mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-22 23:04:33 +00:00
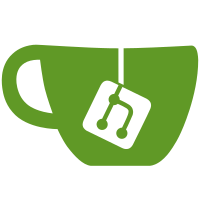
struct Concrete(u32); impl Concrete { fn m(self: &Box<Self>) -> &u32 { &self.0 } } resulted in a confusing error. impl Concrete { fn n(self: &Box<&Self>) -> &u32 { &self.0 } } resulted in no error or warning, despite apparent ambiguity over the elided lifetime. This commit changes two aspects of the behavior. Previously, when examining the self type, we considered lifetimes only if they were immediately adjacent to Self. We now consider lifetimes anywhere in the self type. Secondly, if more than one lifetime is discovered in the self type, we disregard it as a possible lifetime elision candidate. This is a compatibility break, and in fact has required some changes to tests which assumed the earlier behavior. Fixes https://github.com/rust-lang/rust/issues/117715
69 lines
1.5 KiB
Rust
69 lines
1.5 KiB
Rust
//@ run-rustfix
|
|
//@ edition:2018
|
|
|
|
#![feature(arbitrary_self_types)]
|
|
#![allow(non_snake_case, dead_code)]
|
|
|
|
use std::marker::PhantomData;
|
|
use std::ops::Deref;
|
|
use std::pin::Pin;
|
|
|
|
struct Struct {}
|
|
|
|
struct Wrap<T, P>(T, PhantomData<P>);
|
|
|
|
impl<T, P> Deref for Wrap<T, P> {
|
|
type Target = T;
|
|
fn deref(&self) -> &T {
|
|
&self.0
|
|
}
|
|
}
|
|
|
|
impl Struct {
|
|
// Test using `&self` sugar:
|
|
|
|
fn ref_self<'a>(&self, f: &'a u32) -> &'a u32 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
|
|
// Test using `&Self` explicitly:
|
|
|
|
fn ref_Self<'a>(self: &Self, f: &'a u32) -> &'a u32 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
|
|
fn box_ref_Self<'a>(self: Box<&Self>, f: &'a u32) -> &'a u32 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
|
|
fn pin_ref_Self<'a>(self: Pin<&Self>, f: &'a u32) -> &'a u32 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
|
|
fn box_box_ref_Self<'a>(self: Box<Box<&Self>>, f: &'a u32) -> &'a u32 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
|
|
fn box_pin_ref_Self<'a>(self: Box<Pin<&Self>>, f: &'a u32) -> &'a u32 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
|
|
fn wrap_ref_Self_Self<'a>(self: Wrap<&Self, Self>, f: &'a u8) -> &'a u8 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
|
|
fn ref_box_Self<'a>(self: &Box<Self>, f: &'a u32) -> &'a u32 {
|
|
f
|
|
//~^ ERROR lifetime may not live long enough
|
|
}
|
|
}
|
|
|
|
fn main() {}
|