mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 06:51:58 +00:00
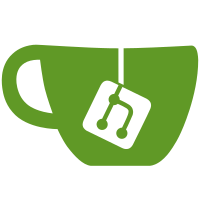
This patch updates the code that looks at the `Assume` type when evaluating if transmutation is possible. An ICE was being triggered in the case that the `Assume` parameter contained an unevaluated const (in this test case, due to a function with missing parameter names). Fixes #110892
41 lines
1.1 KiB
Rust
41 lines
1.1 KiB
Rust
// check-fail
|
|
#![feature(generic_const_exprs, transmutability)]
|
|
#![allow(incomplete_features)]
|
|
|
|
mod assert {
|
|
use std::mem::{Assume, BikeshedIntrinsicFrom};
|
|
|
|
pub fn is_transmutable<
|
|
Src,
|
|
Dst,
|
|
Context,
|
|
const ASSUME_ALIGNMENT: bool,
|
|
const ASSUME_LIFETIMES: bool,
|
|
const ASSUME_SAFETY: bool,
|
|
const ASSUME_VALIDITY: bool,
|
|
>()
|
|
where
|
|
Dst: BikeshedIntrinsicFrom<
|
|
Src,
|
|
Context,
|
|
{ from_options(ASSUME_ALIGNMENT, ASSUME_LIFETIMES, ASSUME_SAFETY, ASSUME_VALIDITY) }
|
|
>,
|
|
{}
|
|
|
|
// This should not cause an ICE
|
|
const fn from_options(
|
|
, //~ ERROR expected parameter name, found `,`
|
|
, //~ ERROR expected parameter name, found `,`
|
|
, //~ ERROR expected parameter name, found `,`
|
|
, //~ ERROR expected parameter name, found `,`
|
|
) -> Assume {} //~ ERROR mismatched types
|
|
}
|
|
|
|
fn main() {
|
|
struct Context;
|
|
#[repr(C)] struct Src;
|
|
#[repr(C)] struct Dst;
|
|
|
|
assert::is_transmutable::<Src, Dst, Context, false, false, { true }, false>();
|
|
}
|