mirror of
https://github.com/rust-lang/rust.git
synced 2025-04-27 18:56:24 +00:00
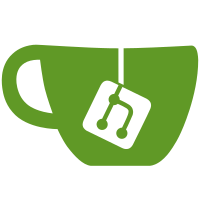
This PR updates the lint added in 9b40bd7
to ignore repr(C) structs that also
have repr(packed) or repr(align(n)).
As these representations can be modifiers on repr(C), it is assumed that users
that add these should know what they are doing, and thus the the lint should not
warn on the respective structs. For example, for the time being, using
repr(packed) and manually padding a repr(C) struct can be done to correctly
align struct members on AIX.
177 lines
4.1 KiB
Rust
177 lines
4.1 KiB
Rust
//@ check-pass
|
|
//@ compile-flags: --target powerpc64-ibm-aix
|
|
//@ needs-llvm-components: powerpc
|
|
//@ add-core-stubs
|
|
#![feature(no_core)]
|
|
#![no_core]
|
|
#![no_std]
|
|
|
|
extern crate minicore;
|
|
use minicore::*;
|
|
|
|
#[warn(uses_power_alignment)]
|
|
|
|
#[repr(C)]
|
|
pub struct Floats {
|
|
a: f64,
|
|
b: u8,
|
|
c: f64, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
d: f32,
|
|
}
|
|
|
|
pub struct Floats2 {
|
|
a: f64,
|
|
b: u32,
|
|
c: f64,
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct Floats3 {
|
|
a: f32,
|
|
b: f32,
|
|
c: i64,
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct Floats4 {
|
|
a: u64,
|
|
b: u32,
|
|
c: f32,
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct Floats5 {
|
|
a: f32,
|
|
b: f64, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
c: f32,
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct FloatAgg1 {
|
|
x: Floats,
|
|
y: f64, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct FloatAgg2 {
|
|
x: i64,
|
|
y: Floats, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct FloatAgg3 {
|
|
x: FloatAgg1,
|
|
// NOTE: the "power" alignment rule is infectious to nested struct fields.
|
|
y: FloatAgg2, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
z: FloatAgg2, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct FloatAgg4 {
|
|
x: FloatAgg1,
|
|
y: FloatAgg2, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct FloatAgg5 {
|
|
x: FloatAgg1,
|
|
y: FloatAgg2, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
z: FloatAgg3, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct FloatAgg6 {
|
|
x: i64,
|
|
y: Floats, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
z: u8,
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct FloatAgg7 {
|
|
x: i64,
|
|
y: Floats, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
z: u8,
|
|
zz: f32,
|
|
}
|
|
|
|
#[repr(C)]
|
|
pub struct A {
|
|
d: f64,
|
|
}
|
|
#[repr(C)]
|
|
pub struct B {
|
|
a: A,
|
|
f: f32,
|
|
d: f64, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
#[repr(C)]
|
|
pub struct C {
|
|
c: u8,
|
|
b: B, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
#[repr(C)]
|
|
pub struct D {
|
|
x: f64,
|
|
}
|
|
#[repr(C)]
|
|
pub struct E {
|
|
x: i32,
|
|
d: D, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
#[repr(C)]
|
|
pub struct F {
|
|
a: u8,
|
|
b: f64, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
#[repr(C)]
|
|
pub struct G {
|
|
a: u8,
|
|
b: u8,
|
|
c: f64, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
d: f32,
|
|
e: f64, //~ WARNING repr(C) does not follow the power alignment rule. This may affect platform C ABI compatibility for this type
|
|
}
|
|
// Should not warn on #[repr(packed)].
|
|
#[repr(packed)]
|
|
pub struct H {
|
|
a: u8,
|
|
b: u8,
|
|
c: f64,
|
|
d: f32,
|
|
e: f64,
|
|
}
|
|
#[repr(C, packed)]
|
|
pub struct I {
|
|
a: u8,
|
|
b: u8,
|
|
c: f64,
|
|
d: f32,
|
|
e: f64,
|
|
}
|
|
#[repr(C)]
|
|
pub struct J {
|
|
a: u8,
|
|
b: I,
|
|
}
|
|
// The lint also ignores diagnosing #[repr(align(n))].
|
|
#[repr(C, align(8))]
|
|
pub struct K {
|
|
a: u8,
|
|
b: u8,
|
|
c: f64,
|
|
d: f32,
|
|
e: f64,
|
|
}
|
|
#[repr(C)]
|
|
pub struct L {
|
|
a: u8,
|
|
b: K,
|
|
}
|
|
#[repr(C, align(8))]
|
|
pub struct M {
|
|
a: u8,
|
|
b: K,
|
|
c: L,
|
|
}
|
|
fn main() { }
|