mirror of
https://github.com/rust-lang/rust.git
synced 2024-10-31 22:41:50 +00:00
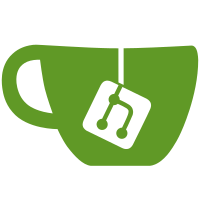
This adds the following functions: * `Option<T>::as_slice(&self) -> &[T]` * `Option<T>::as_slice_mut(&mut self) -> &[T]` The `as_slice` and `as_slice_mut` functions benefit from an optimization that makes them completely branch-free. Note that the optimization's soundness hinges on the fact that either the niche optimization makes the offset of the `Some(_)` contents zero or the mempory layout of `Option<T>` is equal to that of `Option<MaybeUninit<T>>`.
29 lines
561 B
Rust
29 lines
561 B
Rust
// compile-flags: -O
|
|
// only-x86_64
|
|
|
|
#![crate_type = "lib"]
|
|
#![feature(option_as_slice)]
|
|
|
|
extern crate core;
|
|
|
|
use core::num::NonZeroU64;
|
|
use core::option::Option;
|
|
|
|
// CHECK-LABEL: @u64_opt_as_slice
|
|
#[no_mangle]
|
|
pub fn u64_opt_as_slice(o: &Option<u64>) -> &[u64] {
|
|
// CHECK: start:
|
|
// CHECK-NOT: select
|
|
// CHECK: ret
|
|
o.as_slice()
|
|
}
|
|
|
|
// CHECK-LABEL: @nonzero_u64_opt_as_slice
|
|
#[no_mangle]
|
|
pub fn nonzero_u64_opt_as_slice(o: &Option<NonZeroU64>) -> &[NonZeroU64] {
|
|
// CHECK: start:
|
|
// CHECK-NOT: select
|
|
// CHECK: ret
|
|
o.as_slice()
|
|
}
|