As is the current toy program:
fn main() -> std::io::Result<()> {
use std::fs;
let metadata = fs::metadata("foo.txt")?;
assert!(!metadata.is_dir());
Ok(())
}
... observed under strace will issue:
[snip]
statx(0, NULL, AT_STATX_SYNC_AS_STAT, STATX_ALL, NULL) = -1 EFAULT (Bad address)
statx(AT_FDCWD, "foo.txt", AT_STATX_SYNC_AS_STAT, STATX_ALL, {stx_mask=STATX_ALL|STATX_MNT_ID, stx_attributes=0, stx_mode=S_IFREG|0644, stx_size=0, ...}) = 0
While statx is not necessarily always present, checking for it can be
delayed to the first error condition. Said condition may very well never
happen, in which case the check got avoided altogether.
Note this is still suboptimal as there still will be programs issuing
it, but bulk of the problem is removed.
Tested by forbidding the syscall for the binary and observing it
correctly falls back to newfstatat.
While here tidy up the commentary, in particular by denoting some
problems with the current approach.
The test relied on Error::last_os_error() coming from the stat call on
the passed file, but there is no guarantee this will be the case.
Instead extract errno from the error returned by the routine.
Patch de facto written by joboet.
Co-authored-by: joboet <jonasboettiger@icloud.com>
Colorize `cargo check` diagnostics in VSCode via text decorations
Fixes#13648
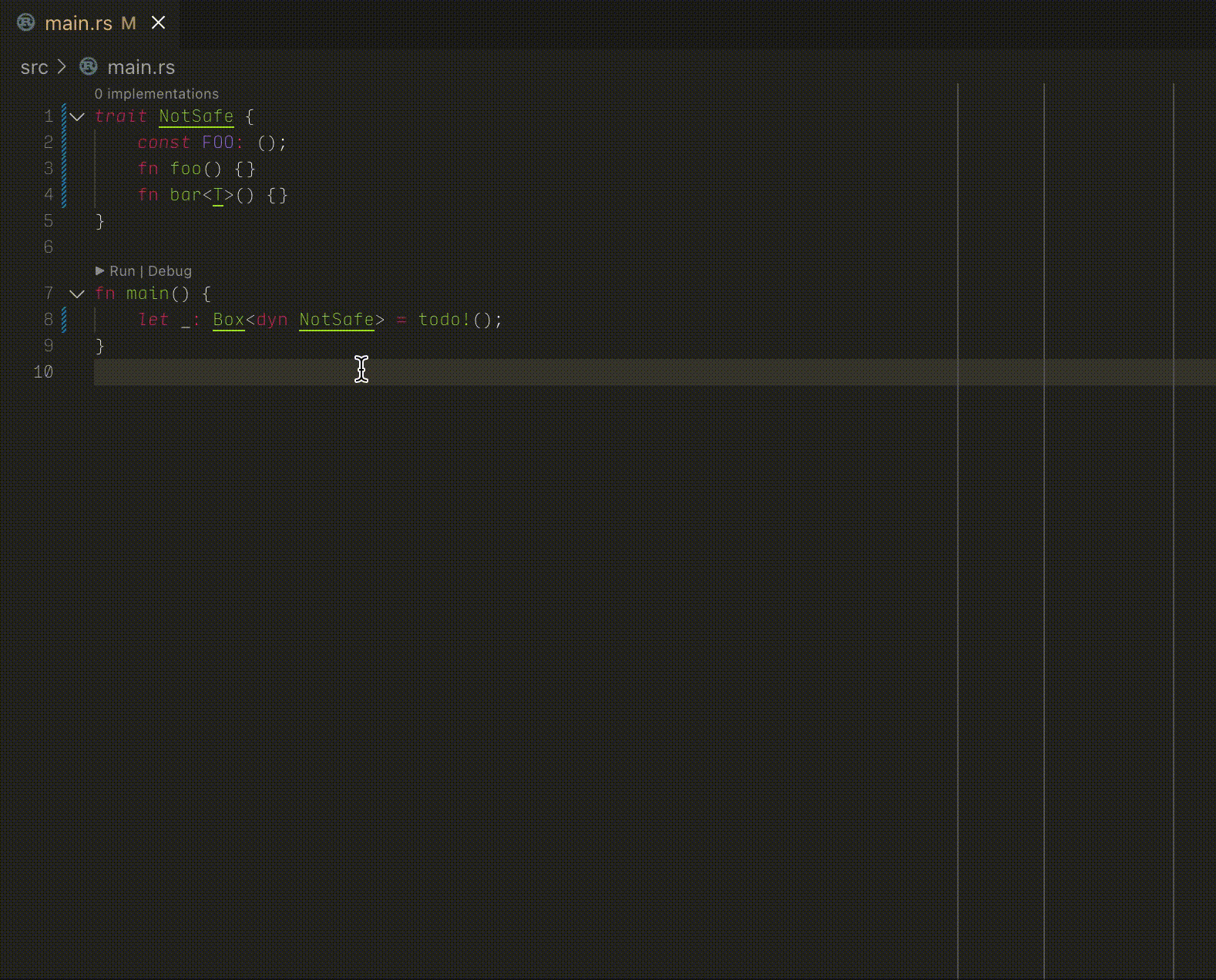
Use ANSI control characters to display text decorations matching the VScode terminal theme, and strip them out when providing text content for rustc diagnostics.
This adds the small [`anser`](https://www.npmjs.com/package/anser) library (MIT license, no dependencies) to parse the control codes, and it also supports HTML output so it should be fairly easy to switch to a rendered HTML/webview implementation in the future
I also updated the default `cargo check` command to use the rendered ANSI diagnostics, although I'm not sure if it makes sense to put this kind of thing behind a feature flag, or whether it might have any issues on Windows (as I believe ANSI codes are not used for colorization there)?
Use ANSI control characters to display text decorations matching the
VScode terminal theme, and strip them out when providing text content
for rustc diagnostics.
This adds the small `anser` library to parse the control codes, and it
also supports HTML output so it should be fairly easy to switch to a
rendered HTML/webview implementation if desired.
Rename `checkOnSave` settings to `check`
Now that flychecks can be triggered without saving the setting name doesn't make that much sense anymore. This PR renames it to just `check`, but keeps `checkOnSave` as the enabling setting.
error-code docs improvements (No. 2)
- Added empty error-code docs for `E0208`, `E0640` and `E0717` with the "internal" header as discussed on Discord.
- Wrote docs and UI test for `E0711`, again with the header.
- `tidy` changes are common-sense and make everything pass, `style.rs` hack is annoying though.
r? ```@GuillaumeGomez```
Use FxIndexSet when updating obligation causes in `adjust_fulfillment_errors_for_expr_obligation`
I have no idea how to test this reliably, but I've **manually** verified it fixes the instability in #106417 that isn't due to dtolnay/trybuild#212.
Fixes#106417
Simplify some canonical type alias names
* delete the `Canonicalized<'tcx>` type alias in favor for `Canonical<'tcx>`
* `CanonicalizedQueryResponse` -> `CanonicalQueryResponse`
I don't particularly care about the latter, but it should be consistent. We could alternatively delete the first alias and rename the struct to `Canonicalized`, and then keep the name of `CanonicalizedQueryResponse` untouched.
Move `check_region_obligations_and_report_errors` to `TypeErrCtxt`
Makes sense for this function to live with its sibling `resolve_regions_and_report_errors`, around which it's basically just a wrapper.
Enable Shadow Call Stack for Fuchsia on AArch64
Fuchsia already uses SCS by default for C/C++ code on ARM hardware. This patch allows SCS to be used for Rust code as well.
Fix ui constant tests for big-endian platforms
A number of tests under ui/const-ptr and ui/consts are currently failing on big-endian platforms as the binary encoding of some constants is hard-coded in the stderr test files.
Fix this by a combination of two types of changes:
- Where possible (i.e. where the particular value of a constant does not affect the purpose of the test), choose constant values that have the same encoding on big- and little-endian platforms.
- Where this is not possible, provide a normalize-stderr-test rule that transforms the printed big-endian encoding of such constants into the corresponding little-endian form.
Fixes part of https://github.com/rust-lang/rust/issues/105383.
Remove invalid case for mutable borrow suggestion
If we have a call such as `foo(&mut buf)` and after reference
collapsing the type is inferred as `&T` where-as the required type is
`&mut T`, don't suggest `foo(&mut mut buf)`. This is wrong syntactically
and the issue lies elsewhere, not in the borrow.
Fixes#105645
Change a commit_if_ok call to probe
Removes an over-eager `commit_if_ok` which makes inference worse.
I'm not entirely sure whether it's ok to remove the check that types are the same, because casting seems to cause equality checks with incorrect types?
Fixes#105037
r? ```@BoxyUwU```
Add action to expand a declarative macro once, inline. Fixes#13598
This commit adds a new r-a method, `expandMacroInline`, which expands the macro that's currently selected. See #13598 for the most applicable issue; though I suspect it'll resolve part of #5949 and make #11888 significantly easier).
The macro works like this:
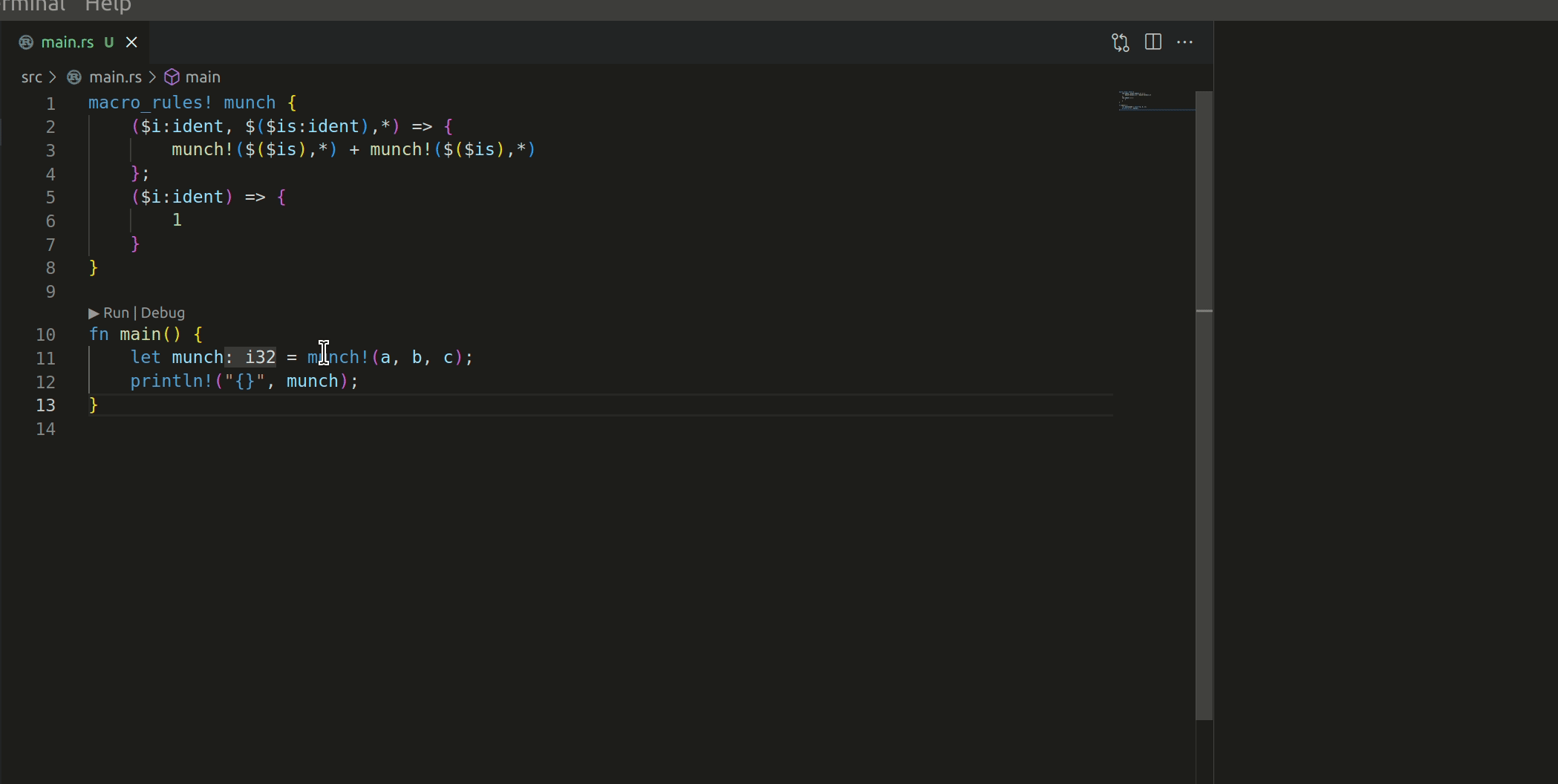
I have 2 questions before this PR can be merged:
1. **Should we rustfmt the output?** The advantage of doing this is neater code. The disadvantages are we'd have to format the whole expr/stmt/block (since there's no point just formatting one part, especially over multiple lines), and maybe it moves the code around more in weird ways. My suggestion here is to start off by not doing any formatting; and if it appears useful we can decide to do formatting in a later release.
2. **Is it worth solving the `$crate` hygiene issue now?** -- I think this PR is usable as of right now for some use-cases; but it is annoying that many common macros (i.e. `println!()`, `format!()`) can't be expanded further unless the user guesses the correct `$crate` value. The trouble with solving that issue is that I think it's complicated and imperfect. If we do solve it; we'd also need to either change the existing `expandMacro`/`expandMacroInline` commands; provide some option to allow/disallow `$crate` expanding; or come to some other compromise.
fix: generate async delegate methods
Fixes a bug where the generated async method doesn't await the result before returning it.
This is an example of what the output looked like:
```rust
struct Age<T>(T);
impl<T> Age<T> {
pub(crate) async fn age<J, 'a>(&'a mut self, ty: T, arg: J) -> T {
self.0
}
}
struct Person<T> {
age: Age<T>,
}
impl<T> Person<T> {
pub(crate) async fn age<J, 'a>(&'a mut self, ty: T, arg: J) -> T {
self.age.age(ty, arg) // .await is missing
}
}
```
The `.await` is missing, so the return type is `impl Future<Output = T>` instead of `T`
Always permit ConstProp to exploit arithmetic identities
Fixes https://github.com/rust-lang/rust/issues/72751
Initially, I thought I would need to enable operand propagation then do something else, but actually https://github.com/rust-lang/rust/pull/74491 already has the fix for the issue in question! It looks like this optimization was put under MIR opt level 3 due to possible soundness/stability implications, then demoted further to MIR opt level 4 when MIR opt level 2 became associated with `--release`.
Perhaps in the past we were doing CTFE on optimized MIR? We aren't anymore, so this optimization has no stability implications.
r? `@oli-obk`
feat: add the ability to limit the number of threads launched by `main_loop`
## Motivation
`main_loop` defaults to launch as many threads as cpus in one machine. When developing on multi-core remote servers on multiple projects, this will lead to thousands of idle threads being created. This is very annoying when one wants check whether his program under developing is running correctly via `htop`.
<img width="756" alt="image" src="https://user-images.githubusercontent.com/41831480/206656419-fa3f0dd2-e554-4f36-be1b-29d54739930c.png">
## Contribution
This patch introduce the configuration option `rust-analyzer.numThreads` to set the desired thread number used by the main thread pool.
This should have no effects on the performance as not all threads are actually used.
<img width="1325" alt="image" src="https://user-images.githubusercontent.com/41831480/206656834-fe625c4c-b993-4771-8a82-7427c297fd41.png">
## Demonstration
The following is a snippet of `lunarvim` configuration using my own build.
```lua
vim.list_extend(lvim.lsp.automatic_configuration.skipped_servers, { "rust_analyzer" })
require("lvim.lsp.manager").setup("rust_analyzer", {
cmd = { "env", "RA_LOG=debug", "RA_LOG_FILE=/tmp/ra-test.log",
"/home/jlhu/Projects/rust-analyzer/target/debug/rust-analyzer",
},
init_options = {
numThreads = 4,
},
settings = {
cachePriming = {
numThreads = 8,
},
},
})
```
## Limitations
The `numThreads` can only be modified via `initializationOptions` in early initialisation because everything has to wait until the thread pool starts including the dynamic settings modification support.
The `numThreads` also does not reflect the end results of how many threads is actually created, because I have not yet tracked down everything that spawns threads.
add wrapping/checked/saturating assist
This addresses #13452
I'm not sure about the structure of the code. I'm not sure if it needs to be 3 separate assists, and if that means it needs to be in 3 separate files as well.
Most of the logic is in `util.rs`, which feels funny to me, but there seems to be a pattern of 1 assist per file, and this seems better than duplicating the logic.
Let me know if anything needs changes 😁