mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 15:01:51 +00:00
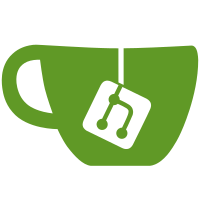
* The WASI targets deal with the `main` symbol a bit differently than native so some `codegen` and `assembly` tests have been ignored. * All `ignore-emscripten` directives have been updated to `ignore-wasm32` to be more clear that all wasm targets are ignored and it's not just Emscripten. * Most `ignore-wasm32-bare` directives are now gone. * Some ignore directives for wasm were switched to `needs-unwind` instead. * Many `ignore-wasm32*` directives are removed as the tests work with WASI as opposed to `wasm32-unknown-unknown`.
80 lines
1.8 KiB
Rust
80 lines
1.8 KiB
Rust
//@ run-pass
|
|
|
|
#![feature(intrinsics, rustc_attrs)]
|
|
|
|
mod rusti {
|
|
extern "rust-intrinsic" {
|
|
pub fn pref_align_of<T>() -> usize;
|
|
#[rustc_safe_intrinsic]
|
|
pub fn min_align_of<T>() -> usize;
|
|
}
|
|
}
|
|
|
|
#[cfg(any(target_os = "android",
|
|
target_os = "dragonfly",
|
|
target_os = "emscripten",
|
|
target_os = "freebsd",
|
|
target_os = "fuchsia",
|
|
target_os = "hurd",
|
|
target_os = "illumos",
|
|
target_os = "linux",
|
|
target_os = "macos",
|
|
target_os = "netbsd",
|
|
target_os = "openbsd",
|
|
target_os = "solaris",
|
|
target_os = "vxworks",
|
|
target_os = "nto",
|
|
))]
|
|
mod m {
|
|
#[cfg(target_arch = "x86")]
|
|
pub fn main() {
|
|
unsafe {
|
|
assert_eq!(::rusti::pref_align_of::<u64>(), 8);
|
|
assert_eq!(::rusti::min_align_of::<u64>(), 4);
|
|
}
|
|
}
|
|
|
|
#[cfg(not(target_arch = "x86"))]
|
|
pub fn main() {
|
|
unsafe {
|
|
assert_eq!(::rusti::pref_align_of::<u64>(), 8);
|
|
assert_eq!(::rusti::min_align_of::<u64>(), 8);
|
|
}
|
|
}
|
|
}
|
|
|
|
#[cfg(target_env = "sgx")]
|
|
mod m {
|
|
#[cfg(target_arch = "x86_64")]
|
|
pub fn main() {
|
|
unsafe {
|
|
assert_eq!(::rusti::pref_align_of::<u64>(), 8);
|
|
assert_eq!(::rusti::min_align_of::<u64>(), 8);
|
|
}
|
|
}
|
|
}
|
|
|
|
#[cfg(target_os = "windows")]
|
|
mod m {
|
|
pub fn main() {
|
|
unsafe {
|
|
assert_eq!(::rusti::pref_align_of::<u64>(), 8);
|
|
assert_eq!(::rusti::min_align_of::<u64>(), 8);
|
|
}
|
|
}
|
|
}
|
|
|
|
#[cfg(target_family = "wasm")]
|
|
mod m {
|
|
pub fn main() {
|
|
unsafe {
|
|
assert_eq!(::rusti::pref_align_of::<u64>(), 8);
|
|
assert_eq!(::rusti::min_align_of::<u64>(), 8);
|
|
}
|
|
}
|
|
}
|
|
|
|
fn main() {
|
|
m::main();
|
|
}
|