bug(s) in the old solver where we don't really support higher ranked built-in `Future` goals for generators. This is not possible to hit on stable code, but [can be hit with `unboxed_closures`](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2021&gist=e935de7181e37e13515ad01720bcb899) (#121653).
* I'm opting not to fix that in this PR. Instead, I just instantiate placeholders when confirming `async Fn` goals.
4. Fixed a bug when generating `FnPtr` shims for `async Fn` trait goals.
r? oli-obk
Fix typo in `rustc_passes/messages.ftl`
Line 190 contains unpaired parentheses:
```
passes_doc_cfg_hide_takes_list =
`#[doc(cfg_hide(...)]` takes a list of attributes
```
The `#[doc(cfg_hide(...)]` contains unpaired parentheses. This PR changes it to `#[doc(cfg_hide(...))]`, which made the parentheses paired.
Deeply normalize obligations in `refining_impl_trait`
We somewhat awkwardly use semantic comparison when checking the `refining_impl_trait` lint. This relies on us being able to normalize bounds eagerly to avoid cases where an unnormalized alias is not considered equal to a normalized alias. Since `normalize` in the new solver is a noop, let's use `deeply_normalize` instead.
r? lcnr
cc ``@tmandry,`` this should fix your bug lol
Opportunistically resolve regions when processing region outlives obligations
Due to the matching in `TypeOutlives` being structural, we should attempt to opportunistically resolve regions before processing region obligations. Thanks ``@lcnr`` for finding this.
r? lcnr
Use `LitKind::Err` for malformed floats
#121120 changed `StringReader::cook_lexer_literal` to return `LitKind::Err` for malformed integer literals. This commit does the same for float literals, for consistency.
r? ``@fmease``
[rustdoc] Prevent inclusion of whitespace character after macro_rules ident
Discovered this bug randomly when looking at:
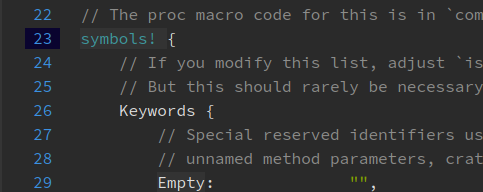
We were too eagerly trying to merge tokens that shouldn't be merged together (for example if you have a code comment followed by a code comment, we merge them in one attribute to reduce the DOM size).
r? ``@notriddle``
Diagnostic renaming
Renaming various diagnostic types from `Diagnostic*` to `Diag*`. Part of https://github.com/rust-lang/compiler-team/issues/722. There are more to do but this is enough for one PR.
r? `@davidtwco`
Process alias-relate obligations in CoerceUnsized loop
After #119106, we now emit `AliasRelate` goals when relating `?0` and `Alias<T, ..>` in the new solver. In the ad-hoc `CoerceUnsized` selection loop, we now may have `AliasRelate` goals which must be processed to constrain type variables which are mentioned in other goals.
---
For example, in the included test, we try to coerce `&<ManuallyDrop<T> as Deref>::Target` to `&dyn Foo`. This requires proving:
* 1 `&<ManuallyDrop<T> as Deref>::Target: CoerceUnsized<&dyn Foo>`
* 2 `<ManuallyDrop<T> as Deref>::Target alias-relate ?0`
* 3 `?0: Unsize<dyn Foo>`
* 4 `?0: Foo`
* 5 `?0: Sized`
If we don't process goal (2.) before processing goal (3.), then we hit ambiguity since `?0` is never constrained, and therefore we bail out, refusing to coerce the types. After processing (2.), we know `?0 := T`, and the rest of the goals can be processed normally.
Adjust printing for RPITITs
1. Call RPITITs `{synthetic#N}` instead of `{opaque#N}`.
2. Fall back to printing the RPITIT like an opaque even when printed as an `AliasTy`, just like we do for `ty::Alias`.
You could argue that (2.) is misleading, but I believe it's more consistent than naming `{synthetic#N}`, which I assume approximately nobody knows where that def path name comes from.
r? lcnr
Fix link generation for foreign macro in jump to definition feature
The crate name is already added to the link so it shouldn't be added a second time for local foreign macros.
r? ``@notriddle``
unix_sigpipe: Simple fixes and improvements in tests
In https://github.com/rust-lang/rust/pull/120832 I included 5 preparatory commits.
It will take a while before discussions there and in https://github.com/rust-lang/rust/issues/62569 is settled, so here is a PR that splits out 4 of the commits that are easy to review, to get them out of the way.
r? ``@davidtwco`` who already approved these commits in https://github.com/rust-lang/rust/pull/120832 (but I have tweaked them a bit and rebased them since then).
For the convenience of my reviewer, here are the full commit messages of the commits:
<details>
<summary>Click to expand</summary>
```
commit 948b1d68ab (HEAD -> unix_sigpipe-tests-fixes, origin/unix_sigpipe-tests-fixes)
Author: Martin Nordholts <martin.nordholts@codetale.se>
Date: Fri Feb 9 07:57:27 2024 +0100
tests: Add unix_sigpipe-different-duplicates.rs test variant
To make sure that
#[unix_sigpipe = "x"]
#[unix_sigpipe = "y"]
behaves like
#[unix_sigpipe = "x"]
#[unix_sigpipe = "x"]
commit d14f15862d
Author: Martin Nordholts <martin.nordholts@codetale.se>
Date: Fri Feb 9 08:47:47 2024 +0100
tests: Combine unix_sigpipe-not-used.rs and unix_sigpipe-only-feature.rs
The only difference between the files is the presence/absence of
#![feature(unix_sigpipe)]
attribute. Avoid duplication by using revisions instead.
commit a1cb3dba84
Author: Martin Nordholts <martin.nordholts@codetale.se>
Date: Fri Feb 9 06:44:56 2024 +0100
tests: Rename unix_sigpipe.rs to unix_sigpipe-bare.rs for clarity
The test is for the "bare" variant of the attribute that looks like this:
#[unix_sigpipe]
which is not allowed, because it must look like this:
#[unix_sigpipe = "sig_ign"]
commit e060274e55
Author: Martin Nordholts <martin.nordholts@codetale.se>
Date: Fri Feb 9 05:48:24 2024 +0100
tests: Fix typo unix_sigpipe-error.rs -> unix_sigpipe-sig_ign.rs
There is no error expected. It's simply the "regular" test for sig_ign.
So rename it.
```
</details>
Tracking issue: https://github.com/rust-lang/rust/issues/97889
- Put every literal in its own braces, rather than just some of them,
for maximal error recovery.
- Add a blank line between every case, for readability.
rename 'try' intrinsic to 'catch_unwind'
The intrinsic has nothing to do with `try` blocks, and corresponds to the stable `catch_unwind` function, so this makes a lot more sense IMO.
Also rename Miri's special function while we are at it, to reflect the level of abstraction it works on: it's an unwinding mechanism, on which Rust implements panics.
avoid generalization inside of aliases
The basic idea of this PR is that we don't generalize aliases when the instantiation could fail later on, either due to the *occurs check* or because of a universe error. We instead replace the whole alias with an inference variable and emit a nested `AliasRelate` goal. This `AliasRelate` then fully normalizes the alias before equating it with the inference variable, at which point the alias can be treated like any other rigid type.
We now treat aliases differently depending on whether they are *rigid* or not. To detect whether an alias is rigid we check whether `NormalizesTo` fails. While we already do so inside of `AliasRelate` anyways, also doing so when instantiating a query response would be both ugly/difficult and likely inefficient. To avoid that I change `instantiate_and_apply_query_response` to relate types completely structurally. This change generally removes a lot of annoying complexity, which is nice. It's implemented by adding a flag to `Equate` to change it to structurally handle aliases.
We currently always apply constraints from canonical queries right away. By providing all the necessary information to the canonical query, we can guarantee that instantiating the query response never fails, which further simplifies the implementation. This does add the invariant that *any information which could cause instantiating type variables to fail must also be available inside of the query*.
While it's acceptable for canonicalization to result in more ambiguity, we must not cause the solver to incompletely structurally relate aliases by erasing information. This means we have to be careful when merging universes during canonicalization. As we only generalize for type and const variables we have to make sure that anything nameable by such a type or const variable inside of the canonical query is also nameable outside of it. Because of this we both stop merging universes of existential variables when canonicalizing inputs, we put all uniquified regions into a higher universe which is not nameable by any type or const variable.
I will look into always replacing aliases with inference variables when generalizing in a later PR unless the alias references bound variables. This should both pretty much fix https://github.com/rust-lang/trait-system-refactor-initiative/issues/4. This may allow us to merge the universes of existential variables again by changing generalize to not consider their universe when deciding whether to generalize aliases. This requires some additional non-trivial changes to alias-relate, so I am leaving that as future work.
Fixes https://github.com/rust-lang/trait-system-refactor-initiative/issues/79. While it would be nice to decrement universe indices when existing a `forall`, that was surprisingly difficult and not necessary to fix this issue. I am really happy with the approach in this PR think it is the correct way forward to also fix the remaining cases of https://github.com/rust-lang/trait-system-refactor-initiative/issues/8.
Fix more #121208 fallout (round 3)
#121208 converted lots of delayed bugs to bugs. Unsurprisingly, there were a few invalid conversion found via fuzzing.
r? `@lcnr`
Don't unnecessarily change `SIGPIPE` disposition in `unix_sigpipe` tests
In `auxiliary/sigpipe-utils.rs`, all we want to know is the current `SIGPIPE` disposition. We should not change it. So use `libc::sigaction` instead of `libc::signal`. That way we can also remove the code that restores it.
Part of https://github.com/rust-lang/rust/issues/97889.