Append .0 to unsuffixed float if it would otherwise become int token
Previously the unsuffixed f32/f64 constructors of `proc_macro::Literal` would create literal tokens that are definitely not a float:
```rust
Literal::f32_unsuffixed(10.0) // 10
Literal::f32_suffixed(10.0) // 10f32
Literal::f64_unsuffixed(10.0) // 10
Literal::f64_suffixed(10.0) // 10f64
```
Notice that the `10` are actually integer tokens if you were to reparse them, not float tokens.
This diff updates `Literal::f32_unsuffixed` and `Literal::f64_unsuffixed` to produce tokens that unambiguously parse as a float. This matches longstanding behavior of the proc-macro2 crate's implementation of these APIs dating back at least 3.5 years, so it's likely an unobjectionable behavior.
```rust
Literal::f32_unsuffixed(10.0) // 10.0
Literal::f32_suffixed(10.0) // 10f32
Literal::f64_unsuffixed(10.0) // 10.0
Literal::f64_suffixed(10.0) // 10f64
```
Fixes https://github.com/dtolnay/syn/issues/1085.
Implementation of GATs outlives lint
See #87479 for background. Closes#87479
The basic premise of this lint/error is to require the user to write where clauses on a GAT when those bounds can be implied or proven from any function on the trait returning that GAT.
## Intuitive Explanation (Attempt) ##
Let's take this trait definition as an example:
```rust
trait Iterable {
type Item<'x>;
fn iter<'a>(&'a self) -> Self::Item<'a>;
}
```
Let's focus on the `iter` function. The first thing to realize is that we know that `Self: 'a` because of `&'a self`. If an impl wants `Self::Item` to contain any data with references, then those references must be derived from `&'a self`. Thus, they must live only as long as `'a`. Furthermore, because of the `Self: 'a` implied bound, they must live only as long as `Self`. Since it's `'a` is used in place of `'x`, it is reasonable to assume that any value of `Self::Item<'x>`, and thus `'x`, will only be able to live as long as `Self`. Therefore, we require this bound on `Item` in the trait.
As another example:
```rust
trait Deserializer<T> {
type Out<'x>;
fn deserialize<'a>(&self, input: &'a T) -> Self::Out<'a>;
}
```
The intuition is similar here, except rather than a `Self: 'a` implied bound, we have a `T: 'a` implied bound. Thus, the data on `Self::Out<'a>` is derived from `&'a T`, and thus it is reasonable to expect that the lifetime `'x` will always be less than `T`.
## Implementation Algorithm ##
* Given a GAT `<P0 as Trait<P1..Pi>>::G<Pi...Pn>` declared as `trait T<A1..Ai> for A0 { type G<Ai...An>; }` used in return type of one associated function `F`
* Given env `E` (including implied bounds) for `F`
* For each lifetime parameter `'a` in `P0...Pn`:
* For each other type parameter `Pi != 'a` in `P0...Pn`: // FIXME: this include of lifetime parameters too
* If `E => (P: 'a)`:
* Require where clause `Ai: 'a`
## Follow-up questions ##
* What should we do when we don't pass params exactly?
For this example:
```rust
trait Des {
type Out<'x, D>;
fn des<'z, T>(&self, data: &'z Wrap<T>) -> Self::Out<'z, Wrap<T>>;
}
```
Should we be requiring a `D: 'x` clause? We pass `Wrap<T>` as `D` and `'z` as `'x`, and should be able to prove that `Wrap<T>: 'z`.
r? `@nikomatsakis`
Rollup of 5 pull requests
Successful merges:
- #89942 (Reorder `widening_impl`s to make the doc clearer)
- #90569 (Fix tests using `only-i686` to use the correct `only-x86` directive)
- #90597 (Warn for variables that are no longer captured)
- #90623 (Remove more checks for LLVM < 12)
- #90626 (Properly register text_direction_codepoint_in_comment lint.)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Properly register text_direction_codepoint_in_comment lint.
This makes it known to the compiler so it can be configured like with `#![allow(text_direction_codepoint_in_comment)]`.
Fixes#90614.
Fix ICE when rustdoc is scraping examples inside of a proc macro
This PR provides a clearer semantics for how --scrape-examples interacts with macros. If an expression's span AND it's enclosing item's span both are not `from_expansion`, then the example will be scraped. The added test case `rustdoc-scrape-examples-macros` shows a variety of situations.
* A macro-rules macro that takes a function call as input: good
* A macro-rules macro that generates a function call as output: bad
* A proc-macro that generates a function call as output: bad
* An attribute macro that generates a function call as output: bad
* An attribute macro that takes a function call as input: good, if the proc macro is designed to propagate the input spans
I ran this updated rustdoc on pyo3 and confirmed that it successfully scrapes examples from inside a proc macro, eg
<img width="1013" alt="Screen Shot 2021-11-04 at 1 11 28 PM" src="https://user-images.githubusercontent.com/663326/140412691-81a3bb6b-a448-4a1b-a293-f7a795553634.png">
(cc `@mejrs)`
Additionally, this PR fixes an ordering bug in the highlighting logic.
Fixes https://github.com/rust-lang/rust/issues/90567.
r? `@jyn514`
Clean up some `-Z unstable-options` in tests.
Several of these tests were for features that have been stabilized, or otherwise don't need `-Z unstable-options`.
Add beginner friendly lifetime elision hint to E0623
Address #90170
Suggest adding a new lifetime parameter when two elided lifetimes should match up but don't.
Example:
```
error[E0623]: lifetime mismatch
--> $DIR/issue-90170-elision-mismatch.rs:2:35
|
LL | fn foo(slice_a: &mut [u8], slice_b: &mut [u8]) {
| --------- --------- these two types are declared with different lifetimes...
LL | core::mem::swap(&mut slice_a, &mut slice_b);
| ^^^^^^^^^^^^ ...but data from `slice_b` flows into `slice_a` here
|
= note: each elided lifetime in input position becomes a distinct lifetime
help: explicitly declare a lifetime and assign it to both
|
LL | fn foo<'a>(slice_a: &'a mut [u8], slice_b: &'a mut [u8]) {
| ++++ ++ ++
```
for
```rust
fn foo(slice_a: &mut [u8], slice_b: &mut [u8]) {
core::mem::swap(&mut slice_a, &mut slice_b);
}
```
Suggest adding a new lifetime parameter when two elided lifetimes should match up but don't
Issue #90170
This also changes the tests introduced by the previous commits because of another rustc issue (#90258)
The exact set of permissions granted when forming a raw reference is
currently undecided https://github.com/rust-lang/rust/issues/56604.
To avoid presupposing any particular outcome, adjust the const
qualification to be compatible with decision where raw reference
constructed from `addr_of!` grants mutable access.
Split doc_cfg and doc_auto_cfg features
Part of #90497.
With this feature, `doc_cfg` won't pick up items automatically anymore.
cc `@Mark-Simulacrum`
r? `@jyn514`
Collect `panic/panic_bounds_check` during monomorphization
This would prevent link time errors if these functions are `#[inline]` (e.g. when `panic_immediate_abort` is used).
Fix#90405Fixrust-lang/cargo#10019
`@rustbot` label: T-compiler A-codegen
[master] Fix CVE-2021-42574
This PR implements new lints to mitigate the impact of [CVE-2021-42574], caused by the presence of bidirectional-override Unicode codepoints in the compiled source code. [See the advisory][advisory] for more information about the vulnerability.
The changes in this PR will be released in tomorrow's nightly release.
[CVE-2021-42574]: https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2021-42574
[advisory]: https://blog.rust-lang.org/2021/11/01/cve-2021-42574.html
Test that promotion follows references when looking for drop
Noticed that this wasn't covered by any of existing tests.
The const checking and const qualification, which currently shares the
implementation with promotion, will likely need a different behaviour
here (see issue #90193).
Fix rare ICE during typeck in rustdoc scrape_examples
While testing the `--scrape-examples` extension on the [wasmtime](https://github.com/bytecodealliance/wasmtime) repository, I found some additional edge cases. Specifically, when asking to typecheck a body containing a function call, I would sometimes get an ICE if:
* The body doesn't exist
* The function's HIR node didn't have a type
This adds checks for both of those conditions.
(Also this updates a test to check that the sources of a reverse-dependency are correctly generated and linked.)
r? `@jyn514`
rustdoc: remove flicker during page load
The search bar has a `:disabled` style that makes it grey, which creates a distracting flicker from grey to white when the page finishes loading. The search bar should stay the same color throughout page load.
A blank white search bar might create an incorrect impression for users with JS turned off. Since they can't use the search functionality, I've hidden the search bar in noscript.css.
Fixes#90246
r? `@GuillaumeGomez`
Demo: https://rustdoc.crud.net/jsha/flashy-searchbar/std/string/struct.String.html
Noticed that this wasn't covered by any of existing tests.
The const checking and const qualification, which currently shares the
implementation with promotion, will likely need a different behaviour
here (see issue #90193).
Add #[must_use] to mem/ptr functions
There's a lot of low-level / unsafe stuff here. Are there legit use cases for ignoring any of these return values?
* No regressions in `./x.py test --stage 1 library/std src/tools/clippy`.
* One regression in `./x.py test --stage 1 src/test/ui`. Fixed.
* I am unable to run `./x.py doc` on my machine so I'll need to wait for the CI to verify doctests pass. I eyeballed all the adjacent tests and they all look okay.
Parent issue: #89692
r? ```@joshtriplett```
Use h3 and h4 for the variant name and the "Fields" subheading.
Remove the "of T" part of the "Fields" subheading.
Remove border-bottom from "Fields" subheading.
Move docblock below "Fields" listing.
Skipping verbose diagnostic suggestions when calling .as_ref() on type not implementing AsRef
Addresses #89806
Skipping suggestions when calling `.as_ref()` for types that do not implement the `AsRef` trait.
r? `@estebank`
Use `is_global` in `candidate_should_be_dropped_in_favor_of`
This manifistated in #90195 with compiler being unable to keep
one candidate for a trait impl, if where is a global impl and more
than one trait bound in the where clause.
Before #87280 `candidate_should_be_dropped_in_favor_of` was using
`TypeFoldable::is_global()` that was enough to discard the two
`ParamCandidate`s. But #87280 changed it to use
`TypeFoldable::is_known_global()` instead, which is pessimistic, so
now the compiler drops the global impl instead (because
`is_known_global` is not sure) and then can't decide between the
two `ParamCandidate`s.
Switching it to use `is_global` again solves the issue.
Fixes#90195.
Improve and test cross-crate hygiene
- Decode the parent expansion for traits and enums in `rustc_resolve`, this was already being used for resolution in typeck
- Avoid suggesting importing names with def-site hygiene, since it's often not useful
- Add more tests
r? `@petrochenkov`
Show all Deref implementations recursively
Fixes#87783.
This is a re-implementation of #80653, so taking the original PR comment:
This changes `rustdoc` to recursively follow `Deref` targets so that methods from all levels are added to the rendered output. This implementation displays the methods from all levels in the expanded state with separate sections for each level.
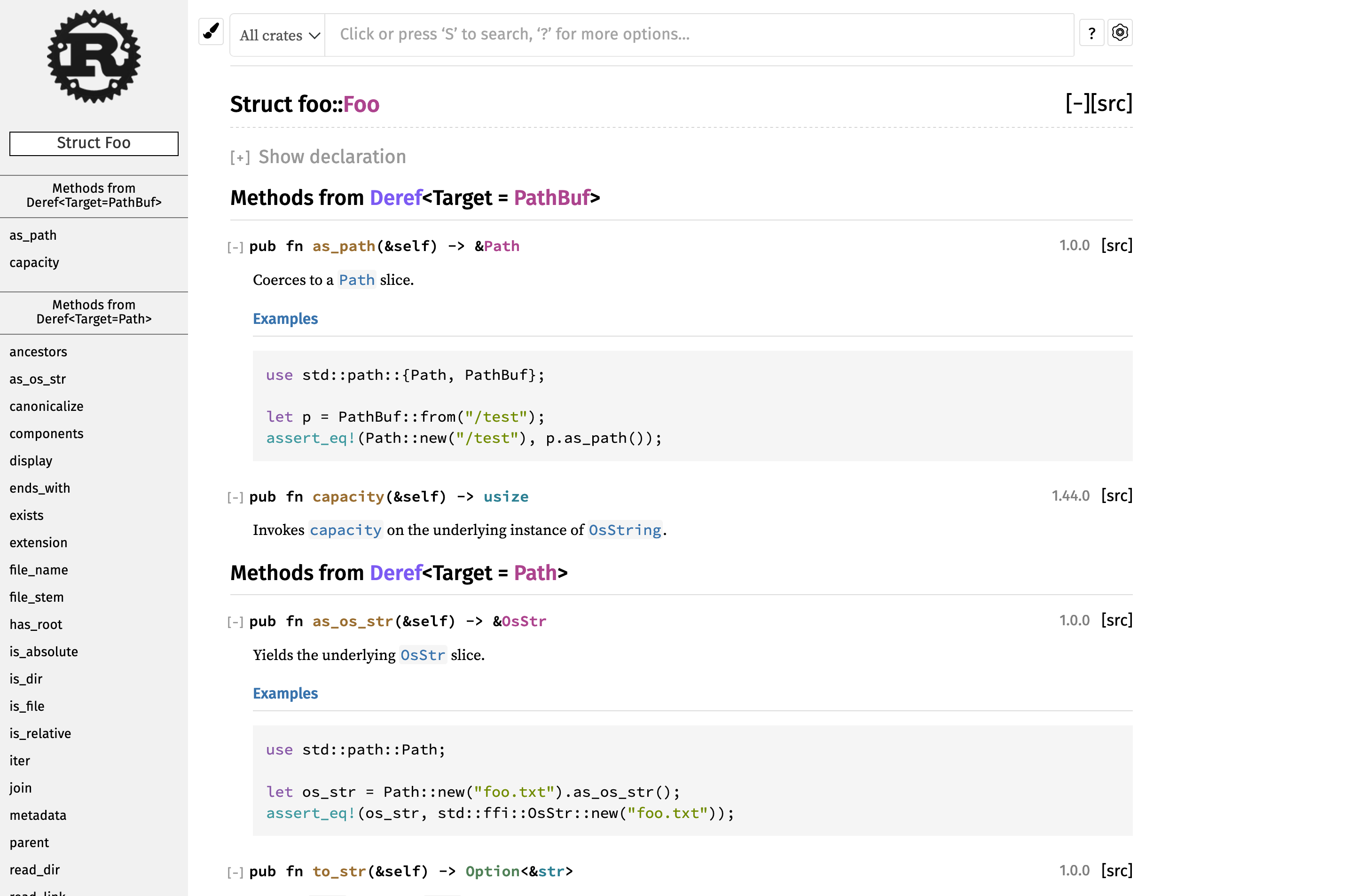
cc `@camelid`
r? `@jyn514`
Repace use of `static_nobundle` with `native_link_modifiers` within Rust codebase
This fixes warnings when building Rust and running tests:
```
warning: library kind `static-nobundle` has been superseded by specifying `-bundle` on library kind `static`. Try `static:-bundle`
warning: `rustc_llvm` (lib) generated 2 warnings (1 duplicate)
```
Unify titles in rustdoc book doc attributes chapter
As discussed in https://github.com/rust-lang/rust/pull/90339.
I wasn't able to find out where the link to the titles was used so let's see if the CI fails. :)
r? ``@camelid``
rustdoc: Fix generics generation in search index
The generics were not added to the search index as they should, instead they were added as arguments. I used this opportunity to allow generics to have generics themselves (will come in very handy for my current rewrite of the search engine!).
r? `@jyn514`
Use type based qualification for unions
Union field access is currently qualified based on the qualification of
a value previously assigned to the union. At the same time, every union
access transmutes the content of the union, which might result in a
different qualification.
For example, consider constants A and B as defined below, under the
current rules neither contains interior mutability, since a value used
in the initial assignment did not contain `UnsafeCell` constructor.
```rust
#![feature(untagged_unions)]
union U { i: u32, c: std::cell::Cell<u32> }
const A: U = U { i: 0 };
const B: std::cell::Cell<u32> = unsafe { U { i: 0 }.c };
```
To avoid the issue, the changes here propose to consider the content of
a union as opaque and use type based qualification for union types.
Fixes#90268.
`@rust-lang/wg-const-eval`
Consider indirect mutation during const qualification dataflow
Previously a local would be qualified if either one of two separate data
flow computations indicated so. First determined if a local could
contain the qualif, but ignored any forms of indirect mutation. Second
determined if a local could be mutably borrowed (and so indirectly
mutated), but which in turn ignored the qualif.
The end result was incorrect because the effect of indirect mutation was
effectivelly ignored in the all but the final stage of computation.
In the new implementation the indirect mutation is directly incorporated
into the qualif data flow. The local variable becomes immediately
qualified once it is mutably borrowed and borrowed place type can
contain the qualif.
In general we will now reject additional programs, program that were
prevously unintentionally accepted.
There are also some cases which are now accepted but were previously
rejected, because previous implementation didn't consider whether
borrowed place could have the qualif under the consideration.
Fixes#90124.
r? `@ecstatic-morse`
Revert "Add rustc lint, warning when iterating over hashmaps"
Fixes perf regressions introduced in https://github.com/rust-lang/rust/pull/90235 by temporarily reverting the relevant PR.
Fixes incorrect handling of ADT's drop requirements
Fixes#90024 and a bunch of duplicates.
The main issue was just that the contract of `NeedsDropTypes::adt_components` was inconsistent; the list of types it might return were the generic parameters themselves or the fields of the ADT, depending on the nature of the drop impl. This meant that the caller could not determine whether a `.subst()` call was still needed on those types; it called `.subst()` in all cases, and this led to ICEs when the returned types were the generic params.
First contribution of more than a few lines, so feedback definitely appreciated.
Union field access is currently qualified based on the qualification of
a value previously assigned to the union. At the same time, every union
access transmutes the content of the union, which might result in a
different qualification.
For example, consider constants A and B as defined below, under the
current rules neither contains interior mutability, since a value used
in the initial assignment did not contain `UnsafeCell` constructor.
```rust
#![feature(untagged_unions)]
union U { i: u32, c: std::cell::Cell<u32> }
const A: U = U { i: 0 };
const B: std::cell::Cell<u32> = unsafe { U { i: 0 }.c };
```
To avoid the issue, the changes here propose to consider the content of
a union as opaque and use type based qualification for union types.
Add hint for people missing `TryFrom`, `TryInto`, `FromIterator` import pre-2021
Adds a hint anytime a `TryFrom`, `TryInto`, `FromIterator` import is suggested noting that these traits are automatically imported in Edition 2021.
fix: inner attribute followed by outer attribute causing ICE
Fixes#87936, #88938, and #89971.
This removes the assertion that validates that there are no outer attributes following inner attributes. Where the inner attribute is invalid you get an actual error.
Add LLVM CFI support to the Rust compiler
This PR adds LLVM Control Flow Integrity (CFI) support to the Rust compiler. It initially provides forward-edge control flow protection for Rust-compiled code only by aggregating function pointers in groups identified by their number of arguments.
Forward-edge control flow protection for C or C++ and Rust -compiled code "mixed binaries" (i.e., for when C or C++ and Rust -compiled code share the same virtual address space) will be provided in later work as part of this project by defining and using compatible type identifiers (see Type metadata in the design document in the tracking issue #89653).
LLVM CFI can be enabled with -Zsanitizer=cfi and requires LTO (i.e., -Clto).
Thank you, `@eddyb` and `@pcc,` for all the help!
Rollup of 3 pull requests
Successful merges:
- #90154 (rustdoc: Remove `GetDefId`)
- #90232 (rustdoc: Use TTF based font instead of OTF for CJK glyphs to improve readability)
- #90278 (rustdoc: use better highlighting for *const, *mut, and &mut)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
rustdoc: Use TTF based font instead of OTF for CJK glyphs to improve readability
Due to Windows' implementation of font rendering, OpenType fonts can be distorted. So the existing font, Noto Sans KR, is not very readable on Windows. This PR improves readability of Korean glyphs on Windows.
## Before

## After

The fonts included in this PR are licensed under the SIL Open Font License and generated with these commands:
```sh
pyftsubset NanumBarunGothic.ttf \
--unicodes=U+AC00-D7AF,U+1100-11FF,U+3130-318F,U+A960-A97F,U+D7B0-D7FF \
--output-file=NanumBarunGothic.ttf.woff --flavor=woff
```
```sh
pyftsubset NanumBarunGothic.ttf \
--unicodes=U+AC00-D7AF,U+1100-11FF,U+3130-318F,U+A960-A97F,U+D7B0-D7FF \
--output-file=NanumBarunGothic.ttf.woff2 --flavor=woff2
```
r? ``@GuillaumeGomez``
Properly check `target_features` not to trigger an assertion
Fixes#89875
I think it should be a condition instead of an assertion to check if it's a register as it's possible that `reg` is a register class.
Also, this isn't related to the issue directly, but `is_target_supported` doesn't check `target_features` attributes. Is there any way to check it on rustc_codegen_llvm?
r? `@Amanieu`
Edit error messages for `rustc_resolve::AmbiguityKind` variants
Edit the language of the ambiguity descriptions for E0659. These strings now appear as notes.
Closes#79717.
Previously a local would be qualified if either one of two separate data
flow computations indicated so. First determined if a local could
contain the qualif, but ignored any forms of indirect mutation. Second
determined if a local could be mutably borrowed (and so indirectly
mutated), but which in turn ignored the qualif.
The end result was incorrect because the effect of indirect mutation was
effectivelly ignored in the all but the final stage of computation.
In the new implementation the indirect mutation is directly incorporated
into the qualif data flow. The local variable becomes immediately
qualified once it is mutably borrowed and borrowed place type can
contain the qualif.
In general we will now reject additional programs, program that were
prevously unintentionally accepted.
There are also some cases which are now accepted but were previously
rejected, because previous implementation didn't consider whether
borrowed place could have the qualif under the consideration.
Emit description of the ambiguity as a note.
Co-authored-by: Noah Lev <camelidcamel@gmail.com>
Co-authored-by: Vadim Petrochenkov <vadim.petrochenkov@gmail.com>
Avoid a branch on key being local for queries that use the same local and extern providers
Currently based on https://github.com/rust-lang/rust/pull/85810 as it slightly conflicts with it. Only the last two commits are new.
This commit adds LLVM Control Flow Integrity (CFI) support to the Rust
compiler. It initially provides forward-edge control flow protection for
Rust-compiled code only by aggregating function pointers in groups
identified by their number of arguments.
Forward-edge control flow protection for C or C++ and Rust -compiled
code "mixed binaries" (i.e., for when C or C++ and Rust -compiled code
share the same virtual address space) will be provided in later work as
part of this project by defining and using compatible type identifiers
(see Type metadata in the design document in the tracking issue #89653).
LLVM CFI can be enabled with -Zsanitizer=cfi and requires LTO (i.e.,
-Clto).
Prevent duplicate caller bounds candidates by exposing default substs in Unevaluated
Fixes https://github.com/rust-lang/rust/issues/89334
The changes introduced in https://github.com/rust-lang/rust/pull/87280 allowed for "duplicate" caller bounds candidates to be assembled that only differed in their default substs having been "exposed" or not and resulted in an ambiguity error during trait selection. To fix this we expose the defaults substs during the creation of the ParamEnv.
r? `@lcnr`
Do not mention a reexported item if it's private
Fixes#90113
The _actual_ regression was introduced in #73652, then #88838 made it worse. This fixes the issue by not counting such an import as a candidate.
Use the "nice E0277 errors"[1] for `!Send` `impl Future` from foreign crate
Partly address #78543 by making the error quieter.
We don't have access to the `typeck` tables from foreign crates, so we
used to completely skip the new code when checking foreign crates. Now,
we carry on and don't provide as nice output (we don't clarify *what* is
making the `Future: !Send`), but at least we no longer emit a sea of
derived obligations in the output.
[1]: https://blog.rust-lang.org/inside-rust/2019/10/11/AsyncAwait-Not-Send-Error-Improvements.html
r? `@tmandry`
Partly address #78543 by making the error quieter.
We don't have access to the `typeck` tables from foreign crates, so we
used to completely skip the new code when checking foreign crates. Now,
we carry on and don't provide as nice output (we don't clarify *what* is
making the `Future: !Send`), but at least we no longer emit a sea of
derived obligations in the output.
[1]: https://blog.rust-lang.org/inside-rust/2019/10/11/AsyncAwait-Not-Send-Error-Improvements.html
Fix ICE when forgetting to `Box` a parameter to a `Self::func` call
Closes#90213 .
Assuming we can get the `DefId` of the receiver causes an ICE if the receiver is `Self`. We can just avoid doing this though.
Update the minimum external LLVM to 12
With this change, we'll have stable support for LLVM 12 and 13.
For reference, the previous increase to LLVM 10 was #83387,
and this replaces the pending increase to LLVM 11 in #90062.
r? `@nagisa` `@nikic`
Rollup of 5 pull requests
Successful merges:
- #85833 (Scrape code examples from examples/ directory for Rustdoc)
- #88041 (Make all proc-macro back-compat lints deny-by-default)
- #89829 (Consider types appearing in const expressions to be invariant)
- #90168 (Reset qualifs when a storage of a local ends)
- #90198 (Add caveat about changing parallelism and function call overhead)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
This fixes warning when building Rust and running tests:
```
warning: library kind `static-nobundle` has been superseded by specifying `-bundle` on library kind `static`. Try `static:-bundle`
warning: `rustc_llvm` (lib) generated 2 warnings (1 duplicate)
```
Reset qualifs when a storage of a local ends
Reset qualifs when a storage of a local ends to ensure that the local qualifs
are affected by the state from previous loop iterations only if the local is
kept alive.
The change should be forward compatible with a stricter handling of indirect
assignments, since storage dead invalidates all existing pointers to the local.
Consider types appearing in const expressions to be invariant
This is an approach to fix#80977.
Currently, a type parameter which is only used in a constant expression is considered bivariant and will trigger error E0392 *"parameter T is never used"*.
Here is a short example:
```rust
pub trait Foo {
const N: usize;
}
struct Bar<T: Foo>([u8; T::N])
where [(); T::N]:;
```
([playgound](https://play.rust-lang.org/?version=nightly&mode=debug&edition=2015&gist=b51a272853f75925e72efc1597478aa5))
While it is possible to silence this error by adding a `PhantomData<T>` field, I think the better solution would be to make `T` invariant.
This would be analogous to the invariance constraints added for associated types.
However, I'm quite new to the compiler and unsure whether this is the right approach.
r? ``@varkor`` (since you authored #60058)
Make all proc-macro back-compat lints deny-by-default
The affected crates have had plenty of time to update.
By keeping these as lints rather than making them hard errors,
we ensure that downstream crates will still be able to compile,
even if they transitive depend on broken versions of the affected
crates.
This should hopefully discourage anyone from writing any
new code which relies on the backwards-compatibility behavior.
Implement coherence checks for negative trait impls
The main purpose of this PR is to be able to [move Error trait to core](https://github.com/rust-lang/project-error-handling/issues/3).
This feature is necessary to handle the following from impl on box.
```rust
impl From<&str> for Box<dyn Error> { ... }
```
Without having negative traits affect coherence moving the error trait into `core` and moving that `From` impl to `alloc` will cause the from impl to no longer compiler because of a potential future incompatibility. The compiler indicates that `&str` _could_ introduce an `Error` impl in the future, and thus prevents the `From` impl in `alloc` that would cause overlap with `From<E: Error> for Box<dyn Error>`. Adding `impl !Error for &str {}` with the negative trait coherence feature will disable this error by encoding a stability guarantee that `&str` will never implement `Error`, making the `From` impl compile.
We would have this in `alloc`:
```rust
impl From<&str> for Box<dyn Error> {} // A
impl<E> From<E> for Box<dyn Error> where E: Error {} // B
```
and this in `core`:
```rust
trait Error {}
impl !Error for &str {}
```
r? `@nikomatsakis`
This PR was built on top of `@yaahc` PR #85764.
Language team proposal: to https://github.com/rust-lang/lang-team/issues/96
to ensure that the local qualifs are affected by the state from previous
loop iterations only if the local is kept alive.
The change should be forward compatible with a stricter handling of
indirect assignments, since storage dead invalidates all existing
pointers to the local.
Make RSplit<T, P>: Clone not require T: Clone
This addresses a TODO comment. The behavior of `#[derive(Clone)]` *does* result in a `T: Clone` requirement. Playground example:
https://play.rust-lang.org/?version=stable&mode=debug&edition=2018&gist=a8b1a9581ff8893baf401d624a53d35b
Add a manual `Clone` implementation, mirroring `Split` and `SplitInclusive`.
`(R)?SplitN(Mut)?` don't have any `Clone` implementations, but I'll leave that for its own pull request.
Implement -Z location-detail flag
This PR implements the `-Z location-detail` flag as described in https://github.com/rust-lang/rfcs/pull/2091 .
`-Z location-detail=val` controls what location details are tracked when using `caller_location`. This allows users to control what location details are printed as part of panic messages, by allowing them to exclude any combination of filenames, line numbers, and column numbers. This option is intended to provide users with a way to mitigate the size impact of `#[track_caller]`.
Some measurements of the savings of this approach on an embedded binary can be found here: https://github.com/rust-lang/rust/issues/70579#issuecomment-942556822 .
Closes#70580 (unless people want to leave that open as a place for discussion of further improvements).
This is my first real PR to rust, so any help correcting mistakes / understanding side effects / improving my tests is appreciated :)
I have one question: RFC 2091 specified this as a debugging option (I think that is what -Z implies?). Does that mean this can never be stabilized without a separate MCP? If so, do I need to submit an MCP now, or is the initial RFC specifying this option sufficient for this to be merged as is, and then an MCP would be needed for eventual stabilization?
add feature flag for `type_changing_struct_update`
This implements the PR0 part of the mentoring notes within #86618.
overrides the previous inactive #86646 pr.
r? ```@nikomatsakis```
Report fatal lexer errors in `--cfg` command line arguments
Fixes#89358. The erroneous behavior was apparently introduced by `@Mark-Simulacrum` in a678e31911; the idea is to silence individual parser errors and instead emit one catch-all error message after parsing. However, for the example in #89358, a fatal lexer error is created here:
edebf77e00/compiler/rustc_parse/src/lexer/mod.rs (L340-L349)
This fatal error aborts the compilation, and so the call to `new_parser_from_source_str()` never returns and the catch-all error message is never emitted. I have therefore changed the `SilentEmitter` to silence only non-fatal errors; with my changes, for the rustc invocation described in #89358:
```sh
rustc --cfg "abc\""
```
I get the following output:
```
error[E0765]: unterminated double quote string
|
= note: this error occurred on the command line: `--cfg=abc"`
```
Add some tests for const_generics_defaults
I think this covers some of the stuff required for stabilisation report, some of these tests are probably covering stuff we already have but it can't hurt to have more :)
r? ````@lcnr````
Fix const qualification when executed after promotion
The const qualification was so far performed before the promotion and
the implementation assumed that it will never encounter a promoted.
With `const_precise_live_drops` feature, checking for live drops is
delayed until after drop elaboration, which in turn runs after
promotion. so the assumption is no longer true. When evaluating
`NeedsNonConstDrop` it is now possible to encounter promoteds.
Use type base qualification for the promoted. It is a sound
approximation in general, and in the specific case of promoteds and
`NeedsNonConstDrop` it is precise.
Fixes#89938.
Update E0637 description to mention `&` w/o an explicit lifetime name
Deal with https://github.com/rust-lang/rust/issues/89824#issuecomment-941598647. Another solution would be splitting the error code into two as (I think) it's a bit unclear to users why they have the same error code.
Don't mark for loop iter expression as desugared
We typically don't mark spans of lowered things as desugared. This helps Clippy rightly discern when code is (not) from expansion. This was discovered by ``@flip1995`` at https://github.com/rust-lang/rust-clippy/pull/7789#issuecomment-939289501.
This addresses a TODO comment. The behavior of #[derive(Clone)]
*does* result in a T: Clone requirement.
Add a manual Clone implementation, matching Split and SplitInclusive.
Add test for line-number setting
The first commit updates the version of the package to be able to have multi-line commands (which looks much nicer for this test).
r? ````@jsha````
Add test for debug logging during incremental compilation
Debug logging during incremental compilation had been broken for some
time, until #89343 fixed it (among other things). Add a test so this is
less likely to break without being noticed. This test is nearly a copy
of the `src/test/ui/rustc-rust-log.rs` test, but tests debug logging in
the incremental compliation code paths.
Erase late-bound regions before computing vtable debuginfo name.
Fixes#90019.
The `msvc_enum_fallback()` for computing enum type names needs to access the memory layout of niche enums in order to determine the type name. `compute_debuginfo_vtable_name()` did not properly erase regions before computing type names which made memory layout computation ICE when encountering un-erased regions.
r? `@wesleywiser`
Fix wrong niche calculation when 2+ niches are placed at the start
When the niche is at the start, existing code incorrectly uses 1 instead of count for subtraction.
Fix#90038
`@rustbot` label: T-compiler
Add test for issue #84957 - `str.as_bytes()` in a `const` expression
Hi, this PR adds a test for issue #84957 . I'm quite new to rustc so let me know if there's anything else that needs doing 😄Closes#84957
Do not promote values with const drop that need to be dropped
Changes from #88558 allowed using `~const Drop` in constants by
introducing a new `NeedsNonConstDrop` qualif.
The new qualif was also used for promotion purposes, and allowed
promotion to happen for values that needs to be dropped but which
do have a const drop impl.
Since for promoted the drop implementation is never executed,
this lead to observable change in behaviour. For example:
```rust
struct Panic();
impl const Drop for Panic {
fn drop(&mut self) {
panic!();
}
}
fn main() {
let _ = &Panic();
}
```
Restore the use of `NeedsDrop` qualif during promotion to avoid the issue.
Suggest a case insensitive match name regardless of levenshtein distance
Fixes#86170
Currently, `find_best_match_for_name` only returns a case insensitive match name depending on a Levenshtein distance. It's a bit unfortunate that that hides some suggestions for typos like `Bar` -> `BAR`. That idea is from https://github.com/rust-lang/rust/pull/46347#discussion_r153701834, but I think it still makes some sense to show a candidate when we find a case insensitive match name as it's more like a typo.
Skipped the `candidate != lookup` check because the current (i.e, `levenshtein_match`) returns the exact same `Symbol` anyway but it doesn't seem to confuse anything on UI tests.
r? ``@estebank``
Fix macro_rules! duplication when reexported in the same module
This can append if within the same module a `#[macro_export] macro_rules!`
is declared but also a reexport of itself producing two export of the same
macro in the same module. In that case we only want to document it once.
Before:
```
Module {
is_crate: true,
items: [
Id("0:4"), // pub use crate::repro as repro2;
Id("0:3"), // macro_rules! repro
Id("0:3"), // duplicate, same as above
],
}
```
After:
```
Module {
is_crate: true,
items: [
Id("0:4"), // pub use crate::repro as repro2;
Id("0:3"), // macro_rules! repro
],
}
```
Fixes https://github.com/rust-lang/rust/issues/89852
The const qualification was so far performed before the promotion and
the implementation assumed that it will never encounter a promoted.
With `const_precise_live_drops` feature, checking for live drops is
delayed until after drop elaboration, which in turn runs after
promotion. so the assumption is no longer true. When evaluating
`NeedsNonConstDrop` it is now possible to encounter promoteds.
Use type base qualification for the promoted. It is a sound
approximation in general, and in the specific case of promoteds and
`NeedsNonConstDrop` it is precise.
Debug logging during incremental compilation had been broken for some
time, until #89343 fixed it (among other things). Add a test so this is
less likely to break without being noticed. This test is nearly a copy
of the `src/test/ui/rustc-rust-log.rs` test, but tests debug logging in
the incremental compliation code paths.
Remove redundant member-constraint check
impl trait will, for each lifetime in the hidden type, register a "member constraint" that says the lifetime must be equal or outlive one of the lifetimes of the impl trait. These member constraints will be solved by borrowck
But, as you can see in the big red block of removed code, there was an ad-hoc check for member constraints happening at the site where they get registered. This check had some minor effects on diagnostics, but will fall down on its feet with my big type alias impl trait refactor. So we removed it and I pulled the removal out into a (hopefully) reviewable PR that works on master directly.
Changes from #88558 allowed using `~const Drop` in constants by
introducing a new `NeedsNonConstDrop` qualif.
The new qualif was also used for promotion purposes, and allowed
promotion to happen for values that needs to be dropped but which
do have a const drop impl.
Since for promoted the drop implementation is never executed,
this lead to observable change in behaviour. For example:
```rust
struct Panic();
impl const Drop for Panic {
fn drop(&mut self) {
panic!();
}
}
fn main() {
let _ = &Panic();
}
```
Restore the use of `NeedsDrop` qualif during promotion to avoid the issue.
Index and hash HIR as part of lowering
Part of https://github.com/rust-lang/rust/pull/88186
~Based on https://github.com/rust-lang/rust/pull/88880 (see merge commit).~
Once HIR is lowered, it is later indexed by the `index_hir` query and hashed for `crate_hash`. This PR moves those post-processing steps to lowering itself. As a side objective, the HIR crate data structure is refactored as an `IndexVec<LocalDefId, Option<OwnerInfo<'hir>>>` where `OwnerInfo` stores all the relevant information for an HIR owner.
r? `@michaelwoerister`
cc `@petrochenkov`
The affected crates have had plenty of time to update.
By keeping these as lints rather than making them hard errors,
we ensure that downstream crates will still be able to compile,
even if they transitive depend on broken versions of the affected
crates.
This should hopefully discourage anyone from writing any
new code which relies on the backwards-compatibility behavior.
Nicer error message if the user attempts to do let...else if
Gives a nice "conditional `else if` is not supported for `let...else`" error when encountering a `let...else if` pattern, as suggested in the [let...else tracking issue](https://github.com/rust-lang/rust/issues/87335#issuecomment-944846205).
Some "parenthesis" and "parentheses" fixes
"Parenthesis" is the singular (e.g. one `(` or one `)`) and "parentheses" is the plural (multiple `(` or `)`s) and this is not hard to mix up so here are some fixes for that.
Inspired by #89958
ty::pretty: prevent infinite recursion for `extern crate` paths.
Fixes#55779, fixes#87932.
This fix is based on `@estebank's` idea in https://github.com/rust-lang/rust/issues/55779#issuecomment-614758510 - but instead of trying to get `try_print_visible_def_path_recur`'s cycle detection to work in this case, this PR "just" disables the "visible path" feature when printing the path to an `extern crate`, so that the old recursion chain of `try_print_visible_def_path -> print_def_path -> try_print_visible_def_path`, is now impossible.
Both tests have been confirmed to crash `rustc` because of a stack overflow, without the fix.
polymorphization: shims and predicates
Supersedes #75737 and #75414. This pull request includes up some changes to polymorphization which hadn't landed previously and gets stage2 bootstrapping and the test suite passing when polymorphization is enabled. There are still issues with `type_id` and polymorphization to investigate but this should get polymorphization in a reasonable state to work on.
- #75737 and #75414 both worked but were blocked on having the rest of the test suite pass (with polymorphization enabled) with and without the PRs. It makes more sense to just land these so that the changes are in.
- #75737's changes remove the restriction of `InstanceDef::Item` on polymorphization, so that shims can now be polymorphized. This won't have much of an effect until polymorphization's analysis is more advanced, but it doesn't hurt.
- #75414's changes remove all logic which marks parameters as used based on their presence in predicates - given #75675, this will enable more polymorphization and avoid the symbol clashes that predicate logic previously sidestepped.
- Polymorphization now explicitly checks (and skips) foreign items, this is necessary for stage2 bootstrapping to work when polymorphization is enabled.
- The conditional determining the emission of a note adding context to a post-monomorphization error has been modified. Polymorphization results in `optimized_mir` running for shims during collection where that wouldn't happen previously, some errors are emitted during `optimized_mir` and these were considered post-monomorphization errors with the existing logic (more errors and shims have a `DefId` coming from the std crate, not the local crate), adding a note that resulted in tests failing. It isn't particularly feasible to change where polymorphization runs or prevent it from using `optimized_mir`, so it seemed more reasonable to not change the conditional.
- `characteristic_def_id_of_type` was being invoked during partitioning for self types of impl blocks which had projections that depended on the value of unused generic parameters of a function - this caused a ICE in a debuginfo test. If partitioning is enabled and the instance needs substitution then this is skipped. That test still fails for me locally, but not with an ICE, but it fails in a fresh checkout too, so 🤷♂️.
r? `@lcnr`
Remove FIXME since there is nothing to be fixed
Resolves#88593.
The errors are deduplicated when displayed to users. They only appear
multiple times in UI tests.
cc ``@jyn514``
r? ``@camelid``
Remove trailing semicolon from macro call span
Macro call site spans are now less surprising/more consistent since they no longer contain a semicolon after the macro call.
The downside is that we need to do a little guesswork to get the semicolon in diagnostics. But this should not be noticeable since it is rare for the semicolon to not immediately follow the macro call.
emitter: current substitution can be multi-line
Fixes#89280.
In `splice_lines`, there is some arithmetic to compute the required alignment such that future substitutions in a suggestion are aligned correctly. However, this assumed that the current substitution's span was only on a single line. In circumstances where this was not true, it could result in a arithmetic overflow when the substitution's end column was less than the substitution's start column.
r? ````@oli-obk````
Restrict the aarch64 outline atomics test to Linux
The test was introduced in #83655, which enables the `outline-atomics` feature for aarch64-unknown-linux-* but not for any other aarch64 targets. The test did not check for Linux causing test failures on aarch64-apple-darwin.
r? `@workingjubilee`
Stabilize `unreachable_unchecked` as `const fn`
Closes#53188
This PR stabilizes `core::hint::unreachable_unchecked` as `const fn`. MIRI is able to detect when this method is called. Stabilization was delayed until `const_panic` was stabilized so as to avoid users calling this method in its place (thus resulting in runtime UB). With #89508, that is no longer an issue.
````@rustbot```` label +A-const-eval +A-const-fn +T-lang +S-blocked
(not sure why it's T-lang, but that's what the tracking issue is)
Use BCryptGenRandom instead of RtlGenRandom on Windows.
This removes usage of RtlGenRandom on Windows, in favour of BCryptGenRandom.
BCryptGenRandom isn't available on XP, but we dropped XP support a while ago.
In `splice_lines`, there is some arithmetic to compute the required
alignment such that future substitutions in a suggestion are aligned
correctly. However, this assumed that the current substitution's span
was only on a single line. In circumstances where this was not true, it
could result in a arithmetic overflow when the substitution's end
column was less than the substitution's start column.
Signed-off-by: David Wood <david.wood@huawei.com>
Rollup of 7 pull requests
Successful merges:
- #86011 (move implicit `Sized` predicate to end of list)
- #89821 (Add a strange test for `unsafe_code` lint.)
- #89859 (add dedicated error variant for writing the discriminant of an uninhabited enum variant)
- #89870 (Suggest Box::pin when Pin::new is used instead)
- #89880 (Use non-checking TLS relocation in aarch64 asm! sym test.)
- #89885 (add long explanation for E0183)
- #89894 (Remove unused dependencies from rustc_const_eval)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Use non-checking TLS relocation in aarch64 asm! sym test.
The checking variant ensures that the offset required is not larger than 12 bits - hence we wouldn't ever need the upper 12 bits.
It's unlikely to ever fail in this small test but this is technically correct.
This was noticed incidentally when we found that LLD doesn't support the `tprel_lo12` relocation, even though LLVM can apparently generate it when using `-mtls-size=12`.
Suggest Box::pin when Pin::new is used instead
This fixes an incorrect diagnostic.
**Based on #89390**; only the last commit is specific to this PR. "Ignore whitespace changes" also helps here.
Add a strange test for `unsafe_code` lint.
The current behavior is a little surprising to me. I'm not sure whether people would change it, but at least let me document the current behavior with a test.
I learnt about this from the [totally-speedy-transmute](https://docs.rs/totally-speedy-transmute) crate.
cc #10599 the original implementation pr.
move implicit `Sized` predicate to end of list
In `Bounds::predicates()`, move the implicit `Sized` predicate to the
end of the generated list. This means that if there is an explicit
`Sized` bound, it will be checked first, and any resulting
diagnostics will have a more useful span.
Fixes#85998, at least partially. ~~Based on #85979, but only the last 2 commits are new for this pull request.~~ (edit: rebased) A full fix would need to deal with where-clauses, and that seems difficult. Basically, predicates are being collected in multiple stages, and there are two places where implicit `Sized` predicates can be inserted: once for generic parameters, and once for where-clauses. I think this insertion is happening too early, and we should actually do it only at points where we collect all of the relevant trait bounds for a type parameter.
I could use some help interpreting the changes to the stderr output. It looks like reordering the predicates changed some diagnostics that don't obviously have anything to do with `Sized` bounds. Possibly some error reporting code is making assumptions about ordering of predicates? The diagnostics for src/test/ui/derives/derives-span-Hash-*.rs seem to have improved, no longer pointing at the type parameter identifier, but src/test/ui/type-alias-impl-trait/generic_duplicate_param_use9.rs became less verbose for some reason.
I also ran into an instance of #84970 while working on this, but I kind of expected that could happen, because I'm reordering predicates. I can open a separate issue on that if it would be helpful.
``@estebank`` this seems likely to conflict (slightly?) with your work on #85947; how would you like to resolve that?
Fix incorrect Box::pin suggestion
The suggestion checked if `Pin<Box<T>>` could be coeerced to the expected
type, but did not check predicates created by the coercion. We now
look for predicates that definitely cannot be satisfied before giving
the suggestion.
The suggestion is still marked MaybeIncorrect because we allow predicates that
are still ambiguous and can't be proven.
Fixes#72117.
Add check that live_region is live in sanitize_promoted
This pull request fixes#88434 by adding a check in `sanitize_promoted` to ensure that only regions which are actually live are added to the `liveness_constraints` of the `BorrowCheckContext`.
To implement this change, I needed to add a method to `LivenessValues` which gets the elements contained by a region:
/// Returns an iterator of all the elements contained by the region `r`
crate fn get_elements(&self, row: N) -> impl Iterator<Item = Location> + '_
Then, inside `sanitize_promoted`, we check whether the iterator returned by this method is non-empty to ensure that the region is actually live at at least one location before adding that region to the `liveness_constraints` of the `BorrowCheckContext`.
This is my first pull request to the Rust repo, so any feedback on how I can improve this pull request or if there is a better way to fix this issue would be very appreciated.
Add `const_eval_select` intrinsic
Adds an intrinsic that calls a given function when evaluated at compiler time, but generates a call to another function when called at runtime.
See https://github.com/rust-lang/const-eval/issues/7 for previous discussion.
r? `@oli-obk.`
The suggestion checked if Pin<Box<T>> could be coeerced to the expected
type, but did not check predicates created by the coercion. We now
look for predicates that definitely cannot be satisfied before giving
the suggestion.
The suggestion is marked MaybeIncorrect because we allow predicates that
are still ambiguous and can't be proven.
suggestion for typoed crate or module
Previously, the compiler didn't suggest similarly named crates or modules. This pull request adds a suggestion for typoed crates or modules.
#76208
before:
```
error[E0433]: failed to resolve: use of undeclared type or module `chono`
--> src/main.rs:2:5
|
2 | use chono::prelude::*;
| ^^^^^ use of undeclared type or module `chono`
```
after:
```
error[E0433]: failed to resolve: use of undeclared type or module `chono`
--> src/main.rs:2:5
|
2 | use chono::prelude::*;
| ^^^^^
| |
| use of undeclared crate or module `chono`
| help: a similar crate or module exists: `chrono`
```
Remove textual span from diagnostic string
This is an unnecessary repetition, as the diagnostic prints the span anyway in the source path right below the message.
I further removed the identification of the node, as that does not give any new information in any of the cases that are changed in tests.
EDIT: also inserted a suggestion that other diagnostics were already emitting
add some more testcases
resolves#52893resolves#68295resolves#87750resolves#88071
All these issues have been fixed according to glacier. Just adding a test so it can be closed.
Can anybody tell me why the github keywords do not work? 🤔
Please edit this post if you can fix it.
Include rmeta candidates in "multiple matching crates" error
Only dylib and rlib candidates were included in the error. I think the
reason is that at the time this error was originally implemented, rmeta
crate sources were represented different from dylib and rlib sources.
I wrote up more detailed analysis in [this comment][1].
The new version of the code is also a bit easier to read and should be
more robust to future changes since it uses `CrateSources::paths()`.
I also changed the code to sort the candidates to make the output deterministic;
added full stderr tests for the error; and added a long error code explanation.
[1]: https://github.com/rust-lang/rust/pull/88675#issuecomment-935282436
cc `@Mark-Simulacrum` `@jyn514`
avoid suggesting the same name
sort candidates
fix a message
use `opt_def_id` instead of `def_id`
move `find_similarly_named_module_or_crate` to rustc_resolve/src/diagnostics.rs
Fix: non_exhaustive_omitted_patterns by filtering unstable and doc hidden variants
Fixes: #89042
Now that #86809 has been merged there are cases (std::io::ErrorKind) where unstable feature gated variants were included in warning/error messages when the feature was not turned on. This filters those variants out of the return of `SplitWildcard::new`.
Variants marked `doc(hidden)` are filtered out of the witnesses list in `Usefulness::apply_constructor`.
Probably worth a perf run 🤷 since this area can be sensitive.
The test is copied from `src/test/ui/crate-loading/crateresolve1.rs` and
its auxiliary tests. I added it to the `compile_fail` code example check
exemption list since it's hard if not impossible to reproduce this error
in a standalone code example.
Only dylib and rlib candidates were included in the error. I think the
reason is that at the time this error was originally implemented, rmeta
crate sources were represented different from dylib and rlib sources.
I wrote up more detailed analysis in [this comment][1].
The new version of the code is also a bit easier to read and should be
more robust to future changes since it uses `CrateSources::paths()`.
[1]: https://github.com/rust-lang/rust/pull/88675#issuecomment-935282436
Add test cases for unstable variants
Add test cases for doc hidden variants
Move is_doc_hidden to method on TyCtxt
Add unstable variants test to reachable-patterns ui test
Rename reachable-patterns -> omitted-patterns
Add #[must_use] to From::from and Into::into
Risk of churn: **High**
Magic 8-Ball says: **Outlook not so good**
I figured I'd put this out there. If we don't do it now maybe we save it for a rainy day.
Parent issue: #89692
r? `@joshtriplett`
Fix function-names test for GDB 10.1
This PR updates the test output in `src/test/debuginfo/function-names.rs` for GDB 10.1.
This should fix issue https://github.com/rust-lang/rust/issues/89750 -- but not the underlying problem of CI ignoring tests if not viable debugger happens to be present.
Ignore type of projections for upvar capturing
Fix#89606
Ignore type of projections for upvar capturing. Originally HashMap is used, and the hash/eq implementation of Place takes the type of projections into account. These types may differ by lifetime which causes #89606 to ICE.
I originally considered erasing regions but `place.ty()` is used when creating upvar tuple type, more than just serving as a key type, so I switched to a linear comparison with custom eq (`compare_place_ignore_ty`) instead.
r? `@wesleywiser`
`@rustbot` label +T-compiler
Add enum_intrinsics_non_enums lint
There is a clippy lint to prevent calling [`mem::discriminant`](https://doc.rust-lang.org/std/mem/fn.discriminant.html) with a non-enum type. I think the lint is worthy of being included in rustc, given that `discriminant::<T>()` where `T` is a non-enum has an unspecified return value, and there are no valid use cases where you'd actually want this.
I've also made the lint check [variant_count](https://doc.rust-lang.org/core/mem/fn.variant_count.html) (#73662).
closes#83899
Add #[must_use] to alloc constructors
Added `#[must_use]`. to the various forms of `new`, `pin`, and `with_capacity` in the `alloc` crate. No extra explanations given as I couldn't think of anything useful to add.
I figure this deserves extra scrutiny compared to the other PRs I've done so far. In particular:
* The 4 `pin`/`pin_in` methods I touched. Are there legitimate use cases for pinning and not using the result? Pinning's a difficult concept I'm not very comfortable with.
* `Box`'s constructors. Do people ever create boxes just for the side effects... allocating or zeroing out memory?
Parent issue: #89692
r? ``@joshtriplett``
Create more accurate debuginfo for vtables.
Before this PR all vtables would have the same name (`"vtable"`) in debuginfo. Now they get an unambiguous name that identifies the implementing type and the trait that is being implemented.
This is only one of several possible improvements:
- This PR describes vtables as arrays of `*const u8` pointers. It would nice to describe them as structs where function pointer is represented by a field with a name indicative of the method it maps to. However, this requires coming up with a naming scheme that avoids clashes between methods with the same name (which is possible if the vtable contains multiple traits).
- The PR does not update the debuginfo we generate for the vtable-pointer field in a fat `dyn` pointer. Right now there does not seem to be an easy way of getting ahold of a vtable-layout without also knowing the concrete self-type of a trait object.
r? `@wesleywiser`
Cleanup src/test/ui/{simd,simd-intrinsic}
Initial motivation was to simplify a huge macro expansion using a tuple, since we can just use an array in `#[repr(simd)]` now for the same result. But also, several tests were going unnoticed during development of SIMD intrinsics because people kept looking in the wrong directory, and many are basically run-pass vs. build-fail versions of the same tests, so let's keep them close together and simplify their names, so they're easier to sift through.