valtree is a version of constants that is inherently safe to be used within types.
This is in contrast to ty::Const which can have different representations of the same value. These representation differences can show up in hashing or equality comparisons, breaking type equality of otherwise equal types.
valtrees do not have this problem.
Shorten `rustc_middle::ty::mod`
Related to #60302.
This PR moves all `Adt*`, `Assoc*`, `Generic*`, and `UpVar*` types to separate files.
This, alongside some `use` reordering, puts `mod.rs` at ~2,200 lines, thus removing the `// ignore-tidy-filelength`.
The particular groups were chosen as they had 4 or more "substantive" members.
This pulls in rust-lang/rustc-rayon#8 to fix#81425. (h/t @ammaraskar)
That revealed weak constraints on `rustc_arena::DropArena`, because its
`DropType` was holding type-erased raw pointers to generic `T`. We can
implement `Send` for `DropType` (under `cfg(parallel_compiler)`) by
requiring all `T: Send` before they're type-erased.
Rollup of 9 pull requests
Successful merges:
- #81309 (always eagerly eval consts in Relate)
- #82217 (Edition-specific preludes)
- #82807 (rustdoc: Remove redundant enableSearchInput function)
- #82924 (WASI: Switch to crt1-command.o to enable support for new-style commands)
- #82949 (Do not attempt to unlock envlock in child process after a fork.)
- #82955 (fix: wrong word)
- #82962 (Treat header as first paragraph for shortened markdown descriptions)
- #82976 (fix error message for copy(_nonoverlapping) overflow)
- #82977 (Rename `Option::get_or_default` to `get_or_insert_default`)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Implement RFC 2945: "C-unwind" ABI
## Implement RFC 2945: "C-unwind" ABI
This branch implements [RFC 2945]. The tracking issue for this RFC is #74990.
The feature gate for the issue is `#![feature(c_unwind)]`.
This RFC was created as part of the ffi-unwind project group tracked at rust-lang/lang-team#19.
### Changes
Further details will be provided in commit messages, but a high-level overview
of the changes follows:
* A boolean `unwind` payload is added to the `C`, `System`, `Stdcall`,
and `Thiscall` variants, marking whether unwinding across FFI boundaries is
acceptable. The cases where each of these variants' `unwind` member is true
correspond with the `C-unwind`, `system-unwind`, `stdcall-unwind`, and
`thiscall-unwind` ABI strings introduced in RFC 2945 [3].
* This commit adds a `c_unwind` feature gate for the new ABI strings.
Tests for this feature gate are included in `src/test/ui/c-unwind/`, which
ensure that this feature gate works correctly for each of the new ABIs.
A new language features entry in the unstable book is added as well.
* We adjust the `rustc_middle::ty::layout::fn_can_unwind` function,
used to compute whether or not a `FnAbi` object represents a function that
should be able to unwind when `panic=unwind` is in use.
* Changes are also made to
`rustc_mir_build::build::should_abort_on_panic` so that the function ABI is
used to determind whether it should abort, assuming that the `panic=unwind`
strategy is being used, and no explicit unwind attribute was provided.
[RFC 2945]: https://github.com/rust-lang/rfcs/blob/master/text/2945-c-unwind-abi.md
Store HIR attributes in a side table
Same idea as #72015 but for attributes.
The objective is to reduce incr-comp invalidations due to modified attributes.
Notably, those due to modified doc comments.
Implementation:
- collect attributes during AST->HIR lowering, in `LocalDefId -> ItemLocalId -> &[Attributes]` nested tables;
- access the attributes through a `hir_owner_attrs` query;
- local refactorings to use this access;
- remove `attrs` from HIR data structures one-by-one.
Change in behaviour:
- the HIR visitor traverses all attributes at once instead of parent-by-parent;
- attribute arrays are sometimes duplicated: for statements and variant constructors;
- as a consequence, attributes are marked as used after unused-attribute lint emission to avoid duplicate lints.
~~Current bug: the lint level is not correctly applied in `std::backtrace_rs`, triggering an unused attribute warning on `#![no_std]`. I welcome suggestions.~~
Stabilize `unsafe_op_in_unsafe_fn` lint
This makes it possible to override the level of the `unsafe_op_in_unsafe_fn`, as proposed in https://github.com/rust-lang/rust/issues/71668#issuecomment-729770896.
Tracking issue: #71668
r? ```@nikomatsakis``` cc ```@SimonSapin``` ```@RalfJung```
# Stabilization report
This is a stabilization report for `#![feature(unsafe_block_in_unsafe_fn)]`.
## Summary
Currently, the body of unsafe functions is an unsafe block, i.e. you can perform unsafe operations inside.
The `unsafe_op_in_unsafe_fn` lint, stabilized here, can be used to change this behavior, so performing unsafe operations in unsafe functions requires an unsafe block.
For now, the lint is allow-by-default, which means that this PR does not change anything without overriding the lint level.
For more information, see [RFC 2585](https://github.com/rust-lang/rfcs/blob/master/text/2585-unsafe-block-in-unsafe-fn.md)
### Example
```rust
// An `unsafe fn` for demonstration purposes.
// Calling this is an unsafe operation.
unsafe fn unsf() {}
// #[allow(unsafe_op_in_unsafe_fn)] by default,
// the behavior of `unsafe fn` is unchanged
unsafe fn allowed() {
// Here, no `unsafe` block is needed to
// perform unsafe operations...
unsf();
// ...and any `unsafe` block is considered
// unused and is warned on by the compiler.
unsafe {
unsf();
}
}
#[warn(unsafe_op_in_unsafe_fn)]
unsafe fn warned() {
// Removing this `unsafe` block will
// cause the compiler to emit a warning.
// (Also, no "unused unsafe" warning will be emitted here.)
unsafe {
unsf();
}
}
#[deny(unsafe_op_in_unsafe_fn)]
unsafe fn denied() {
// Removing this `unsafe` block will
// cause a compilation error.
// (Also, no "unused unsafe" warning will be emitted here.)
unsafe {
unsf();
}
}
```
### Add debug assertion to check `AbiDatas` ordering
This makes a small alteration to `Abi::index`, so that we include a
debug assertion to check that the index we are returning corresponds
with the same abi in our data array.
This will help prevent ordering bugs in the future, which can
manifest in rather strange errors.
### Using exhaustive ABI matches
This slightly modifies the changes from our previous commits,
favoring exhaustive matches in place of `_ => ...` fall-through
arms.
This should help with maintenance in the future, when additional
ABI's are added, or when existing ABI's are modified.
### List all `-unwind` ABI's in unstable book
This updates the `c-unwind` page in the unstable book to list _all_
of the other ABI strings that are introduced by this feature gate.
Now, all of the ABI's specified by RFC 2945 are shown.
Co-authored-by: Amanieu d'Antras <amanieu@gmail.com>
Co-authored-by: Niko Matsakis <niko@alum.mit.edu>
### Changes
This commit implements unwind ABI's, specified in RFC 2945.
We adjust the `rustc_middle::ty::layout::fn_can_unwind` function,
used to compute whether or not a `FnAbi` object represents a
function that should be able to unwind when `panic=unwind` is in
use.
Changes are also made to
`rustc_mir_build::build::should_abort_on_panic` so that the
function ABI is used to determind whether it should abort, assuming
that the `panic=unwind` strategy is being used, and no explicit
unwind attribute was provided.
### Tests
Unit tests, checking that the behavior is correct for `C-unwind`,
`stdcall-unwind`, `system-unwind`, and `thiscall-unwind`, are
included. These alternative `unwind` ABI strings are specified in
RFC 2945, in the "_Other `unwind` ABI strings_" section.
Additionally, a test case is included to assert that the LLVM IR
generated for an external function defined with the `C-unwind` ABI
will be appropriately labeled with the `nounwind` LLVM attribute
when the `panic=abort` compilation flag is used.
### Ignore Directives
This commit uses `ignore-*` directives in two of our `*-unwind` ABI
test cases.
Specifically, the `stdcall-unwind` and `thiscall-unwind` test cases
ignore architectures that do not support `stdcall` and `thiscall`,
respectively.
These directives are cribbed from
`src/test/ui/c-variadic/variadic-ffi-1.rs` for `stdcall`, and
`src/test/ui/extern/extern-thiscall.rs` for `thiscall`.
### Overview
This commit begins the implementation work for RFC 2945. For more
information, see the rendered RFC [1] and tracking issue [2].
A boolean `unwind` payload is added to the `C`, `System`, `Stdcall`,
and `Thiscall` variants, marking whether unwinding across FFI
boundaries is acceptable. The cases where each of these variants'
`unwind` member is true correspond with the `C-unwind`,
`system-unwind`, `stdcall-unwind`, and `thiscall-unwind` ABI strings
introduced in RFC 2945 [3].
### Feature Gate and Unstable Book
This commit adds a `c_unwind` feature gate for the new ABI strings.
Tests for this feature gate are included in `src/test/ui/c-unwind/`,
which ensure that this feature gate works correctly for each of the
new ABIs.
A new language features entry in the unstable book is added as well.
### Further Work To Be Done
This commit does not proceed to implement the new unwinding ABIs,
and is intentionally scoped specifically to *defining* the ABIs and
their feature flag.
### One Note on Test Churn
This will lead to some test churn, in re-blessing hash tests, as the
deleted comment in `src/librustc_target/spec/abi.rs` mentioned,
because we can no longer guarantee the ordering of the `Abi`
variants.
While this is a downside, this decision was made bearing in mind
that RFC 2945 states the following, in the "Other `unwind` Strings"
section [3]:
> More unwind variants of existing ABI strings may be introduced,
> with the same semantics, without an additional RFC.
Adding a new variant for each of these cases, rather than specifying
a payload for a given ABI, would quickly become untenable, and make
working with the `Abi` enum prone to mistakes.
This approach encodes the unwinding information *into* a given ABI,
to account for the future possibility of other `-unwind` ABI
strings.
### Ignore Directives
`ignore-*` directives are used in two of our `*-unwind` ABI test
cases.
Specifically, the `stdcall-unwind` and `thiscall-unwind` test cases
ignore architectures that do not support `stdcall` and
`thiscall`, respectively.
These directives are cribbed from
`src/test/ui/c-variadic/variadic-ffi-1.rs` for `stdcall`, and
`src/test/ui/extern/extern-thiscall.rs` for `thiscall`.
This would otherwise fail on some targets, see:
fcf697f902
### Footnotes
[1]: https://github.com/rust-lang/rfcs/blob/master/text/2945-c-unwind-abi.md
[2]: https://github.com/rust-lang/rust/issues/74990
[3]: https://github.com/rust-lang/rfcs/blob/master/text/2945-c-unwind-abi.md#other-unwind-abi-strings
This updates all places where match branches check on StatementKind or UseContext.
This doesn't properly implement them, but adds TODOs where they are, and also adds some best
guesses to what they should be in some cases.
I'm still not totally sure if this is the right way to implement the memcpy, but that portion
compiles correctly now. Now to fix the compile errors everywhere else :).
Change x64 size checks to not apply to x32.
Rust contains various size checks conditional on target_arch = "x86_64", but these checks were never intended to apply to x86_64-unknown-linux-gnux32. Add target_pointer_width = "64" to the conditions.
Rust contains various size checks conditional on target_arch = "x86_64",
but these checks were never intended to apply to
x86_64-unknown-linux-gnux32. Add target_pointer_width = "64" to the
conditions.
Implement NOOP_METHOD_CALL lint
Implements the beginnings of https://github.com/rust-lang/lang-team/issues/67 - a lint for detecting noop method calls (e.g, calling `<&T as Clone>::clone()` when `T: !Clone`).
This PR does not fully realize the vision and has a few limitations that need to be addressed either before merging or in subsequent PRs:
* [ ] No UFCS support
* [ ] The warning message is pretty plain
* [ ] Doesn't work for `ToOwned`
The implementation uses [`Instance::resolve`](https://doc.rust-lang.org/nightly/nightly-rustc/rustc_middle/ty/instance/struct.Instance.html#method.resolve) which is normally later in the compiler. It seems that there are some invariants that this function relies on that we try our best to respect. For instance, it expects substitutions to have happened, which haven't yet performed, but we check first for `needs_subst` to ensure we're dealing with a monomorphic type.
Thank you to ```@davidtwco,``` ```@Aaron1011,``` and ```@wesleywiser``` for helping me at various points through out this PR ❤️.
Update measureme dependency to the latest version
This version adds the ability to use `rdpmc` hardware-based performance
counters instead of wall-clock time for measuring duration. This also
introduces a dependency on the `perf-event-open-sys` crate on Linux
which is used when using hardware counters.
r? ```@oli-obk```
Skip Ty w/o infer ty/const in trait select
Remove some allocations & also add `skip_current_subtree` to skip subtrees with no inferred items.
r? `@eddyb` since marked in the FIXME
This version adds the ability to use `rdpmc` hardware-based performance
counters instead of wall-clock time for measuring duration. This also
introduces a dependency on the `perf-event-open-sys` crate on Linux
which is used when using hardware counters.
Make the `Query` enum a simple struct.
A lot of code in `rustc_query_system` is generic over it, only to encode an exceptional error case: query cycles.
The delayed computations are now done at cycle detection.
MIR-OPT: Pass to deduplicate blocks
This pass finds basic blocks that are completely equal,
and replaces all uses with just one of them.
```bash
$ RUSTC_LOG=rustc_mir::transform::deduplicate_blocks ./x.py build --stage 2 | grep "SUCCESS: Replacing: " > log
...
$ cat log | wc -l
23875
```
Fix sizes of repr(C) enums on hexagon
Enums on hexagon use a smallest size (but at least 1 byte) that fits all
the enumeration values. This is unlike many other ABIs where enums are
at least 32 bits.
Fixes#82100
Enums on hexagon use a smallest size (but at least 1 byte) that fits all
the enumeration values. This is unlike many other ABIs where enums are
at least 32 bits.
Rollup of 7 pull requests
Successful merges:
- #80595 (`impl PartialEq<Punct> for char`; symmetry for #78636)
- #81991 (Fix panic in 'remove semicolon' when types are not local)
- #82176 (fix MIR fn-ptr pretty-printing)
- #82244 (Keep consistency in example for Stdin StdinLock)
- #82260 (rustc: Show ``@path`` usage in stable)
- #82316 (Fix minor mistake in LTO docs.)
- #82332 (Don't generate src link on dummy spans)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
fix MIR fn-ptr pretty-printing
An uninitialized function pointer would get printed as `{{uninit fn()}` (notice the unbalanced parentheses), and a dangling fn ptr would ICE. This fixes both of that.
However, I have no idea how to add tests for this.
Also, I don't understand this MIR pretty-printing code. Somehow the print function `pretty_print_const_scalar` actually *returns* a transformed form of the const (but there is no doc comment explaining what is being returned); some match arms do `p!` while others do `self =`, and there's a wild mixture of `p!` and `write!`... all very mysterious and confusing.^^
r? ``@oli-obk``
name async generators something more human friendly in type error diagnostic
fixes#81457
Some details:
1. I opted to load the generator kind from the hir in TyCategory. I also use 1 impl in the hir for the descr
2. I named both the source of the future, in addition to the general type (`future`), not sure what is preferred
3. I am not sure what is required to make sure "generator" is not referred to anywhere. A brief `rg "\"generator\"" showed me that most diagnostics correctly distinguish from generators and async generator, but the `descr` of `DefKind` is pretty general (not sure how thats used)
4. should the descr impl of AsyncGeneratorKind use its display impl instead of copying the string?
Print -Ztime-passes (and misc stats/logs) on stderr, not stdout.
I've tried not to change anything that looked similar to `rustc --print`, where people might use automation, and/or any "bulk" prints, such as dumping an entire Graphviz (`dot`) graph on stdout.
The reason I want `-Ztime-passes` to be on stderr like debug logging is I can get a complete (and correctly interleaved) view just by looking at stderr, which is merely a convenience when running `rustc`/Cargo directly, but even more important when it's nested in a build script, as Cargo will split the build script output into stdout (named `output`) and `stderr`.
Ensure valid TraitRefs are created for GATs
This fixes `ProjectionTy::trait_ref` to use the correct substs. Places that need all of the substs have been updated to not use `trait_ref`.
r? ````@jackh726````
Implement RFC 2580: Pointer metadata & VTable
RFC: https://github.com/rust-lang/rfcs/pull/2580
~~Before merging this PR:~~
* [x] Wait for the end of the RFC’s [FCP to merge](https://github.com/rust-lang/rfcs/pull/2580#issuecomment-759145278).
* [x] Open a tracking issue: https://github.com/rust-lang/rust/issues/81513
* [x] Update `#[unstable]` attributes in the PR with the tracking issue number
----
This PR extends the language with a new lang item for the `Pointee` trait which is special-cased in trait resolution to implement it for all types. Even in generic contexts, parameters can be assumed to implement it without a corresponding bound.
For this I mostly imitated what the compiler was already doing for the `DiscriminantKind` trait. I’m very unfamiliar with compiler internals, so careful review is appreciated.
This PR also extends the standard library with new unstable APIs in `core::ptr` and `std::ptr`:
```rust
pub trait Pointee {
/// One of `()`, `usize`, or `DynMetadata<dyn SomeTrait>`
type Metadata: Copy + Send + Sync + Ord + Hash + Unpin;
}
pub trait Thin = Pointee<Metadata = ()>;
pub const fn metadata<T: ?Sized>(ptr: *const T) -> <T as Pointee>::Metadata {}
pub const fn from_raw_parts<T: ?Sized>(*const (), <T as Pointee>::Metadata) -> *const T {}
pub const fn from_raw_parts_mut<T: ?Sized>(*mut (),<T as Pointee>::Metadata) -> *mut T {}
impl<T: ?Sized> NonNull<T> {
pub const fn from_raw_parts(NonNull<()>, <T as Pointee>::Metadata) -> NonNull<T> {}
/// Convenience for `(ptr.cast(), metadata(ptr))`
pub const fn to_raw_parts(self) -> (NonNull<()>, <T as Pointee>::Metadata) {}
}
impl<T: ?Sized> *const T {
pub const fn to_raw_parts(self) -> (*const (), <T as Pointee>::Metadata) {}
}
impl<T: ?Sized> *mut T {
pub const fn to_raw_parts(self) -> (*mut (), <T as Pointee>::Metadata) {}
}
/// `<dyn SomeTrait as Pointee>::Metadata == DynMetadata<dyn SomeTrait>`
pub struct DynMetadata<Dyn: ?Sized> {
// Private pointer to vtable
}
impl<Dyn: ?Sized> DynMetadata<Dyn> {
pub fn size_of(self) -> usize {}
pub fn align_of(self) -> usize {}
pub fn layout(self) -> crate::alloc::Layout {}
}
unsafe impl<Dyn: ?Sized> Send for DynMetadata<Dyn> {}
unsafe impl<Dyn: ?Sized> Sync for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> Debug for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> Unpin for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> Copy for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> Clone for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> Eq for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> PartialEq for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> Ord for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> PartialOrd for DynMetadata<Dyn> {}
impl<Dyn: ?Sized> Hash for DynMetadata<Dyn> {}
```
API differences from the RFC, in areas noted as unresolved questions in the RFC:
* Module-level functions instead of associated `from_raw_parts` functions on `*const T` and `*mut T`, following the precedent of `null`, `slice_from_raw_parts`, etc.
* Added `to_raw_parts`
Only store a LocalDefId in some HIR nodes
Some HIR nodes are guaranteed to be HIR owners: Item, TraitItem, ImplItem, ForeignItem and MacroDef.
As a consequence, we do not need to store the `HirId`'s `local_id`, and we can directly store a `LocalDefId`.
This allows to avoid a bit of the dance with `tcx.hir().local_def_id` and `tcx.hir().local_def_id_to_hir_id` mappings.
avoid full-slicing slices
If we already have a slice, there is no need to get another full-range slice from that, just use the original.
clippy::redundant_slicing
const_generics: Fix incorrect ty::ParamEnv::empty() usage
Fixes#80561
Not sure if I should keep the `debug!(..)`s or not but its the second time I've needed them so they sure seem useful lol
cc ``@lcnr``
r? ``@oli-obk``
const_generics: Dont evaluate array length const when handling errors
Fixes#79518Fixes#78246
cc ````@lcnr````
This was ICE'ing because we dont pass in the correct ``ParamEnv`` which meant that there was no ``Self: Foo`` predicate to make ``Self::Assoc`` well formed which caused an ICE when trying to normalize ``Self::Assoc`` in the mir interpreter
r? ````@varkor````
Suggest to create a new `const` item if the `fn` in the array is a `const fn`
Fixes#73734. If the `fn` in the array repeat expression is a `const fn`, suggest creating a new `const` item. On nightly, suggest creating an inline `const` block. This PR also removes the `suggest_const_in_array_repeat_expressions` as it is no longer necessary.
Example:
```rust
fn main() {
// Should not compile but hint to create a new const item (stable) or an inline const block (nightly)
let strings: [String; 5] = [String::new(); 5];
println!("{:?}", strings);
}
```
Gives this error:
```
error[E0277]: the trait bound `std::string::String: std::marker::Copy` is not satisfied
--> $DIR/const-fn-in-vec.rs:3:32
|
2 | let strings: [String; 5] = [String::new(); 5];
| ^^^^^^^^^^^^^^^^^^ the trait `std::marker::Copy` is not implemented for `String`
|
= note: the `Copy` trait is required because the repeated element will be copied
```
With this change, this is the error message:
```
error[E0277]: the trait bound `String: Copy` is not satisfied
--> $DIR/const-fn-in-vec.rs:3:32
|
LL | let strings: [String; 5] = [String::new(); 5];
| ^^^^^^^^^^^^^^^^^^ the trait `Copy` is not implemented for `String`
|
= help: moving the function call to a new `const` item will resolve the error
```
Check the result cache before the DepGraph when ensuring queries
Split out of https://github.com/rust-lang/rust/pull/70951
Calling `ensure` on already forced queries is a common operation.
Looking at the results cache first is faster than checking the DepGraph for a green node.
Try fast_reject::simplify_type in coherence before doing full check
This is a reattempt at landing #69010 (by `@jonas-schievink).` The change adds a fast path for coherence checking to see if there's no way for types to unify since full coherence checking can be somewhat expensive.
This has big effects on code generated by the [`windows`](https://github.com/microsoft/windows-rs) which in some cases spends as much as 20% of compilation time in the `specialization_graph_of` query. In local benchmarks this took a compilation that previously took ~500 seconds down to ~380 seconds.
This is surely not going to make a difference on much smaller crates, so the question is whether it will have a negative impact. #69010 was closed because some of the perf suite crates did show small regressions.
Additional discussion of this issue is happening [here](https://rust-lang.zulipchat.com/#narrow/stream/247081-t-compiler.2Fperformance/topic/windows-rs.20perf).
Rename HIR UnOp variants
This renames the variants in HIR UnOp from
enum UnOp {
UnDeref,
UnNot,
UnNeg,
}
to
enum UnOp {
Deref,
Not,
Neg,
}
Motivations:
- This is more consistent with the rest of the code base where most enum
variants don't have a prefix.
- These variants are never used without the `UnOp` prefix so the extra
`Un` prefix doesn't help with readability. E.g. we don't have any
`UnDeref`s in the code, we only have `UnOp::UnDeref`.
- MIR `UnOp` type variants don't have a prefix so this is more
consistent with MIR types.
- "un" prefix reads like "inverse" or "reverse", so as a beginner in
rustc code base when I see "UnDeref" what comes to my mind is
something like `&*` instead of just `*`.
This renames the variants in HIR UnOp from
enum UnOp {
UnDeref,
UnNot,
UnNeg,
}
to
enum UnOp {
Deref,
Not,
Neg,
}
Motivations:
- This is more consistent with the rest of the code base where most enum
variants don't have a prefix.
- These variants are never used without the `UnOp` prefix so the extra
`Un` prefix doesn't help with readability. E.g. we don't have any
`UnDeref`s in the code, we only have `UnOp::UnDeref`.
- MIR `UnOp` type variants don't have a prefix so this is more
consistent with MIR types.
- "un" prefix reads like "inverse" or "reverse", so as a beginner in
rustc code base when I see "UnDeref" what comes to my mind is
something like "&*" instead of just "*".
Rollup of 11 pull requests
Successful merges:
- #72209 (Add checking for no_mangle to unsafe_code lint)
- #80732 (Allow Trait inheritance with cycles on associated types take 2)
- #81697 (Add "every" as a doc alias for "all".)
- #81826 (Prefer match over combinators to make some Box methods inlineable)
- #81834 (Resolve typedef in HashMap lldb pretty-printer only if possible)
- #81841 ([rustbuild] Output rustdoc-json-types docs )
- #81849 (Expand the docs for ops::ControlFlow a bit)
- #81876 (parser: Fix panic in 'const impl' recovery)
- #81882 (⬆️ rust-analyzer)
- #81888 (Fix pretty printer macro_rules with semicolon.)
- #81896 (Remove outdated comment in windows' mutex.rs)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Allow Trait inheritance with cycles on associated types take 2
This reverts the revert of #79209 and fixes the ICEs that's occasioned by that PR exposing some problems that are addressed in #80648 and #79811.
For easier review I'd say, check only the last commit, the first one is just a revert of the revert of #79209 which was already approved.
This also could be considered part or the actual fix of #79560 but I guess for that to be closed and fixed completely we would need to land #80648 and #79811 too.
r? `@nikomatsakis`
cc `@Aaron1011`
Improve SIMD type element count validation
Resolvesrust-lang/stdsimd#53.
These changes are motivated by `stdsimd` moving in the direction of const generic vectors, e.g.:
```rust
#[repr(simd)]
struct SimdF32<const N: usize>([f32; N]);
```
This makes a few changes:
* Establishes a maximum SIMD lane count of 2^16 (65536). This value is arbitrary, but attempts to validate lane count before hitting potential errors in the backend. It's not clear what LLVM's maximum lane count is, but cranelift's appears to be much less than `usize::MAX`, at least.
* Expands some SIMD intrinsics to support arbitrary lane counts. This resolves the ICE in the linked issue.
* Attempts to catch invalid-sized vectors during typeck when possible.
Unresolved questions:
* Generic-length vectors can't be validated in typeck and are only validated after monomorphization while computing layout. This "works", but the errors simply bail out with no context beyond the name of the type. Should these errors instead return `LayoutError` or otherwise provide context in some way? As it stands, users of `stdsimd` could trivially produce monomorphization errors by making zero-length vectors.
cc `@bjorn3`
Add lint for `panic!(123)` which is not accepted in Rust 2021.
This extends the `panic_fmt` lint to warn for all cases where the first argument cannot be interpreted as a format string, as will happen in Rust 2021.
It suggests to add `"{}",` to format the message as a string. In the case of `std::panic!()`, it also suggests the recently stabilized
`std::panic::panic_any()` function as an alternative.
It renames the lint to `non_fmt_panic` to match the lint naming guidelines.
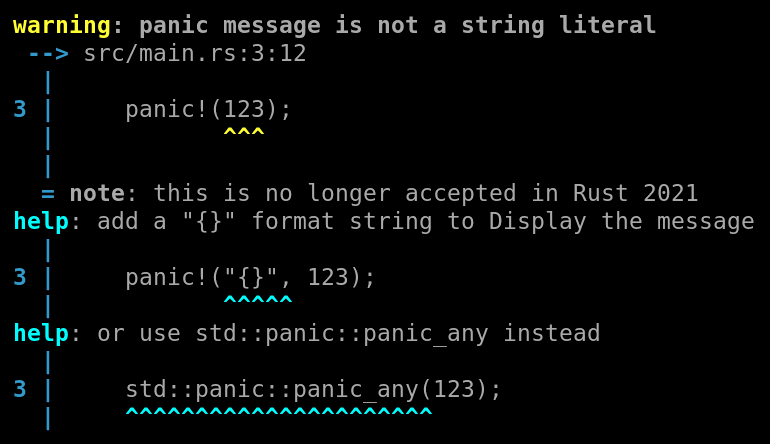
This is part of #80162.
r? ```@estebank```
introduce future-compatibility warning for forbidden lint groups
We used to ignore `forbid(group)` scenarios completely. This changed in #78864, but that led to a number of regressions (#80988, #81218).
This PR introduces a future compatibility warning for the case where a group is forbidden but then an individual lint within that group is allowed. We now issue a FCW when we see the "allow", but permit it to take effect.
r? ``@Mark-Simulacrum``
Add a new ABI to support cmse_nonsecure_call
This adds support for the `cmse_nonsecure_call` feature to be able to perform non-secure function call.
See the discussion on Zulip [here](https://rust-lang.zulipchat.com/#narrow/stream/131828-t-compiler/topic/Support.20for.20callsite.20attributes/near/223054928).
This is a followup to #75810 which added `cmse_nonsecure_entry`. As for that PR, I assume that the changes are small enough to not have to go through a RFC but I don't mind doing one if needed 😃
I did not yet create a tracking issue, but if most of it is fine, I can create one and update the various files accordingly (they refer to the other tracking issue now).
On the Zulip chat, I believe `@jonas-schievink` volunteered to be a reviewer 💯
We used to ignore `forbid(group)` scenarios completely. This changed
in #78864, but that led to a number of regressions (#80988, #81218).
This PR introduces a future compatibility warning for the case where
a group is forbidden but then an individual lint within that group
is allowed. We now issue a FCW when we see the "allow", but permit
it to take effect.
Upgrade Chalk
~~Blocked on rust-lang/chalk#670~~
~~Now blocked on rust-lang/chalk#680 and release~~
In addition to the straight upgrade, I also tried to fix some tests by properly returning variables and max universes in the solution. Unfortunately, this actually triggers the same perf problem that rustc traits code runs into in `canonicalizer`. Not sure what the root cause of this problem is, or why it's supposed to be solved in chalk.
r? ```@nikomatsakis```
This commit adds a new ABI to be selected via `extern
"C-cmse-nonsecure-call"` on function pointers in order for the compiler to
apply the corresponding cmse_nonsecure_call callsite attribute.
For Armv8-M targets supporting TrustZone-M, this will perform a
non-secure function call by saving, clearing and calling a non-secure
function pointer using the BLXNS instruction.
See the page on the unstable book for details.
Signed-off-by: Hugues de Valon <hugues.devalon@arm.com>
Remove the remains of query categories
Back in October 2020 in #77830 ``@cjgillot`` removed the query categories information from the profiler, but the actual definitions which query was in which category remained, although unused.
Here I clean that up, to simplify the query definitions even further.
It's unfortunate that this loses all the context for `git blame`, ~~but I'm working on moving those query definitions into `rustc_query_system`, which will lose that context anyway.~~ EDIT: Might not work out.
The functional changes are in the first commit. The second one only changes the indentation.
Add visitors for checking #[inline]
Add visitors for checking #[inline] with struct field
Fix test for #[inline]
Add visitors for checking #[inline] with #[macro_export] macro
Add visitors for checking #[inline] without #[macro_export] macro
Add use alias with Visitor
Fix lint error
Reduce unnecessary variable
Co-authored-by: LingMan <LingMan@users.noreply.github.com>
Change error to warning
Add warning for checking field, arm with #[allow_internal_unstable]
Add name resolver
Formatting
Formatting
Fix error fixture
Add checking field, arm, macro def
Remove const_in_array_repeat
Fixes#80371. Fixes#81315. Fixes#80767. Fixes#75682.
I thought there might be some issue with `Repeats(_, 0)`, but if you increase the items in the array it still ICEs. I'm not sure if this is the best fix but it does fix the given issue.
2229: Fix issues with move closures and mutability
This PR fixes two issues when feature `capture_disjoint_fields` is used.
1. Can't mutate using a mutable reference
2. Move closures try to move value out through a reference.
To do so, we
1. Compute the mutability of the capture and store it as part of the `CapturedPlace` that is written in TypeckResults
2. Restrict capture precision. Note this is temporary for now, to allow the feature to be used with move closures and ByValue captures and might change depending on discussions with the lang team.
- No Derefs are captured for ByValue captures, since that will result in value behind a reference getting moved.
- No projections are applied to raw pointers since these require unsafe blocks. We capture
them completely.
r? `````@nikomatsakis`````
Stabilize `unsigned_abs`
Resolves#74913.
This PR stabilizes the `i*::unsigned_abs()` method, which returns the absolute value of an integer _as its unsigned equivalent_. This has the advantage that it does not overflow on `i*::MIN`.
I have gone ahead and used this in a couple locations throughout the repository.
When `capture_disjoint_fields` is not enabled, checking if the root variable
binding is mutable would suffice.
However with the feature enabled, the captured place might be mutable
because it dereferences a mutable reference.
This PR computes the mutability of each capture after capture analysis
in rustc_typeck. We store this in `ty::CapturedPlace` and then use
`ty::CapturedPlace::mutability` in mir_build and borrow_check.
Refractor a few more types to `rustc_type_ir`
In the continuation of #79169, ~~blocked on that PR~~.
This PR:
- moves `IntVarValue`, `FloatVarValue`, `InferTy` (and friends) and `Variance`
- creates the `IntTy`, `UintTy` and `FloatTy` enums in `rustc_type_ir`, based on their `ast` and `chalk_ir` equilavents, and uses them for types in the rest of the compiler.
~~I will split up that commit to make this easier to review and to have a better commit history.~~
EDIT: done, I split the PR in commits of 200-ish lines each
r? `````@nikomatsakis````` cc `````@jackh726`````
Enforce that query results implement Debug
Currently, we require that query keys implement `Debug`, but we do not do the same for query values. This can make incremental compilation bugs difficult to debug - there isn't a good place to print out the result loaded from disk.
This PR adds `Debug` bounds to several query-related functions, allowing us to debug-print the query value when an 'unstable fingerprint' error occurs. This required adding `#[derive(Debug)]` to a fairly large number of types - hopefully, this doesn't have much of an impact on compiler bootstrapping times.
Prevent query cycles in the MIR inliner
r? `@eddyb` `@wesleywiser`
cc `@rust-lang/wg-mir-opt`
The general design is that we have a new query that is run on the `validated_mir` instead of on the `optimized_mir`. That query is forced before going into the optimization pipeline, so as to not try to read from a stolen MIR.
The query should not be cached cross crate, as you should never call it for items from other crates. By its very design calls into other crates can never cause query cycles.
This is a pessimistic approach to inlining, since we strictly have more calls in the `validated_mir` than we have in `optimized_mir`, but that's not a problem imo.
Various ABI refactorings
This includes changes to the rust abi and various refactorings that will hopefully make it easier to use the abi handling infrastructure of rustc in cg_clif. There are several refactorings that I haven't done. I am opening this draft PR to check that I haven't broken any non x86_64 architectures.
r? `@ghost`