mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 06:51:58 +00:00
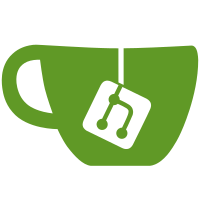
Decompose singular `matches!` with or-patterns to individual `matches!` statements to enable branchless code output. The following functions were changed: - `is_ascii_alphanumeric` - `is_ascii_hexdigit` - `is_ascii_punctuation` Add codegen tests Co-authored-by: George Bateman <george.bateman16@gmail.com> Co-authored-by: scottmcm <scottmcm@users.noreply.github.com>
48 lines
1.0 KiB
Rust
48 lines
1.0 KiB
Rust
// Checks that these functions are branchless.
|
|
//
|
|
// compile-flags: -O
|
|
|
|
#![crate_type = "lib"]
|
|
|
|
// CHECK-LABEL: @is_ascii_alphanumeric_char
|
|
#[no_mangle]
|
|
pub fn is_ascii_alphanumeric_char(x: char) -> bool {
|
|
// CHECK-NOT: br
|
|
x.is_ascii_alphanumeric()
|
|
}
|
|
|
|
// CHECK-LABEL: @is_ascii_alphanumeric_u8
|
|
#[no_mangle]
|
|
pub fn is_ascii_alphanumeric_u8(x: u8) -> bool {
|
|
// CHECK-NOT: br
|
|
x.is_ascii_alphanumeric()
|
|
}
|
|
|
|
// CHECK-LABEL: @is_ascii_hexdigit_char
|
|
#[no_mangle]
|
|
pub fn is_ascii_hexdigit_char(x: char) -> bool {
|
|
// CHECK-NOT: br
|
|
x.is_ascii_hexdigit()
|
|
}
|
|
|
|
// CHECK-LABEL: @is_ascii_hexdigit_u8
|
|
#[no_mangle]
|
|
pub fn is_ascii_hexdigit_u8(x: u8) -> bool {
|
|
// CHECK-NOT: br
|
|
x.is_ascii_hexdigit()
|
|
}
|
|
|
|
// CHECK-LABEL: @is_ascii_punctuation_char
|
|
#[no_mangle]
|
|
pub fn is_ascii_punctuation_char(x: char) -> bool {
|
|
// CHECK-NOT: br
|
|
x.is_ascii_punctuation()
|
|
}
|
|
|
|
// CHECK-LABEL: @is_ascii_punctuation_u8
|
|
#[no_mangle]
|
|
pub fn is_ascii_punctuation_u8(x: u8) -> bool {
|
|
// CHECK-NOT: br
|
|
x.is_ascii_punctuation()
|
|
}
|