mirror of
https://github.com/rust-lang/rust.git
synced 2024-11-01 06:51:58 +00:00
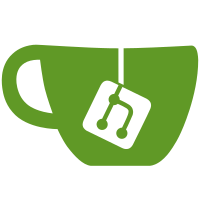
The `mir!` macro has multiple parts: - An optional return type annotation. - A sequence of zero or more local declarations. - A mandatory starting anonymous basic block, which is brace-delimited. - A sequence of zero of more additional named basic blocks. Some `mir!` invocations use braces with a "block" style, like so: ``` mir! { let _unit: (); { let non_copy = S(42); let ptr = std::ptr::addr_of_mut!(non_copy); // Inside `callee`, the first argument and `*ptr` are basically // aliasing places! Call(_unit = callee(Move(*ptr), ptr), ReturnTo(after_call), UnwindContinue()) } after_call = { Return() } } ``` Some invocations use parens with a "block" style, like so: ``` mir!( let x: [i32; 2]; let one: i32; { x = [42, 43]; one = 1; x = [one, 2]; RET = Move(x); Return() } ) ``` And some invocations uses parens with a "tighter" style, like so: ``` mir!({ SetDiscriminant(*b, 0); Return() }) ``` This last style is generally used for cases where just the mandatory starting basic block is present. Its braces are placed next to the parens. This commit changes all `mir!` invocations to use braces with a "block" style. Why? - Consistency is good. - The contents of the invocation is a block of code, so it's odd to use parens. They are more normally used for function-like macros. - Most importantly, the next commit will enable rustfmt for `tests/mir-opt/`. rustfmt is more aggressive about formatting macros that use parens than macros that use braces. Without this commit's changes, rustfmt would break a couple of `mir!` macro invocations that use braces within `tests/mir-opt` by inserting an extraneous comma. E.g.: ``` mir!(type RET = (i32, bool);, { // extraneous comma after ';' RET.0 = 1; RET.1 = true; Return() }) ``` Switching those `mir!` invocations to use braces avoids that problem, resulting in this, which is nicer to read as well as being valid syntax: ``` mir! { type RET = (i32, bool); { RET.0 = 1; RET.1 = true; Return() } } ```
23 lines
602 B
Rust
23 lines
602 B
Rust
// skip-filecheck
|
|
// Test that MatchBranchSimplification doesn't ICE on a SwitchInt where
|
|
// one of the targets is the block that the SwitchInt terminates.
|
|
#![crate_type = "lib"]
|
|
#![feature(core_intrinsics, custom_mir)]
|
|
use std::intrinsics::mir::*;
|
|
|
|
// EMIT_MIR switch_to_self.test.MatchBranchSimplification.diff
|
|
#[custom_mir(dialect = "runtime", phase = "post-cleanup")]
|
|
pub fn test(x: bool) {
|
|
mir! {
|
|
{
|
|
Goto(bb0)
|
|
}
|
|
bb0 = {
|
|
match x { false => bb0, _ => bb1 }
|
|
}
|
|
bb1 = {
|
|
match x { false => bb0, _ => bb1 }
|
|
}
|
|
}
|
|
}
|