Use u32 over Option<u32> in DebugLoc
~~Changes `Option<u32>` fields in `DebugLoc` to `Option<NonZeroU32>`. Since the respective fields (`line` and `col`) are guaranteed to be 1-based, this layout optimization is a freebie.~~
EDIT: Changes `Option<u32>` fields in `DebugLoc` to `u32`. As `@bugadani` pointed out, an `Option<NonZeroU32>` is probably an unnecessary layer of abstraction since the `None` variant is always used as `UNKNOWN_LINE_NUMBER` (which is just `0`). Also, `SourceInfo` in `metadata.rs` already uses a `u32` instead of an `Option<u32>` to encode the same information, so I think this change is warranted.
Since `@jyn514` raised some concerns over measuring performance in a similar PR (#82255), does this need a perf run?
Sync rustc_codegen_cranelift
The main highlight of this sync is removal of support for the old x86 Cranelift backend. This made it possible to use native atomic instructions rather than hackishly using a global mutex. 128bit integer support has also seen a few bugfixes and performance improvements. And finally I have formatted everything using the same rustfmt config as the rest of this repo.
r? ````@ghost````
````@rustbot```` label +A-codegen +A-cranelift +T-compiler
Cleanup rustdoc warnings
## Clean up error reporting for deprecated passes
Using `error!` here goes all the way back to the original commit, https://github.com/rust-lang/rust/pull/8540. I don't see any reason to use logging; rustdoc should use diagnostics wherever possible. See https://github.com/rust-lang/rust/pull/81932#issuecomment-785291244 for further context.
- Use spans for deprecated attributes
- Use a proper diagnostic for unknown passes, instead of error logging
- Add tests for unknown passes
- Improve some wording in diagnostics
## Report that `doc(plugins)` doesn't work using diagnostics instead of `eprintln!`
This also adds a test for the output.
This was added in https://github.com/rust-lang/rust/pull/52194. I don't see any particular reason not to use diagnostics here, I think it was just missed in https://github.com/rust-lang/rust/pull/50541.
Change built-in kernel targets to be os = none throughout
Whether for Rust's own `target_os`, LLVM's triples, or GNU config's, the
OS-related have fields have been for code running *on* that OS, not code
hat is *part* of the OS.
The difference is huge, as syscall interfaces are nothing like
freestanding interfaces. Kernels are (hypervisors and other more exotic
situations aside) freestanding programs that use the interfaces provided
by the hardware. It's *those* interfaces, the ones external to the
program being built and its software dependencies, that are the content
of the target.
For the Linux Kernel in particular, `target_env: "gnu"` is removed for
the same reason: that `-gnu` refers to glibc or GNU/linux, neither of
which applies to the kernel itself.
Relates to #74247
Move check only relevant in error case out of critical path
Move the check for potentially forgotten `return` in a tail expression
of arbitrary expressions into the coercion error branch to avoid
computing unncessary coercion checks on successful code.
Follow up to #81458.
Rust contains various size checks conditional on target_arch = "x86_64",
but these checks were never intended to apply to
x86_64-unknown-linux-gnux32. Add target_pointer_width = "64" to the
conditions.
Warn on `#![doc(test(...))]` on items other than the crate root and use future incompatible lint
Part of #82672.
This PR does multiple things:
* Create a new `INVALID_DOC_ATTRIBUTE` lint which is also "future incompatible", allowing us to use it as a warning for the moment until it turns (eventually) into a hard error.
* Use this link when `#![doc(test(...))]` isn't used at the crate level.
* Make #82702 use this new lint as well.
r? ``@jyn514``
When rustdoc lints were changed to be tool lints, the `rustdoc` group
was removed, leading to spurious warnings like
```
warning: unknown lint: `rustdoc`
```
The lint group still worked when rustdoc ran, since rustdoc added the group itself.
This renames the group to `rustdoc::all` for consistency with
`clippy::all` and the rest of the rustdoc lints.
Add diagnostic item to `Default` trait
This PR adds diagnostic item to `Default` trait to be used by rust-lang/rust-clippy#6562 issue.
Also fixes the obsolete path to the `symbols.rs` file in the comment.
Add suggestion `.collect()` for iterators in iterators
Closes#81584
```
error[E0515]: cannot return value referencing function parameter `y`
--> main3.rs:4:38
|
4 | ... .map(|y| y.iter().map(|x| x + 1))
| -^^^^^^^^^^^^^^^^^^^^^^
| |
| returns a value referencing data owned by the current function
| `y` is borrowed here
| help: Maybe use `.collect()` to allocate the iterator
```
Added the suggestion: `help: Maybe use `.collect()` to allocate the iterator`
resolve: Reduce scope of `pub_use_of_private_extern_crate` deprecation lint
This lint was deny-by-default since July 2017, crater showed 7 uses on crates.io back then (https://github.com/rust-lang/rust/pull/42894#issuecomment-311921147).
Unfortunately, the construction `pub use foo as bar` where `foo` is `extern crate foo;` was used by an older version `bitflags`, so turning it into an error causes too many regressions.
So, this PR reduces the scope of the lint instead of turning it into a hard error, and only turns some more rarely used components of it into errors.
Implement NOOP_METHOD_CALL lint
Implements the beginnings of https://github.com/rust-lang/lang-team/issues/67 - a lint for detecting noop method calls (e.g, calling `<&T as Clone>::clone()` when `T: !Clone`).
This PR does not fully realize the vision and has a few limitations that need to be addressed either before merging or in subsequent PRs:
* [ ] No UFCS support
* [ ] The warning message is pretty plain
* [ ] Doesn't work for `ToOwned`
The implementation uses [`Instance::resolve`](https://doc.rust-lang.org/nightly/nightly-rustc/rustc_middle/ty/instance/struct.Instance.html#method.resolve) which is normally later in the compiler. It seems that there are some invariants that this function relies on that we try our best to respect. For instance, it expects substitutions to have happened, which haven't yet performed, but we check first for `needs_subst` to ensure we're dealing with a monomorphic type.
Thank you to ```@davidtwco,``` ```@Aaron1011,``` and ```@wesleywiser``` for helping me at various points through out this PR ❤️.
Inherit `#[stable(..)]` annotations in enum variants and fields from its item
Lint changes for #65515. The stdlib will have to be updated once this lands in beta and that version is promoted in master.
This ownership kind is only constructed in the case of path attributes like `#[path = ".."]` without a file name segment, which always represent some kind of directories and will produce and error on attempt to parse them as a module file.
Fix polymorphization ICE on associated types in trait decls using const generics in bounds
r? `@davidtwco`
only the last commit actually changes something
Upgrade to LLVM 12
This implements the necessary adjustments to make rustc work with LLVM 12. I didn't encounter any major issues so far.
r? `@cuviper`
Pass callee expr to `confirm_builtin_call`. This removes a partial
pattern match in `confirm_builtin_call` and the `panic` in the `else`
branch. The diff is large because of indentation changes caused by
removing the if-let.
Add `-Z unpretty` flags for the AST
Implements rust-lang/compiler-team#408.
Builds on #82269, but if that PR is rejected or stalls out, I can implement this without #82269.
cc rust-lang/rustc-dev-guide#1062
Move the check for potentially forgotten `return` in a tail expression
of arbitrary expressions into the coercion error branch to avoid
computing unncessary coercion checks on successful code.
Follow up to #81458.
This works around a design defect in the LLVM 12 pass builder
implementation. In LLVM 13, the PreLink ThinLTO pipeline properly
respects the OptimizerLastEPCallbacks.
Update tracing to 0.1.25
* Update tracing from 0.1.18 to 0.1.25
* Update tracing-subscriber from 0.2.13 to 0.2.16
* Update tracing-tree from 0.1.6 to 0.1.8
* Add pin-project-lite to the list of allowed dependencies (it is now a direct dependency of tracing).
Revert non-power-of-two vector restriction
Removes the power of two restriction from rustc. As discussed in https://github.com/rust-lang/stdsimd/issues/63
r? ```@calebzulawski```
cc ```@workingjubilee``` ```@thomcc```
Optimize counting digits in line numbers during error reporting further
This one-ups #82248 by switching the strategy: Instead of dividing the value by 10 repeatedly, we compare with a limit that we multiply by 10 repeatedly. In my benchmarks, this took between 50% and 25% of the original time. The reasons for being faster are:
1. While LLVM is able to replace a division by constant with a multiply + shift, a plain multiplication is still faster. However, this doesn't even factor, because
2. Multiplication, unlike division, is const. We also use a simple for-loop instead of a more complex loop + break, which allows
3. rustc to const-fold the whole loop, and indeed the assembly output simply shows a series of comparisons.
Rollup of 10 pull requests
Successful merges:
- #80189 (Convert primitives in the standard library to intra-doc links)
- #80874 (Update intra-doc link documentation to match the implementation)
- #82376 (Add option to enable MIR inlining independently of mir-opt-level)
- #82516 (Add incomplete feature gate for inherent associate types.)
- #82579 (Fix turbofish recovery with multiple generic args)
- #82593 (Teach rustdoc how to display WASI.)
- #82597 (Get TyCtxt from self instead of passing as argument in AutoTraitFinder)
- #82627 (Erase late bound regions to avoid ICE)
- #82661 (⬆️ rust-analyzer)
- #82691 (Update books)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Add incomplete feature gate for inherent associate types.
Mentored by ``````@oli-obk``````
So far the only change is that instead of giving an automatic error, the following code compiles:
```rust
struct Foo;
impl Foo {
type Bar = isize;
}
```
The backend work to make it actually usable isn't there yet. In particular, this:
```rust
let x : Foo::Bar;
```
will give you:
```sh
error[E0223]: ambiguous associated type
--> /$RUSTC_DIR/src/test/ui/assoc-inherent.rs:15:13
|
LL | let x : Foo::Bar;
| ^^^^^^^^ help: use fully-qualified syntax: `<Foo as Trait>::Bar`
```
Add option to enable MIR inlining independently of mir-opt-level
Add `-Zinline-mir` option that enables MIR inlining independently of the
current MIR opt level. The primary use-case is enabling MIR inlining on the
default MIR opt level.
Turn inlining thresholds into optional values to make it possible to configure
different defaults depending on the current mir-opt-level (although thresholds
are yet to be used in such a manner).
Remove an old FIXME comment and inline attribute
Apparently #35870 caused a problem in this code (which originally
returned an impl trait) and `#[inline]` was added as a workaround, in
ade79d7609.
The issue is now fixed and the comment and `#[inline]` can now be
removed.
Note that the FIXME was removed because this can't be fixed,
`register_renamed` calls LintId::of and there's no LintId for rustdoc
lints when rustc is running.
- Move MISSING_CRATE_LEVEL_DOCS to rustdoc directly
- Update documentation
This also takes the opportunity to make the `no-crate-level-doc-lint`
test more specific.
- Use `register_renamed` when rustdoc is running so the lint will still
be active and use a structured suggestion
- Test the behavior for rustc, not just for rustdoc (because it differs)
- Rename `broken_intra_doc_links` to `rustdoc::broken_intra_doc_links`
- Ensure that the old lint names still work and give deprecation errors
- Register lints even when running doctests
Otherwise, all `rustdoc::` lints would be ignored.
- Register all existing lints as removed
This unfortunately doesn't work with `register_renamed` because tool
lints have not yet been registered when rustc is running. For similar
reasons, `check_backwards_compat` doesn't work either. Call
`register_removed` directly instead.
- Fix fallout
+ Rustdoc lints for compiler/
+ Rustdoc lints for library/
Note that this does *not* suggest `rustdoc::broken_intra_doc_links` for
`rustdoc::intra_doc_link_resolution_failure`, since there was no time
when the latter was valid.
Highlight identifier span instead of whole pattern span in `unused` lint
Fixes#81314
This pretty much just changes the span highlighted in the lint from `pat_sp` to `ident.span`. There's however an exception, which is in patterns with shorthands like `Point { y, ref mut x }`, where a suggestion to change just `x` would be invalid; in those cases I had to keep the pattern span. Another option would be suggesting something like `Point { y, x: ref mut _x }`.
I also added a new test since there weren't any test that checked the `unused` lint with optional patterns.
The definition of this struct changes in LLVM 12 due to the addition
of branch coverage support. To avoid future mismatches, declare our
own struct and then convert between them.
Whether for Rust's own `target_os`, LLVM's triples, or GNU config's, the
OS-related have fields have been for code running *on* that OS, not code
that is *part* of the OS.
The difference is huge, as syscall interfaces are nothing like
freestanding interfaces. Kernels are (hypervisors and other more exotic
situations aside) freestanding programs that use the interfaces provided
by the hardware. It's *those* interfaces, the ones external to the
program being built and its software dependencies, that are the content
of the target.
For the Linux Kernel in particular, `target_env: "gnu"` is removed for
the same reason: that `-gnu` refers to glibc or GNU/linux, neither of
which applies to the kernel itself.
Relates to #74247
Thanks @ojeda for catching some things.
Apply lint restrictions from renamed lints
Previously, if you denied the old name of a renamed lint, it would warn
about using the new name, but otherwise do nothing. Now, it will behave
the same as if you'd used the new name.
Fixes https://github.com/rust-lang/rust/issues/82615.
r? `@ehuss`
make x86_64-pc-solaris the default target for x86-64 Solaris
This change makes `x86_64-pc-solaris` the default compilation target for x86-64 Solaris/Illumos (based on [this exchange](https://github.com/rust-lang/rust/issues/68214#issuecomment-748042054) with `@varkor).`
I tried several ways of doing this (leveraging the alias support added with #61761 and improved/fixed with #80073) and found out that cross-compilation to the new one is by far the simplest way of doing this. It can be achieved by adding the following arguments: `--build x86_64-sun-solaris --host x86_64-pc-solaris --target x86_64-pc-solaris` and enabling the cross compilation with `PKG_CONFIG_ALLOW_CROSS=1` environment variable.
I also removed alias support altogether - `x86_64-pc-solaris` and `x86_64-sun-solaris` are now two separate targets. The problem with aliases is that even if rust internally knows that two are the same, other tools building with rust don't know that, resulting in build issues like the one with firefox mentioned [here](https://github.com/rust-lang/rust/issues/68214#issuecomment-746144229). I think that once the dust settles and `x86_64-pc-solaris` becomes the default, `x86_64-sun-solaris` can be removed.
If you agree with the above, I have two subsequent questions:
1. Is there a preferred way to display deprecation warnings when `x86_64-sun-solaris` is passed into the compiler as an argument? I am not sure whether target deprecation was done before.
2. Where would be the best way to document this change for those using rust on Solaris? Without the cross-compilation arguments (used once to build a new version), the build won't work. Should I add it into [RELEASES.md](https://github.com/rust-lang/rust/blob/master/RELEASES.md)?
Thanks!
Remove storage markers if they won't be used during code generation
The storage markers constitute a substantial portion of all MIR
statements. At the same time, for builds without any optimizations,
the storage markers have no further use during and after MIR
optimization phase.
If storage markers are not necessary for code generation, remove them.
Fixed support for macOS Catalyst on ARM64
When I initially added Arm64 Catalyst support in https://github.com/rust-lang/rust/pull/77484 I had access to a DTK. However, while waiting to merge the PR some other changes were merged which caused conflicts in the branch. When fixing those conflicts I had no access to the DTK anymore and didn't try out if the resulting binaries did indeed work on Apple Silicon. I finally have a M1 and I realized that some small changes were necessary to support Apple Silicon. This PR adds the required changes. I've been running binaries generated with this branch for some time now and they work without issues.
Apparently #35870 caused a problem in this code (which originally
returned an impl trait) and `#[inline]` was added as a workaround, in
ade79d7609.
The issue is now fixed and the comment and `#[inline]` can now be
removed.
The first argument to an x86-interrupt ABI function was implicitly
treated as byval prior to LLVM 12. Since LLVM 12, it has to be
marked as such explicitly: 2e0e03c6a0
Previously, if you denied the old name of a renamed lint, it would warn
about using the new name, but otherwise do nothing. Now, it will behave
the same as if you'd used the new name.
Remove the x86_64-rumprun-netbsd target
Herein we remove the target from the compiler and the code from libstd intended to support the now-defunct rumprun project.
Closes#81514
The storage markers constitute a substantial portion of all MIR
statements. At the same time, for builds without any optimizations,
the storage markers have no further use during and after MIR
optimization phase.
If storage markers are not necessary for code generation, remove them.
Update measureme dependency to the latest version
This version adds the ability to use `rdpmc` hardware-based performance
counters instead of wall-clock time for measuring duration. This also
introduces a dependency on the `perf-event-open-sys` crate on Linux
which is used when using hardware counters.
r? ```@oli-obk```
Link crtbegin/crtend on musl to terminate .eh_frame
For some targets, rustc uses a "CRT fallback", where it links CRT
object files it ships instead of letting the host compiler link
them.
On musl, rustc currently links crt1, crti and crtn (provided by
libc), but does not link crtbegin and crtend (provided by libgcc).
In particular, crtend is responsible for terminating the .eh_frame
section. Lack of terminator may result in segfaults during
unwinding, as reported in #47551 and encountered by the LLVM 12
update in #81451.
This patch links crtbegin and crtend for musl as well, following
the table at the top of crt_objects.rs.
r? ``@nagisa``
Suggest character encoding is incorrect when encountering random null bytes
This adds a note whenever null bytes are seen at the start of a token unexpectedly, since those tend to come from UTF-16 encoded files without a [BOM](https://en.wikipedia.org/wiki/Byte_order_mark) (if a UTF-16 BOM appears it won't be valid UTF-8, but if there is no BOM it be both valid UTF-16 and valid but garbled UTF-8). This approach was suggested in https://github.com/rust-lang/rust/issues/73979#issuecomment-653976451.
Closes#73979.
Skip Ty w/o infer ty/const in trait select
Remove some allocations & also add `skip_current_subtree` to skip subtrees with no inferred items.
r? `@eddyb` since marked in the FIXME
This adds recovery when in array type syntax user writes
[X; Y<Z, ...>]
instead of
[X; Y::<Z, ...>]
Fixes#82566
Note that whenever we parse an expression and know that the next token
cannot be `,`, we should be calling
check_mistyped_turbofish_with_multiple_type_params for this recovery.
Previously we only did this for statement parsing (e.g. `let x = f<a,
b>;`). We now also do it when parsing the length field in array type
syntax.
check_mistyped_turbofish_with_multiple_type_params was previously
expecting type arguments between angle brackets, which is not right, as
we can also see const expressions. We now use generic argument parser
instead of type parser.
Test with one, two, and three generic arguments added to check
consistentcy between
1. check_no_chained_comparison: Called after parsing a nested binop
application like `x < A > ...` where angle brackets are interpreted as
binary operators and `A` is an expression.
2. check_mistyped_turbofish_with_multiple_type_params: called by
`parse_full_stmt` when we expect to see a semicolon after parsing an
expression but don't see it.
(In `T2<1, 2>::C;`, the expression is `T2 < 1`)
When token-based attribute handling is implemeneted in #80689,
we will need to access tokens from `HasAttrs` (to perform
cfg-stripping), and we will to access attributes from `HasTokens` (to
construct a `PreexpTokenStream`).
This PR merges the `HasAttrs` and `HasTokens` traits into a new
`AstLike` trait. The previous `HasAttrs` impls from `Vec<Attribute>` and `AttrVec`
are removed - they aren't attribute targets, so the impls never really
made sense.
Use small hash set in `mir_inliner_callees`
Use small hash set in `mir_inliner_callees` to avoid temporary
allocation when possible and quadratic behaviour for large number of
callees.
Improve anonymous lifetime note to indicate the target span
Improvement for #81650
Cc #81995
Message after this improvement:
(Improve note in the middle)
```
error[E0311]: the parameter type `T` may not live long enough
--> src/main.rs:25:11
|
24 | fn play_with<T: Animal + Send>(scope: &Scope, animal: T) {
| -- help: consider adding an explicit lifetime bound...: `T: 'a +`
25 | scope.spawn(move |_| {
| ^^^^^
|
note: the parameter type `T` must be valid for the anonymous lifetime defined on the function body at 24:40...
--> src/main.rs:24:40
|
24 | fn play_with<T: Animal + Send>(scope: &Scope, animal: T) {
| ^^^^^
note: ...so that the type `[closure@src/main.rs:25:17: 27:6]` will meet its required lifetime bounds
--> src/main.rs:25:11
|
25 | scope.spawn(move |_| {
| ^^^^^
```
r? ``````@estebank``````
Replace const_cstr with cstr crate
This PR replaces the `const_cstr` macro inside `rustc_data_structures` with `cstr` macro from [cstr](https://crates.io/crates/cstr) crate.
The two macros basically serve the same purpose, which is to generate `&'static CStr` from a string literal. `cstr` is better because it validates the literal at compile time, while the existing `const_cstr` does it at runtime when `debug_assertions` is enabled. In addition, the value `cstr` generates can be used in constant context (which is seemingly not needed anywhere currently, though).
Set codegen thread names
Set names on threads spawned during codegen. Various debugging and profiling tools can take advantage of this to show a more useful identifier for threads.
For example, gdb will show thread names in `info threads`:
```
(gdb) info threads
Id Target Id Frame
1 Thread 0x7fffefa7ec40 (LWP 2905) "rustc" __pthread_clockjoin_ex (threadid=140737214134016, thread_return=0x0, clockid=<optimized out>, abstime=<optimized out>, block=<optimized out>)
at pthread_join_common.c:145
2 Thread 0x7fffefa7b700 (LWP 2957) "rustc" 0x00007ffff125eaa8 in llvm::X86_MC::initLLVMToSEHAndCVRegMapping(llvm::MCRegisterInfo*) ()
from /home/wesley/.rustup/toolchains/stage1/lib/librustc_driver-f866439e29074957.so
3 Thread 0x7fffeef0f700 (LWP 3116) "rustc" futex_wait_cancelable (private=0, expected=0, futex_word=0x7fffe8602ac8) at ../sysdeps/nptl/futex-internal.h:183
* 4 Thread 0x7fffeed0e700 (LWP 3123) "rustc" rustc_codegen_ssa:🔙:write::spawn_work (cgcx=..., work=...) at /home/wesley/code/rust/rust/compiler/rustc_codegen_ssa/src/back/write.rs:1573
6 Thread 0x7fffe113b700 (LWP 3150) "opt foof.7rcbfp" 0x00007ffff2940e62 in llvm::CallGraph::populateCallGraphNode(llvm::CallGraphNode*) ()
from /home/wesley/.rustup/toolchains/stage1/lib/librustc_driver-f866439e29074957.so
8 Thread 0x7fffe0d39700 (LWP 3158) "opt foof.7rcbfp" 0x00007fffefe8998e in malloc_consolidate (av=av@entry=0x7ffe2c000020) at malloc.c:4492
9 Thread 0x7fffe0f3a700 (LWP 3162) "opt foof.7rcbfp" 0x00007fffefef27c4 in __libc_open64 (file=0x7fffe0f38608 "foof.foof.7rcbfp3g-cgu.6.rcgu.o", oflag=524865) at ../sysdeps/unix/sysv/linux/open64.c:48
(gdb)
```
and Windows Performance Analyzer will also show this information when profiling:
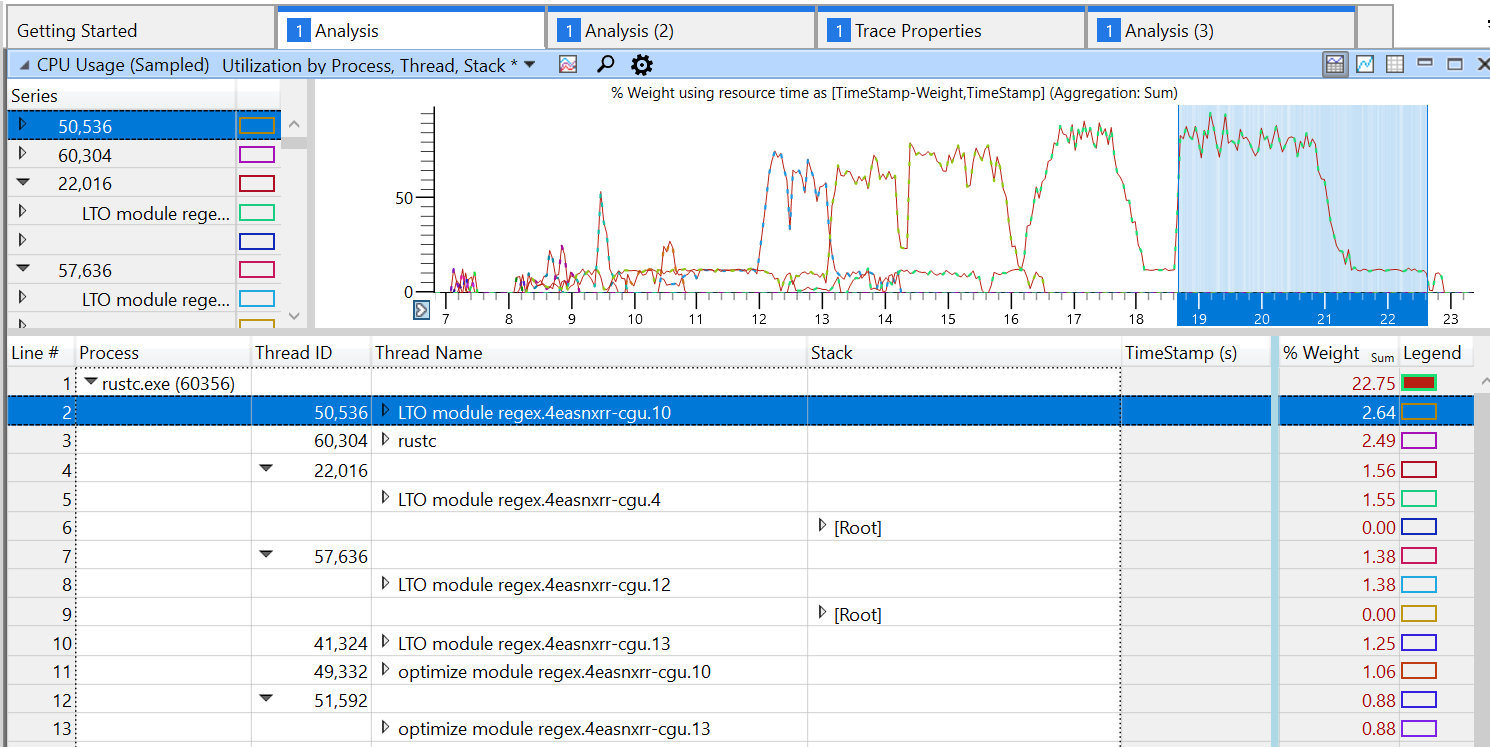
Consider inexpensive inlining criteria first
Refactor inlining decisions so that inexpensive criteria are considered first:
1. Based on code generation attributes.
2. Based on MIR availability (examines call graph).
3. Based on MIR body.
Reword labels on E0308 involving async fn return type
Fix for #80658.
When someone writes code like this:
```rust
fn foo() -> u8 {
async fn async_fn() -> () {}
async_fn()
}
```
And they try to compile it, they will see an error that looks like this:
```bash
error[E0308]: mismatched types
--> test.rs:4:5
|
1 | fn foo() -> u8 {
| -- expected `u8` because of return type
2 | async fn async_fn() -> () {}
| -- checked the `Output` of this `async fn`, found opaque type
3 |
4 | async_fn()
| ^^^^^^^^^^ expected `u8`, found opaque type
|
= note: while checking the return type of this `async fn`
= note: expected type `u8`
found opaque type `impl Future`
```
For some targets, rustc uses a "CRT fallback", where it links CRT
object files it ships instead of letting the host compiler link
them.
On musl, rustc currently links crt1, crti and crtn (provided by
libc), but does not link crtbegin and crtend (provided by libgcc).
In particular, crtend is responsible for terminating the .eh_frame
section. Lack of terminator may result in segfaults during
unwinding, as reported in #47551 and encountered by the LLVM 12
update in #81451.
This patch links crtbegin and crtend for musl as well, following
the table at the top of crt_objects.rs.
[librustdoc] Only split lang string on `,`, ` `, and `\t`
Split markdown lang strings into tokens on `,`.
The previous behavior was to split lang strings into tokens on any
character that wasn't a `_`, `_`, or alphanumeric.
This is a potentially breaking change, so please scrutinize! See discussion in #78344.
I noticed some test cases that made me wonder if there might have been some reason for the original behavior:
```
t("{.no_run .example}", false, true, Ignore::None, true, false, false, false, v(), None);
t("{.sh .should_panic}", true, false, Ignore::None, false, false, false, false, v(), None);
t("{.example .rust}", false, false, Ignore::None, true, false, false, false, v(), None);
t("{.test_harness .rust}", false, false, Ignore::None, true, true, false, false, v(), None);
```
It seemed pretty peculiar to specifically test lang strings in braces, with all the tokens prefixed by `.`.
I did some digging, and it looks like the test cases were added way back in [this commit from 2014](https://github.com/rust-lang/rust/commit/3fef7a74ca9a) by `@skade.`
It looks like they were added just to make sure that the splitting was permissive, and aren't testing that those strings in particular are accepted.
Closes https://github.com/rust-lang/rust/issues/78344.
This version adds the ability to use `rdpmc` hardware-based performance
counters instead of wall-clock time for measuring duration. This also
introduces a dependency on the `perf-event-open-sys` crate on Linux
which is used when using hardware counters.
Move pick_by_value_method docs above function header
- Currently style triggers #81183 so we can't add `#[instrument]` to
this function.
- Having docs above the header is more consistent with the rest of the
code base.
Cleanup `PpMode` and friends
This PR:
- Separates `PpSourceMode` and `PpHirMode` to remove invalid states
- Renames the variant to remove the redundant `Ppm` prefix
- Adds basic documentation for the different pretty-print modes
- Cleanups some code to make it more idiomatic
Not sure if this is actually useful, but it looks cleaner to me.
Add #[rustc_legacy_const_generics]
This is the first step towards removing `#[rustc_args_required_const]`: a new attribute is added which rewrites function calls of the form `func(a, b, c)` to `func::<{b}>(a, c)`. This allows previously stabilized functions in `stdarch` which use `rustc_args_required_const` to use const generics instead.
This new attribute is not intended to ever be stabilized, it is only intended for use in `stdarch` as a replacement for `#[rustc_args_required_const]`.
```rust
#[rustc_legacy_const_generics(1)]
pub fn foo<const Y: usize>(x: usize, z: usize) -> [usize; 3] {
[x, Y, z]
}
fn main() {
assert_eq!(foo(0 + 0, 1 + 1, 2 + 2), [0, 2, 4]);
assert_eq!(foo::<{1 + 1}>(0 + 0, 2 + 2), [0, 2, 4]);
}
```
r? `@oli-obk`
Improve error msgs when found type is deref of expected
This improves help messages in two cases:
- When expected type is `T` and found type is `&T`, we now look through blocks
and suggest dereferencing the expression of the block, rather than the whole
block.
- In the above case, if the expression is an `&`, we not suggest removing the
`&` instead of adding `*`.
Both of these are demonstrated in the regression test. Before this patch the
first error in the test would be:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:8:9
|
5 | / if true {
6 | | a
| | - expected because of this
7 | | } else {
8 | | b
| | ^ expected `usize`, found `&usize`
9 | | };
| |_____- `if` and `else` have incompatible types
|
help: consider dereferencing the borrow
|
7 | } else *{
8 | b
9 | };
|
Now:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:8:9
|
5 | / if true {
6 | | a
| | - expected because of this
7 | | } else {
8 | | b
| | ^
| | |
| | expected `usize`, found `&usize`
| | help: consider dereferencing the borrow: `*b`
9 | | };
| |_____- `if` and `else` have incompatible types
The second error:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:14:9
|
11 | / if true {
12 | | 1
| | - expected because of this
13 | | } else {
14 | | &1
| | ^^ expected integer, found `&{integer}`
15 | | };
| |_____- `if` and `else` have incompatible types
|
help: consider dereferencing the borrow
|
13 | } else *{
14 | &1
15 | };
|
now:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:14:9
|
11 | / if true {
12 | | 1
| | - expected because of this
13 | | } else {
14 | | &1
| | ^-
| | ||
| | |help: consider removing the `&`: `1`
| | expected integer, found `&{integer}`
15 | | };
| |_____- `if` and `else` have incompatible types
Fixes#82361
---
r? ````@estebank````