mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 15:01:51 +00:00
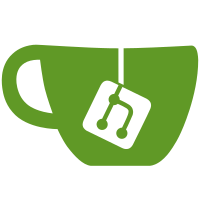
When going through auto-deref, the `<T as Clone>` impl sometimes needs to be specified for rustc to actually clone the value and not the reference. ``` error[E0507]: cannot move out of dereference of `S` --> $DIR/needs-clone-through-deref.rs:15:18 | LL | for _ in self.clone().into_iter() {} | ^^^^^^^^^^^^ ----------- value moved due to this method call | | | move occurs because value has type `Vec<usize>`, which does not implement the `Copy` trait | note: `into_iter` takes ownership of the receiver `self`, which moves value --> $SRC_DIR/core/src/iter/traits/collect.rs:LL:COL help: you can `clone` the value and consume it, but this might not be your desired behavior | LL | for _ in <Vec<usize> as Clone>::clone(&self.clone()).into_iter() {} | ++++++++++++++++++++++++++++++ + ``` CC #109429.
19 lines
403 B
Rust
19 lines
403 B
Rust
// run-rustfix
|
|
#![allow(dead_code, noop_method_call)]
|
|
use std::ops::Deref;
|
|
struct S(Vec<usize>);
|
|
impl Deref for S {
|
|
type Target = Vec<usize>;
|
|
fn deref(&self) -> &Self::Target {
|
|
&self.0
|
|
}
|
|
}
|
|
|
|
impl S {
|
|
fn foo(&self) {
|
|
// `self.clone()` returns `&S`, not `Vec`
|
|
for _ in self.clone().into_iter() {} //~ ERROR cannot move out of dereference of `S`
|
|
}
|
|
}
|
|
fn main() {}
|