Currently we always do this:
```
use rustc_fluent_macro::fluent_messages;
...
fluent_messages! { "./example.ftl" }
```
But there is no need, we can just do this everywhere:
```
rustc_fluent_macro::fluent_messages! { "./example.ftl" }
```
which is shorter.
The `fluent_messages!` macro produces uses of
`crate::{D,Subd}iagnosticMessage`, which means that every crate using
the macro must have this import:
```
use rustc_errors::{DiagnosticMessage, SubdiagnosticMessage};
```
This commit changes the macro to instead use
`rustc_errors::{D,Subd}iagnosticMessage`, which avoids the need for the
imports.
Replace `option.map(cond) == Some(true)` with `option.is_some_and(cond)`
Requested by `@fmease` in https://github.com/rust-lang/rust/pull/118226#pullrequestreview-1747432292.
There is also a much larger number of `option.map_or(false, cond)` that can be changed separately if someone wants.
r? fmease
Most notably, this commit changes the `pub use crate::*;` in that file
to `use crate::*;`. This requires a lot of `use` items in other crates
to be adjusted, because everything defined within `rustc_span::*` was
also available via `rustc_span::source_map::*`, which is bizarre.
The commit also removes `SourceMap::span_to_relative_line_string`, which
is unused.
- Sort dependencies and features sections.
- Add `tidy` markers to the sorted sections so they stay sorted.
- Remove empty `[lib`] sections.
- Remove "See more keys..." comments.
Excluded files:
- rustc_codegen_{cranelift,gcc}, because they're external.
- rustc_lexer, because it has external use.
- stable_mir, because it has external use.
Stop telling people to submit bugs for internal feature ICEs
This keeps track of usage of internal features, and changes the message to instead tell them that using internal features is not supported.
I thought about several ways to do this but now used the explicit threading of an `Arc<AtomicBool>` through `Session`. This is not exactly incremental-safe, but this is fine, as this is set during macro expansion, which is pre-incremental, and also only affects the output of ICEs, at which point incremental correctness doesn't matter much anyways.
See [MCP 620.](https://github.com/rust-lang/compiler-team/issues/596)
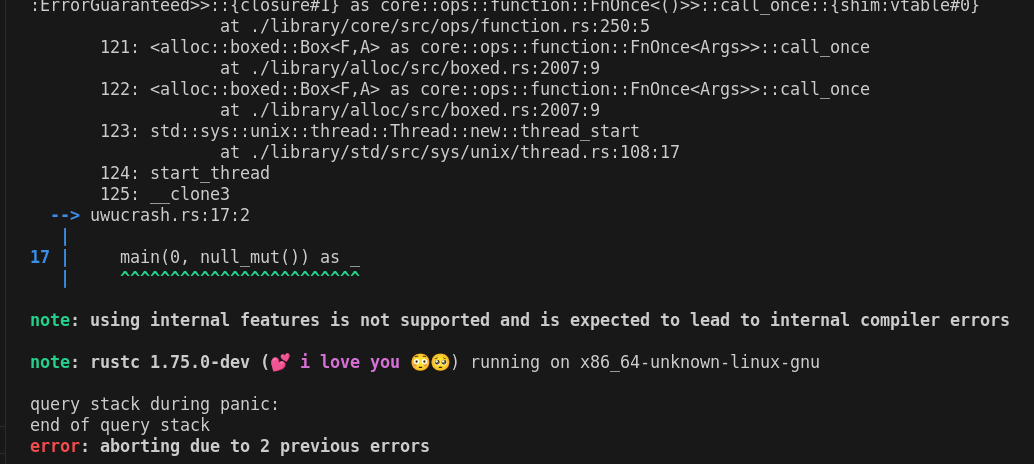
This keeps track of usage of internal features, and changes the message
to instead tell them that using internal features is not supported.
See MCP 620.
It's a better name, and lets "active features" refer to the features
that are active in a particular program, due to being declared or
enabled by the edition.
The commit also renames `Features::enabled` as `Features::active` to
match this; I changed my mind and have decided that "active" is a little
better thatn "enabled" for this, particularly because a number of
pre-existing comments use "active" in this way.
Finally, the commit renames `Status::Stable` as `Status::Accepted`, to
match `ACCEPTED_FEATURES`.
`State` is used to distinguish active vs accepted vs removed features.
However, these can also be distinguished by their location, in
`ACTIVE_FEATURES`, `ACCEPTED_FEATURES`, and `REMOVED_FEATURES`.
So this commit removes `State` and moves the internals of its variants
next to the `Feature` in each element of `*_FEATURES`, introducing new
types `ActiveFeature` and `RemovedFeature`. (There is no need for
`AcceptedFeature` because `State::Accepted` had no fields.)
This is a tighter type representation, avoids the need for some runtime
checks, and makes the code a bit shorter.
Currently `rust_20XX_preview` features aren't recorded as declared even
when they are explicit declared. Similarly, redundant edition-dependent
features (e.g. `test_2018_feature`) aren't recorded as declared.
This commit marks them as recorded. There is no detectable functional
change, but it makes things more consistent.
The word "active" is currently used in two different and confusing ways:
- `ACTIVE_FEATURES` actually means "available unstable features"
- `Features::active_features` actually means "features declared in the
crate's code", which can include feature within `ACTIVE_FEATURES` but
also others.
(This is also distinct from "enabled" features which includes declared
features but also some edition-specific features automatically enabled
depending on the edition in use.)
This commit changes the `Features::active_features` to
`Features::declared_features` which actually matches its meaning.
Likewise, `Features::active` becomes `Features::declared`.
The new way of doing things:
- Avoids some code duplication.
- Distinguishes the `crate_edition` (which comes from `--edition`) and
the `features_edition` (which combines `--edition` along with any
`rustc_20XX_preview` features), which is useful.
- Has a simpler initial loop, one that just looks for
`rustc_20XX_preview` features in order to compute `features_edition`.
- Creates a fallible alternative to `Features::enabled`, which is
useful.
It's not easy to see how exactly the old and new code are equivalent,
but it's reassuring to know that the test coverage is quite good for
this stuff.
There is a single features (`no_stack_check`) in
`STABLE_REMOVED_FEATURES`. But the treatment of
`STABLE_REMOVED_FEATURES` and `REMOVED_FEATURES` is actually identical.
So this commit just merges them, and uses a comment to record
`no_stack_check`'s unique "stable removed" status.
This also lets `State::Stabilized` (which was a terrible name) be
removed.
`Nonterminal`-related cleanups
In #114647 I am trying to remove `Nonterminal`. It has a number of preliminary cleanups that are worth merging even if #114647 doesn't merge, so let's do them in this PR.
r? `@petrochenkov`
Lots of tiny incremental simplifications of `EmitterWriter` internals
ignore the first commit, it's https://github.com/rust-lang/rust/pull/114088 squashed and rebased, but it's needed to use to use `derive_setters`, as they need a newer `syn` version.
Then this PR starts out with removing many arguments that are almost always defaulted to `None` or `false` and replace them with builder methods that can set these fields in the few cases that want to set them.
After that it's one commit after the other that removes or merges things until everything becomes some very simple trait objects
It lints against features that are inteded to be internal to the
compiler and standard library. Implements MCP #596.
We allow `internal_features` in the standard library and compiler as those
use many features and this _is_ the standard library from the "internal to the compiler and
standard library" after all.
Marking some features as internal wasn't exactly the most scientific approach, I just marked some
mostly obvious features. While there is a categorization in the macro,
it's not very well upheld (should probably be fixed in another PR).
We always pass `-Ainternal_features` in the testsuite
About 400 UI tests and several other tests use internal features.
Instead of throwing the attribute on each one, just always allow them.
There's nothing wrong with testing internal features^^
It's the same as `Delimiter`, minus the `Invisible` variant. I'm
generally in favour of using types to make impossible states
unrepresentable, but this one feels very low-value, and the conversions
between the two types are annoying and confusing.
Look at the change in `src/tools/rustfmt/src/expr.rs` for an example:
the old code converted from `MacDelimiter` to `Delimiter` and back
again, for no good reason. This suggests the author was confused about
the types.
Move doc comment desugaring out of `TokenCursor`.
It's awkward that `TokenCursor` sometimes desugars doc comments on the fly, but usually doesn't.
r? `@petrochenkov`