mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 06:51:58 +00:00
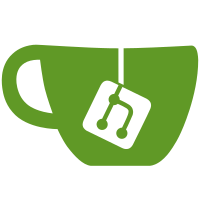
Allocate an extra space for unsized locals and manually align the storage, since alloca doesn't support dynamic alignment.
31 lines
565 B
Rust
31 lines
565 B
Rust
// Test that unsized locals uphold alignment requirements.
|
|
// Regression test for #71416.
|
|
// run-pass
|
|
#![feature(unsized_locals)]
|
|
#![allow(incomplete_features)]
|
|
use std::any::Any;
|
|
|
|
#[repr(align(256))]
|
|
#[allow(dead_code)]
|
|
struct A {
|
|
v: u8
|
|
}
|
|
|
|
impl A {
|
|
fn f(&self) -> *const A {
|
|
assert_eq!(self as *const A as usize % 256, 0);
|
|
self
|
|
}
|
|
}
|
|
|
|
fn mk() -> Box<dyn Any> {
|
|
Box::new(A { v: 4 })
|
|
}
|
|
|
|
fn main() {
|
|
let x = *mk();
|
|
let dwncst = x.downcast_ref::<A>().unwrap();
|
|
let addr = dwncst.f();
|
|
assert_eq!(addr as usize % 256, 0);
|
|
}
|