Rework `no_coverage` to `coverage(off)`
As discussed at the tail of https://github.com/rust-lang/rust/issues/84605 this replaces the `no_coverage` attribute with a `coverage` attribute that takes sub-parameters (currently `off` and `on`) to control the coverage instrumentation.
Allows future-proofing for things like `coverage(off, reason="Tested live", issue="#12345")` or similar.
Allow redirecting subprocess stdout to our stderr etc. (redux)
This is the code from #88561, tidied up, including review suggestions, and with the for-testing-only CI commit removed. FCP for the API completed in #88561.
I have made a new MR to facilitate review. The discussion there is very cluttered and the branch is full of changes (in many cases as a result of changes to other Rust stdlib APIs since then). Assuming this MR is approvedl we should close that one.
### Reviewer doing a de novo review
Just code review these four commits.. FCP discussion starts here: https://github.com/rust-lang/rust/pull/88561#issuecomment-1640527595
Portability tests: you can see that this branch works on Windows too by looking at the CI results in #88561, which has the same code changes as this branch but with an additional "DO NOT MERGE" commit to make the Windows tests run.
### Reviewer doing an incremental review from some version of #88561
Review the new commits since your last review. I haven't force pushed the branch there.
git diff the two branches (eg `git diff 176886197d6..0842b69c219`). You'll see that the only difference is in gitlab CI files. You can also see that *this* MR doesn't touch those files.
This implementation is wrong. Like the impl for From<File>, it is
forced to panic because process::Stdio in unsupported/process.rs
doesn't have a suitable variant.
The root cause of the problem is that process::Stdio in
unsupported/process.rs has any information in it at all.
I'm pretty sure that it should just be a unit struct. However,
making that build on all platforms is going to be a lot of work,
iterating through CI and/or wrestling Docker.
I don't think this extra panic is making things significantly worse.
For now I have added some TODOs.
Clarify stability guarantee for lifetimes in enum discriminants
Since `std::mem::Discriminant` erases lifetimes, it should be clarified that changing the concrete value of a lifetime parameter does not change the value of an enum discriminant for a given variant. This is useful as it guarantees that it is safe to transmute `Discriminant<Foo<'a>>` to `Discriminant<Foo<'b>>` for any combination of `'a` and `'b`. This also holds for type-generics as long as the type parameters do not change, e.g. `Discriminant<Foo<T, 'a>>` can be transmuted to `Discriminant<Foo<T, 'b>>`.
Side note: Is what I've written actually enough to imply soundness (or rather codify it), or should it specifically be spelled out that it's OK to transmute in the above way?
Lint on invalid usage of `UnsafeCell::raw_get` in reference casting
This PR proposes to take into account `UnsafeCell::raw_get` method call for non-Freeze types for the `invalid_reference_casting` lint.
The goal of this is to catch those kind of invalid reference casting:
```rust
fn as_mut<T>(x: &T) -> &mut T {
unsafe { &mut *std::cell::UnsafeCell::raw_get(x as *const _ as *const _) }
//~^ ERROR casting `&T` to `&mut T` is undefined behavior
}
```
r? `@est31`
Update stdarch submodule and remove special handling in cranelift codegen for some AVX and SSE2 LLVM intrinsics
https://github.com/rust-lang/stdarch/pull/1463 reimplemented some x86 intrinsics to avoid using some x86-specific LLVM intrinsics:
* Store unaligned (`_mm*_storeu_*`) use `<*mut _>::write_unaligned` instead of `llvm.x86.*.storeu.*`.
* Shift by immediate (`_mm*_s{ll,rl,ra}i_epi*`) use `if` (srl, sll) or `min` (sra) to simulate the behaviour when the RHS is out of range. RHS is constant, so the `if`/`min` will be optimized away.
This PR updates the stdarch submodule to pull these changes and removes special handling for those LLVM intrinsics from cranelift codegen. I left gcc codegen untouched because there are some autogenerated lists.
Move RawOsError defination to sys
This was originally a part of https://github.com/rust-lang/rust/pull/105861, but I feel it should be its own PR since the raw os error is still unstable.
Outline panicking code for `RefCell::borrow` and `RefCell::borrow_mut`
This outlines panicking code for `RefCell::borrow` and `RefCell::borrow_mut` to reduce code size.
Use std::io::Error::is_interrupted everywhere
In https://github.com/rust-lang/rust/pull/115228 I introduced this helper and started using it, this PR uses it to replace all applicable uses of `std::io::Error::kind`. The justification is the same; for whatever reason LLVM totally flops optimizing `Error::kind` so it's nice to use it less.
FYI ``@mkroening`` I swear the hermit changes look good, but I was so sure about the previous PR.
RangeFull: Remove parens around .. in documentation snippet
I’ve removed unnecessary parentheses in a documentation snippet documenting `RangeFull`. It could’ve lead people to believe the parentheses were necessary.
kmc-solid: Fix `is_interrupted`
Follow-up to #115228. Fixes a build error in [`*-kmc-solid_*`](https://doc.rust-lang.org/nightly/rustc/platform-support/kmc-solid.html) Tier 3 targets.
```
error[E0603]: function `is_interrupted` is private
--> library\std\src\sys\solid\mod.rs:77:12
|
77 | error::is_interrupted(code)
| ^^^^^^^^^^^^^^ private function
|
note: the function `is_interrupted` is defined here
--> library\std\src\sys\solid\error.rs:35:1
|
35 | fn is_interrupted(er: abi::ER) -> bool {
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
```
Add alignment to the NPO guarantee
This PR [changes](https://github.com/rust-lang/rust/pull/114845#discussion_r1294363357) "same size" to "same size and alignment" in the option module's null pointer optimization docs in <https://doc.rust-lang.org/std/option/#representation>.
As far as I know, this has been true for a long time in the actual rustc implementation, but it's not in the text of those docs, so I figured I'd bring this up to FCP it.
I also see no particular reason that we'd ever *want* to have higher alignment on these. In many of the cases it's impossible, as the minimum alignment is already the size of the type, but even if we *could* do things like on 32-bit we could say that `NonZeroU64` is 4-align but `Option<NonZeroU64>` is 8-align, I just don't see any value in doing that, so feel completely fine closing this door for the few things on which the NPO is already guaranteed. These are basically all primitives, and should end up with the same size & alignment as those primitives.
(There's no layout guarantee for something like `Option<[u8; 3]>`, where it'd be at least plausible to consider raising the alignment from 1 to 4 on, say, some hypothetical target that doesn't have efficient unaligned 4-byte load/stores. And even if we ever did start to offer some kind of guarantee around such a type, I doubt we'd put it under the "null pointer" optimization header.)
Screenshots for the new examples:
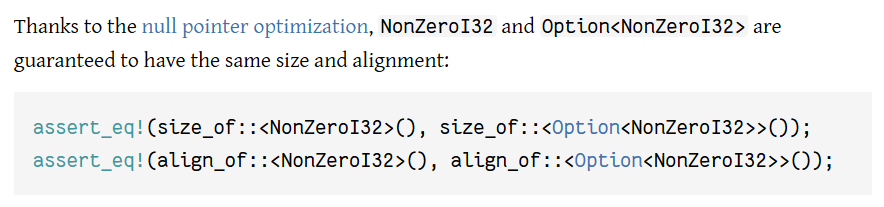
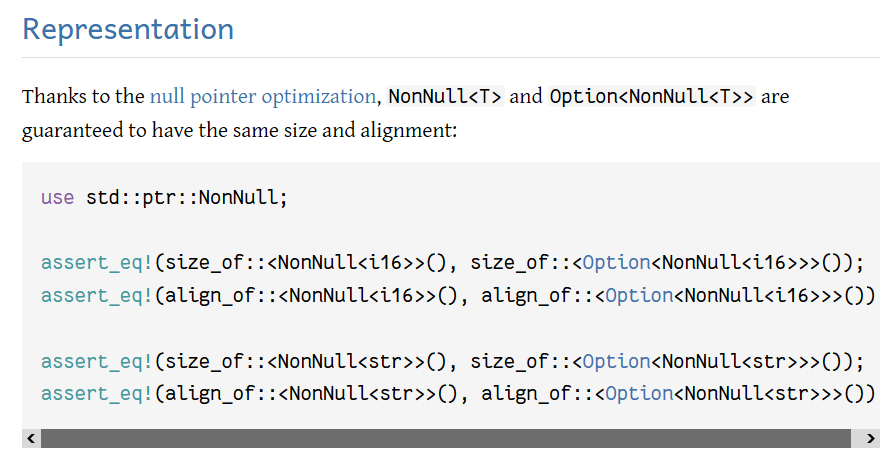
Implement Step for ascii::Char
This allows iterating over ranges of `ascii::Char`, similarly to ranges of `char`.
Note that `ascii::Char` is still unstable, tracked in #110998.
docs: improve std::fs::read doc
#### What does this PR do
1. Rephrase a confusing sentence in the document of `std::fs::read()`
-----
Closes#114432
cc `@Dexus0` `@saethlin`
Add note that Vec::as_mut_ptr() does not materialize a reference to the internal buffer
See discussion on https://github.com/thomcc/rust-typed-arena/issues/62 and [t-opsem](https://rust-lang.zulipchat.com/#narrow/stream/136281-t-opsem/topic/is.20this.20typed_arena.20code.20sound.20under.20stacked.2Ftree.20borrows.3F)
This method already does the correct thing here, but it is worth guaranteeing that it does so it can be used more freely in unsafe code without having to worry about potential Stacked/Tree Borrows violations. This moves one more unsafe usage pattern from the "very likely sound but technically not fully defined" box into "definitely sound", and currently our surface area of the latter is woefully small.
I'm not sure how best to word this, opening this PR as a way to start discussion.