mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 15:01:51 +00:00
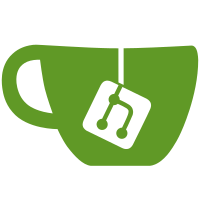
Currently, we assume that ScalarPair is always represented using a two-element struct, both as an immediate value and when stored in memory. This currently works fairly well, but runs into problems with https://github.com/rust-lang/rust/pull/116672, where a ScalarPair involving an i128 type can no longer be represented as a two-element struct in memory. For example, the tuple `(i32, i128)` needs to be represented in-memory as `{ i32, [3 x i32], i128 }` to satisfy alignment requirement. Using `{ i32, i128 }` instead will result in the second element being stored at the wrong offset (prior to LLVM 18). Resolve this issue by no longer requiring that the immediate and in-memory type for ScalarPair are the same. The in-memory type will now look the same as for normal struct types (and will include padding filler and similar), while the immediate type stays a simple two-element struct type. This also means that booleans in immediate ScalarPair are now represented as i1 rather than i8, just like we do everywhere else. The core change here is to llvm_type (which now treats ScalarPair as a normal struct) and immediate_llvm_type (which returns the two-element struct that llvm_type used to produce). The rest is fixing things up to no longer assume these are the same. In particular, this switches places that try to get pointers to the ScalarPair elements to use byte-geps instead of struct-geps.
291 lines
7.7 KiB
Rust
291 lines
7.7 KiB
Rust
// compile-flags: -O -C no-prepopulate-passes
|
|
|
|
#![crate_type = "lib"]
|
|
#![feature(dyn_star)]
|
|
|
|
use std::mem::MaybeUninit;
|
|
use std::num::NonZeroU64;
|
|
use std::marker::PhantomPinned;
|
|
use std::ptr::NonNull;
|
|
|
|
pub struct S {
|
|
_field: [i32; 8],
|
|
}
|
|
|
|
pub struct UnsafeInner {
|
|
_field: std::cell::UnsafeCell<i16>,
|
|
}
|
|
|
|
pub struct NotUnpin {
|
|
_field: i32,
|
|
_marker: PhantomPinned,
|
|
}
|
|
|
|
pub enum MyBool {
|
|
True,
|
|
False,
|
|
}
|
|
|
|
// CHECK: noundef zeroext i1 @boolean(i1 noundef zeroext %x)
|
|
#[no_mangle]
|
|
pub fn boolean(x: bool) -> bool {
|
|
x
|
|
}
|
|
|
|
// CHECK: i8 @maybeuninit_boolean(i8 %x)
|
|
#[no_mangle]
|
|
pub fn maybeuninit_boolean(x: MaybeUninit<bool>) -> MaybeUninit<bool> {
|
|
x
|
|
}
|
|
|
|
// CHECK: noundef zeroext i1 @enum_bool(i1 noundef zeroext %x)
|
|
#[no_mangle]
|
|
pub fn enum_bool(x: MyBool) -> MyBool {
|
|
x
|
|
}
|
|
|
|
// CHECK: i8 @maybeuninit_enum_bool(i8 %x)
|
|
#[no_mangle]
|
|
pub fn maybeuninit_enum_bool(x: MaybeUninit<MyBool>) -> MaybeUninit<MyBool> {
|
|
x
|
|
}
|
|
|
|
// CHECK: noundef i32 @char(i32 noundef %x)
|
|
#[no_mangle]
|
|
pub fn char(x: char) -> char {
|
|
x
|
|
}
|
|
|
|
// CHECK: i32 @maybeuninit_char(i32 %x)
|
|
#[no_mangle]
|
|
pub fn maybeuninit_char(x: MaybeUninit<char>) -> MaybeUninit<char> {
|
|
x
|
|
}
|
|
|
|
// CHECK: noundef i64 @int(i64 noundef %x)
|
|
#[no_mangle]
|
|
pub fn int(x: u64) -> u64 {
|
|
x
|
|
}
|
|
|
|
// CHECK: noundef i64 @nonzero_int(i64 noundef %x)
|
|
#[no_mangle]
|
|
pub fn nonzero_int(x: NonZeroU64) -> NonZeroU64 {
|
|
x
|
|
}
|
|
|
|
// CHECK: noundef i64 @option_nonzero_int(i64 noundef %x)
|
|
#[no_mangle]
|
|
pub fn option_nonzero_int(x: Option<NonZeroU64>) -> Option<NonZeroU64> {
|
|
x
|
|
}
|
|
|
|
// CHECK: @readonly_borrow(ptr noalias noundef readonly align 4 dereferenceable(4) %_1)
|
|
// FIXME #25759 This should also have `nocapture`
|
|
#[no_mangle]
|
|
pub fn readonly_borrow(_: &i32) {
|
|
}
|
|
|
|
// CHECK: noundef align 4 dereferenceable(4) ptr @readonly_borrow_ret()
|
|
#[no_mangle]
|
|
pub fn readonly_borrow_ret() -> &'static i32 {
|
|
loop {}
|
|
}
|
|
|
|
// CHECK: @static_borrow(ptr noalias noundef readonly align 4 dereferenceable(4) %_1)
|
|
// static borrow may be captured
|
|
#[no_mangle]
|
|
pub fn static_borrow(_: &'static i32) {
|
|
}
|
|
|
|
// CHECK: @named_borrow(ptr noalias noundef readonly align 4 dereferenceable(4) %_1)
|
|
// borrow with named lifetime may be captured
|
|
#[no_mangle]
|
|
pub fn named_borrow<'r>(_: &'r i32) {
|
|
}
|
|
|
|
// CHECK: @unsafe_borrow(ptr noundef nonnull align 2 %_1)
|
|
// unsafe interior means this isn't actually readonly and there may be aliases ...
|
|
#[no_mangle]
|
|
pub fn unsafe_borrow(_: &UnsafeInner) {
|
|
}
|
|
|
|
// CHECK: @mutable_unsafe_borrow(ptr noalias noundef align 2 dereferenceable(2) %_1)
|
|
// ... unless this is a mutable borrow, those never alias
|
|
#[no_mangle]
|
|
pub fn mutable_unsafe_borrow(_: &mut UnsafeInner) {
|
|
}
|
|
|
|
// CHECK: @mutable_borrow(ptr noalias noundef align 4 dereferenceable(4) %_1)
|
|
// FIXME #25759 This should also have `nocapture`
|
|
#[no_mangle]
|
|
pub fn mutable_borrow(_: &mut i32) {
|
|
}
|
|
|
|
// CHECK: noundef align 4 dereferenceable(4) ptr @mutable_borrow_ret()
|
|
#[no_mangle]
|
|
pub fn mutable_borrow_ret() -> &'static mut i32 {
|
|
loop {}
|
|
}
|
|
|
|
#[no_mangle]
|
|
// CHECK: @mutable_notunpin_borrow(ptr noundef nonnull align 4 %_1)
|
|
// This one is *not* `noalias` because it might be self-referential.
|
|
// It is also not `dereferenceable` due to
|
|
// <https://github.com/rust-lang/unsafe-code-guidelines/issues/381>.
|
|
pub fn mutable_notunpin_borrow(_: &mut NotUnpin) {
|
|
}
|
|
|
|
// CHECK: @notunpin_borrow(ptr noalias noundef readonly align 4 dereferenceable(4) %_1)
|
|
// But `&NotUnpin` behaves perfectly normal.
|
|
#[no_mangle]
|
|
pub fn notunpin_borrow(_: &NotUnpin) {
|
|
}
|
|
|
|
// CHECK: @indirect_struct(ptr noalias nocapture noundef readonly align 4 dereferenceable(32) %_1)
|
|
#[no_mangle]
|
|
pub fn indirect_struct(_: S) {
|
|
}
|
|
|
|
// CHECK: @borrowed_struct(ptr noalias noundef readonly align 4 dereferenceable(32) %_1)
|
|
// FIXME #25759 This should also have `nocapture`
|
|
#[no_mangle]
|
|
pub fn borrowed_struct(_: &S) {
|
|
}
|
|
|
|
// CHECK: @option_borrow(ptr noalias noundef readonly align 4 dereferenceable_or_null(4) %x)
|
|
#[no_mangle]
|
|
pub fn option_borrow(x: Option<&i32>) {
|
|
}
|
|
|
|
// CHECK: @option_borrow_mut(ptr noalias noundef align 4 dereferenceable_or_null(4) %x)
|
|
#[no_mangle]
|
|
pub fn option_borrow_mut(x: Option<&mut i32>) {
|
|
}
|
|
|
|
// CHECK: @raw_struct(ptr noundef %_1)
|
|
#[no_mangle]
|
|
pub fn raw_struct(_: *const S) {
|
|
}
|
|
|
|
// CHECK: @raw_option_nonnull_struct(ptr noundef %_1)
|
|
#[no_mangle]
|
|
pub fn raw_option_nonnull_struct(_: Option<NonNull<S>>) {
|
|
}
|
|
|
|
|
|
// `Box` can get deallocated during execution of the function, so it should
|
|
// not get `dereferenceable`.
|
|
// CHECK: noundef nonnull align 4 ptr @_box(ptr noalias noundef nonnull align 4 %x)
|
|
#[no_mangle]
|
|
pub fn _box(x: Box<i32>) -> Box<i32> {
|
|
x
|
|
}
|
|
|
|
// CHECK: noundef nonnull align 4 ptr @notunpin_box(ptr noundef nonnull align 4 %x)
|
|
#[no_mangle]
|
|
pub fn notunpin_box(x: Box<NotUnpin>) -> Box<NotUnpin> {
|
|
x
|
|
}
|
|
|
|
// CHECK: @struct_return(ptr noalias nocapture noundef sret(%S) align 4 dereferenceable(32){{( %_0)?}})
|
|
#[no_mangle]
|
|
pub fn struct_return() -> S {
|
|
S {
|
|
_field: [0, 0, 0, 0, 0, 0, 0, 0]
|
|
}
|
|
}
|
|
|
|
// Hack to get the correct size for the length part in slices
|
|
// CHECK: @helper([[USIZE:i[0-9]+]] noundef %_1)
|
|
#[no_mangle]
|
|
pub fn helper(_: usize) {
|
|
}
|
|
|
|
// CHECK: @slice(ptr noalias noundef nonnull readonly align 1 %_1.0, [[USIZE]] noundef %_1.1)
|
|
// FIXME #25759 This should also have `nocapture`
|
|
#[no_mangle]
|
|
pub fn slice(_: &[u8]) {
|
|
}
|
|
|
|
// CHECK: @mutable_slice(ptr noalias noundef nonnull align 1 %_1.0, [[USIZE]] noundef %_1.1)
|
|
// FIXME #25759 This should also have `nocapture`
|
|
#[no_mangle]
|
|
pub fn mutable_slice(_: &mut [u8]) {
|
|
}
|
|
|
|
// CHECK: @unsafe_slice(ptr noundef nonnull align 2 %_1.0, [[USIZE]] noundef %_1.1)
|
|
// unsafe interior means this isn't actually readonly and there may be aliases ...
|
|
#[no_mangle]
|
|
pub fn unsafe_slice(_: &[UnsafeInner]) {
|
|
}
|
|
|
|
// CHECK: @raw_slice(ptr noundef %_1.0, [[USIZE]] noundef %_1.1)
|
|
#[no_mangle]
|
|
pub fn raw_slice(_: *const [u8]) {
|
|
}
|
|
|
|
// CHECK: @str(ptr noalias noundef nonnull readonly align 1 %_1.0, [[USIZE]] noundef %_1.1)
|
|
// FIXME #25759 This should also have `nocapture`
|
|
#[no_mangle]
|
|
pub fn str(_: &[u8]) {
|
|
}
|
|
|
|
// CHECK: @trait_borrow(ptr noundef nonnull align 1 %_1.0, {{.+}} noalias noundef readonly align {{.*}} dereferenceable({{.*}}) %_1.1)
|
|
// FIXME #25759 This should also have `nocapture`
|
|
#[no_mangle]
|
|
pub fn trait_borrow(_: &dyn Drop) {
|
|
}
|
|
|
|
// CHECK: @option_trait_borrow(ptr noundef align 1 %x.0, ptr %x.1)
|
|
#[no_mangle]
|
|
pub fn option_trait_borrow(x: Option<&dyn Drop>) {
|
|
}
|
|
|
|
// CHECK: @option_trait_borrow_mut(ptr noundef align 1 %x.0, ptr %x.1)
|
|
#[no_mangle]
|
|
pub fn option_trait_borrow_mut(x: Option<&mut dyn Drop>) {
|
|
}
|
|
|
|
// CHECK: @trait_raw(ptr noundef %_1.0, {{.+}} noalias noundef readonly align {{.*}} dereferenceable({{.*}}) %_1.1)
|
|
#[no_mangle]
|
|
pub fn trait_raw(_: *const dyn Drop) {
|
|
}
|
|
|
|
// CHECK: @trait_box(ptr noalias noundef nonnull align 1{{( %0)?}}, {{.+}} noalias noundef readonly align {{.*}} dereferenceable({{.*}}){{( %1)?}})
|
|
#[no_mangle]
|
|
pub fn trait_box(_: Box<dyn Drop + Unpin>) {
|
|
}
|
|
|
|
// CHECK: { ptr, ptr } @trait_option(ptr noalias noundef align 1 %x.0, ptr %x.1)
|
|
#[no_mangle]
|
|
pub fn trait_option(x: Option<Box<dyn Drop + Unpin>>) -> Option<Box<dyn Drop + Unpin>> {
|
|
x
|
|
}
|
|
|
|
// CHECK: { ptr, [[USIZE]] } @return_slice(ptr noalias noundef nonnull readonly align 2 %x.0, [[USIZE]] noundef %x.1)
|
|
#[no_mangle]
|
|
pub fn return_slice(x: &[u16]) -> &[u16] {
|
|
x
|
|
}
|
|
|
|
// CHECK: { i16, i16 } @enum_id_1(i16 noundef %x.0, i16 %x.1)
|
|
#[no_mangle]
|
|
pub fn enum_id_1(x: Option<Result<u16, u16>>) -> Option<Result<u16, u16>> {
|
|
x
|
|
}
|
|
|
|
// CHECK: { i1, i8 } @enum_id_2(i1 noundef zeroext %x.0, i8 %x.1)
|
|
#[no_mangle]
|
|
pub fn enum_id_2(x: Option<u8>) -> Option<u8> {
|
|
x
|
|
}
|
|
|
|
// CHECK: { ptr, {{.+}} } @dyn_star(ptr noundef %x.0, {{.+}} noalias noundef readonly align {{.*}} dereferenceable({{.*}}) %x.1)
|
|
// Expect an ABI something like `{ {}*, [3 x i64]* }`, but that's hard to match on generically,
|
|
// so do like the `trait_box` test and just match on `{{.+}}` for the vtable.
|
|
#[no_mangle]
|
|
pub fn dyn_star(x: dyn* Drop) -> dyn* Drop {
|
|
x
|
|
}
|