Improve renaming suggestion when item starts with underscore
Fixes https://github.com/rust-lang/rust/issues/121776.
It goes from:
```terminal
error[E0433]: failed to resolve: use of undeclared type `Foo`
--> src/foo.rs:6:13
|
6 | let _ = Foo::Bar;
| ^^^ use of undeclared type `Foo`
|
help: an enum with a similar name exists, consider changing it
|
1 | enum Foo {
| ~~~
```
to:
```terminal
error[E0433]: failed to resolve: use of undeclared type `Foo`
--> foo.rs:6:13
|
6 | let _ = Foo::Bar;
| ^^^ use of undeclared type `Foo`
|
help: an enum with a similar name exists, consider renaming `_Foo` into `Foo`
|
1 | enum Foo {
| ~~~
error: aborting due to 1 previous error
```
bootstrap/format: send larger batches to rustfmt
This helps on systems with low core counts. To benchmark this I made a lot of files be modified:
```
for FILE in $(find compiler/ -name "*.rs"); do echo "// end of the file" >>$FILE; done
```
Then I ran
```
hyperfine "./x.py fmt -j1" -w 1 -r 4
```
Before this patch:
```
Benchmark 1: ./x.py fmt -j1
Time (mean ± σ): 3.426 s ± 0.032 s [User: 4.681 s, System: 1.376 s]
Range (min … max): 3.389 s … 3.462 s 4 runs
```
With this patch:
```
Benchmark 1: ./x.py fmt -j1
Time (mean ± σ): 2.530 s ± 0.054 s [User: 4.042 s, System: 0.467 s]
Range (min … max): 2.452 s … 2.576 s 4 runs
```
add platform-specific function to get the error number for HermitOS
Extending `std` to get the last error number for HermitOS.
HermitOS is a tier 3 platform and this PR changes only files, wich are related to the tier 3 platform.
Fix `async Fn` confirmation for `FnDef`/`FnPtr`/`Closure` types
Fixes three issues:
1. The code in `extract_tupled_inputs_and_output_from_async_callable` was accidentally getting the *future* type and the *output* type (returned by the future) messed up for fnptr/fndef/closure types. :/
2. We have a (class of) bug(s) in the old solver where we don't really support higher ranked built-in `Future` goals for generators. This is not possible to hit on stable code, but [can be hit with `unboxed_closures`](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2021&gist=e935de7181e37e13515ad01720bcb899) (#121653).
* I'm opting not to fix that in this PR. Instead, I just instantiate placeholders when confirming `async Fn` goals.
4. Fixed a bug when generating `FnPtr` shims for `async Fn` trait goals.
r? oli-obk
Fix typo in `rustc_passes/messages.ftl`
Line 190 contains unpaired parentheses:
```
passes_doc_cfg_hide_takes_list =
`#[doc(cfg_hide(...)]` takes a list of attributes
```
The `#[doc(cfg_hide(...)]` contains unpaired parentheses. This PR changes it to `#[doc(cfg_hide(...))]`, which made the parentheses paired.
Implement unwind safety for Condvar on all platforms
Closes#118009
This commit adds unwind safety consistency to Condvar. Previously, only select platforms implemented unwind safety through auto traits. Known by this committer: On Linux, `Condvar` implemented `UnwindSafe` but on Mac and Windows, it did not. This change changes the implementation from auto to explicit.
In #118009, it was suggested that the platform differences were a bug and that a simple PR could address this. In trying to determine the best information to put in the `#[stable]` attribute, it [was suggested](https://github.com/rust-lang/rust/issues/121690#issuecomment-1968298470) I copy the stability information from the previous unwind safety implementations.
Restore the standard library review rotation to its former glory
This adds 7 reviewers to the standard library review rotation, bringing the total back up to 10 people. Specifically:
* On the main rotation: ``@cuviper`` ``@Mark-Simulacrum`` ``@m-ou-se`` ``@Amanieu`` ``@Nilstrieb`` ``@workingjubilee`` ``@joboet`` ``@jhpratt``
* For `core` only: ``@scottmcm``
* For `std` only: ``@ChrisDenton``
For everyone pinged here, please confirm that you are happy to be added to the review rotation.
Deeply normalize obligations in `refining_impl_trait`
We somewhat awkwardly use semantic comparison when checking the `refining_impl_trait` lint. This relies on us being able to normalize bounds eagerly to avoid cases where an unnormalized alias is not considered equal to a normalized alias. Since `normalize` in the new solver is a noop, let's use `deeply_normalize` instead.
r? lcnr
cc ``@tmandry,`` this should fix your bug lol
Have `String` use `SliceIndex` impls from `str`
This PR simplifies the implementation of `Index` and `IndexMut` on `String`, and in the process enables indexing `String` by any user types that implement `SliceIndex<str>`.
Similar to #47832
r? libs
Not sure if this warrants a crater run.
Increase visibility of `join_path` and `split_paths`
Add some crosslinking among `std::env` pages to make it easier to discover `join_paths` and `split_paths`. Also add aliases to help anyone searching for `PATH`.
This commit:
- Moves the ICE file create/open outside the loop. (Redoing it on every
loop iteration works, but is really weird.)
- Moves the explanatory note emission above the loop, which removes the
need for the `enumerate` call.
- Introduces a `decorate` local.
Opportunistically resolve regions when processing region outlives obligations
Due to the matching in `TypeOutlives` being structural, we should attempt to opportunistically resolve regions before processing region obligations. Thanks ``@lcnr`` for finding this.
r? lcnr
Use `LitKind::Err` for malformed floats
#121120 changed `StringReader::cook_lexer_literal` to return `LitKind::Err` for malformed integer literals. This commit does the same for float literals, for consistency.
r? ``@fmease``
[rustdoc] Prevent inclusion of whitespace character after macro_rules ident
Discovered this bug randomly when looking at:
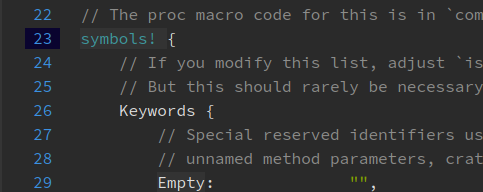
We were too eagerly trying to merge tokens that shouldn't be merged together (for example if you have a code comment followed by a code comment, we merge them in one attribute to reduce the DOM size).
r? ``@notriddle``
Closes#118009
This commit adds unwind safety to Condvar. Previously, only select
platforms implemented unwind safety through auto traits. Known by this
committer: Linux was unwind safe, but Mac and Windows are not before
this change.
Extending `std` to get the last error number for HermitOS.
HermitOS is a tier 3 platform and this PR changes only files,
wich are related to the tier 3 platform.
Diagnostic renaming
Renaming various diagnostic types from `Diagnostic*` to `Diag*`. Part of https://github.com/rust-lang/compiler-team/issues/722. There are more to do but this is enough for one PR.
r? `@davidtwco`
The C wrapper program represents a typical use case (linking
C libraries with Rust libraries) but it was not made explicit how
this was supposed to work in the usage example.
Also: correct a table alignment error for hexagon-unknown-none-elf on the
general platform support doc.
Rollup of 12 pull requests
Successful merges:
- #120051 (Add `display` method to `OsStr`)
- #121226 (Fix issues in suggesting importing extern crate paths)
- #121423 (Remove the `UntranslatableDiagnosticTrivial` lint.)
- #121527 (unix_sigpipe: Simple fixes and improvements in tests)
- #121572 (Add test case for primitive links in alias js)
- #121661 (Changing some attributes to only_local.)
- #121680 (Fix link generation for foreign macro in jump to definition feature)
- #121686 (Adjust printing for RPITITs)
- #121691 (handle unavailable creation time as `io::ErrorKind::Unsupported`)
- #121695 (Split rustc_type_ir to avoid rustc_ast from depending on it)
- #121698 (CFI: Fix typo in test file names)
- #121702 (Process alias-relate obligations in CoerceUnsized loop)
r? `@ghost`
`@rustbot` modify labels: rollup