mirror of
https://github.com/rust-lang/rust.git
synced 2025-05-01 12:37:37 +00:00
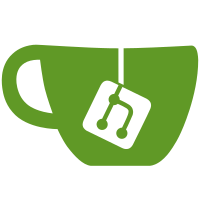
When writing a no_std binary, you'll be greeted with nonsensical errors mentioning lang items like eh_personality and start. That's pretty bad because it makes you think that you need to define them somewhere! But oh no, now you're getting the `internal_features` lint telling you that you shouldn't use them! But you need a no_std binary! What now? No problem! Writing a no_std binary is super easy. Just use panic=abort and supply your own platform specific entrypoint symbol (like `main`) and you're good to go. Would be nice if the compiler told you that, right? This makes it so that it does do that.
107 lines
2.8 KiB
Rust
107 lines
2.8 KiB
Rust
use std::path::PathBuf;
|
|
|
|
use crate::fluent_generated as fluent;
|
|
use rustc_errors::{DiagCtxt, DiagnosticBuilder, EmissionGuarantee, IntoDiagnostic, Level};
|
|
use rustc_macros::{Diagnostic, LintDiagnostic};
|
|
use rustc_span::{Span, Symbol};
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_recursion_limit)]
|
|
pub struct RecursionLimit {
|
|
#[primary_span]
|
|
pub span: Span,
|
|
pub shrunk: String,
|
|
#[note]
|
|
pub def_span: Span,
|
|
pub def_path_str: String,
|
|
#[note(monomorphize_written_to_path)]
|
|
pub was_written: Option<()>,
|
|
pub path: PathBuf,
|
|
}
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_type_length_limit)]
|
|
#[help(monomorphize_consider_type_length_limit)]
|
|
pub struct TypeLengthLimit {
|
|
#[primary_span]
|
|
pub span: Span,
|
|
pub shrunk: String,
|
|
#[note(monomorphize_written_to_path)]
|
|
pub was_written: Option<()>,
|
|
pub path: PathBuf,
|
|
pub type_length: usize,
|
|
}
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_no_optimized_mir)]
|
|
pub struct NoOptimizedMir {
|
|
#[note]
|
|
pub span: Span,
|
|
pub crate_name: Symbol,
|
|
}
|
|
|
|
pub struct UnusedGenericParamsHint {
|
|
pub span: Span,
|
|
pub param_spans: Vec<Span>,
|
|
pub param_names: Vec<String>,
|
|
}
|
|
|
|
impl<G: EmissionGuarantee> IntoDiagnostic<'_, G> for UnusedGenericParamsHint {
|
|
#[track_caller]
|
|
fn into_diagnostic(self, dcx: &'_ DiagCtxt, level: Level) -> DiagnosticBuilder<'_, G> {
|
|
let mut diag =
|
|
DiagnosticBuilder::new(dcx, level, fluent::monomorphize_unused_generic_params);
|
|
diag.span(self.span);
|
|
for (span, name) in self.param_spans.into_iter().zip(self.param_names) {
|
|
// FIXME: I can figure out how to do a label with a fluent string with a fixed message,
|
|
// or a label with a dynamic value in a hard-coded string, but I haven't figured out
|
|
// how to combine the two. 😢
|
|
diag.span_label(span, format!("generic parameter `{name}` is unused"));
|
|
}
|
|
diag
|
|
}
|
|
}
|
|
|
|
#[derive(LintDiagnostic)]
|
|
#[diag(monomorphize_large_assignments)]
|
|
#[note]
|
|
pub struct LargeAssignmentsLint {
|
|
#[label]
|
|
pub span: Span,
|
|
pub size: u64,
|
|
pub limit: u64,
|
|
}
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_symbol_already_defined)]
|
|
pub struct SymbolAlreadyDefined {
|
|
#[primary_span]
|
|
pub span: Option<Span>,
|
|
pub symbol: String,
|
|
}
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_couldnt_dump_mono_stats)]
|
|
pub struct CouldntDumpMonoStats {
|
|
pub error: String,
|
|
}
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_encountered_error_while_instantiating)]
|
|
pub struct EncounteredErrorWhileInstantiating {
|
|
#[primary_span]
|
|
pub span: Span,
|
|
pub formatted_item: String,
|
|
}
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_start_not_found)]
|
|
#[help]
|
|
pub struct StartNotFound;
|
|
|
|
#[derive(Diagnostic)]
|
|
#[diag(monomorphize_unknown_cgu_collection_mode)]
|
|
pub struct UnknownCguCollectionMode<'a> {
|
|
pub mode: &'a str,
|
|
}
|