mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 06:51:58 +00:00
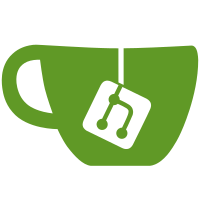
Like #107044, this will let us track compatibility with LLVM 16 going forward, especially after we eventually upgrade our own to the next. This also drops `tidy` here and in `x86_64-gnu-llvm-15`, syncing with that change in #106085.
39 lines
915 B
Rust
39 lines
915 B
Rust
// compile-flags: -O -Z randomize-layout=no
|
|
// only-x86_64
|
|
// ignore-llvm-version: 16.0.0
|
|
// ^ needs https://reviews.llvm.org/D146149 in 16.0.1
|
|
|
|
#![crate_type = "lib"]
|
|
#![feature(option_as_slice)]
|
|
|
|
extern crate core;
|
|
|
|
use core::num::NonZeroU64;
|
|
use core::option::Option;
|
|
|
|
// CHECK-LABEL: @u64_opt_as_slice
|
|
#[no_mangle]
|
|
pub fn u64_opt_as_slice(o: &Option<u64>) -> &[u64] {
|
|
// CHECK-NOT: select
|
|
// CHECK-NOT: br
|
|
// CHECK-NOT: switch
|
|
// CHECK-NOT: icmp
|
|
o.as_slice()
|
|
}
|
|
|
|
// CHECK-LABEL: @nonzero_u64_opt_as_slice
|
|
#[no_mangle]
|
|
pub fn nonzero_u64_opt_as_slice(o: &Option<NonZeroU64>) -> &[NonZeroU64] {
|
|
// CHECK-NOT: select
|
|
// CHECK-NOT: br
|
|
// CHECK-NOT: switch
|
|
// CHECK-NOT: icmp
|
|
// CHECK: %[[NZ:.+]] = icmp ne i64 %{{.+}}, 0
|
|
// CHECK-NEXT: zext i1 %[[NZ]] to i64
|
|
// CHECK-NOT: select
|
|
// CHECK-NOT: br
|
|
// CHECK-NOT: switch
|
|
// CHECK-NOT: icmp
|
|
o.as_slice()
|
|
}
|