Suggest character encoding is incorrect when encountering random null bytes
This adds a note whenever null bytes are seen at the start of a token unexpectedly, since those tend to come from UTF-16 encoded files without a [BOM](https://en.wikipedia.org/wiki/Byte_order_mark) (if a UTF-16 BOM appears it won't be valid UTF-8, but if there is no BOM it be both valid UTF-16 and valid but garbled UTF-8). This approach was suggested in https://github.com/rust-lang/rust/issues/73979#issuecomment-653976451.
Closes#73979.
Skip Ty w/o infer ty/const in trait select
Remove some allocations & also add `skip_current_subtree` to skip subtrees with no inferred items.
r? `@eddyb` since marked in the FIXME
When token-based attribute handling is implemeneted in #80689,
we will need to access tokens from `HasAttrs` (to perform
cfg-stripping), and we will to access attributes from `HasTokens` (to
construct a `PreexpTokenStream`).
This PR merges the `HasAttrs` and `HasTokens` traits into a new
`AstLike` trait. The previous `HasAttrs` impls from `Vec<Attribute>` and `AttrVec`
are removed - they aren't attribute targets, so the impls never really
made sense.
Use small hash set in `mir_inliner_callees`
Use small hash set in `mir_inliner_callees` to avoid temporary
allocation when possible and quadratic behaviour for large number of
callees.
Improve anonymous lifetime note to indicate the target span
Improvement for #81650
Cc #81995
Message after this improvement:
(Improve note in the middle)
```
error[E0311]: the parameter type `T` may not live long enough
--> src/main.rs:25:11
|
24 | fn play_with<T: Animal + Send>(scope: &Scope, animal: T) {
| -- help: consider adding an explicit lifetime bound...: `T: 'a +`
25 | scope.spawn(move |_| {
| ^^^^^
|
note: the parameter type `T` must be valid for the anonymous lifetime defined on the function body at 24:40...
--> src/main.rs:24:40
|
24 | fn play_with<T: Animal + Send>(scope: &Scope, animal: T) {
| ^^^^^
note: ...so that the type `[closure@src/main.rs:25:17: 27:6]` will meet its required lifetime bounds
--> src/main.rs:25:11
|
25 | scope.spawn(move |_| {
| ^^^^^
```
r? ``````@estebank``````
Replace const_cstr with cstr crate
This PR replaces the `const_cstr` macro inside `rustc_data_structures` with `cstr` macro from [cstr](https://crates.io/crates/cstr) crate.
The two macros basically serve the same purpose, which is to generate `&'static CStr` from a string literal. `cstr` is better because it validates the literal at compile time, while the existing `const_cstr` does it at runtime when `debug_assertions` is enabled. In addition, the value `cstr` generates can be used in constant context (which is seemingly not needed anywhere currently, though).
Set codegen thread names
Set names on threads spawned during codegen. Various debugging and profiling tools can take advantage of this to show a more useful identifier for threads.
For example, gdb will show thread names in `info threads`:
```
(gdb) info threads
Id Target Id Frame
1 Thread 0x7fffefa7ec40 (LWP 2905) "rustc" __pthread_clockjoin_ex (threadid=140737214134016, thread_return=0x0, clockid=<optimized out>, abstime=<optimized out>, block=<optimized out>)
at pthread_join_common.c:145
2 Thread 0x7fffefa7b700 (LWP 2957) "rustc" 0x00007ffff125eaa8 in llvm::X86_MC::initLLVMToSEHAndCVRegMapping(llvm::MCRegisterInfo*) ()
from /home/wesley/.rustup/toolchains/stage1/lib/librustc_driver-f866439e29074957.so
3 Thread 0x7fffeef0f700 (LWP 3116) "rustc" futex_wait_cancelable (private=0, expected=0, futex_word=0x7fffe8602ac8) at ../sysdeps/nptl/futex-internal.h:183
* 4 Thread 0x7fffeed0e700 (LWP 3123) "rustc" rustc_codegen_ssa:🔙:write::spawn_work (cgcx=..., work=...) at /home/wesley/code/rust/rust/compiler/rustc_codegen_ssa/src/back/write.rs:1573
6 Thread 0x7fffe113b700 (LWP 3150) "opt foof.7rcbfp" 0x00007ffff2940e62 in llvm::CallGraph::populateCallGraphNode(llvm::CallGraphNode*) ()
from /home/wesley/.rustup/toolchains/stage1/lib/librustc_driver-f866439e29074957.so
8 Thread 0x7fffe0d39700 (LWP 3158) "opt foof.7rcbfp" 0x00007fffefe8998e in malloc_consolidate (av=av@entry=0x7ffe2c000020) at malloc.c:4492
9 Thread 0x7fffe0f3a700 (LWP 3162) "opt foof.7rcbfp" 0x00007fffefef27c4 in __libc_open64 (file=0x7fffe0f38608 "foof.foof.7rcbfp3g-cgu.6.rcgu.o", oflag=524865) at ../sysdeps/unix/sysv/linux/open64.c:48
(gdb)
```
and Windows Performance Analyzer will also show this information when profiling:
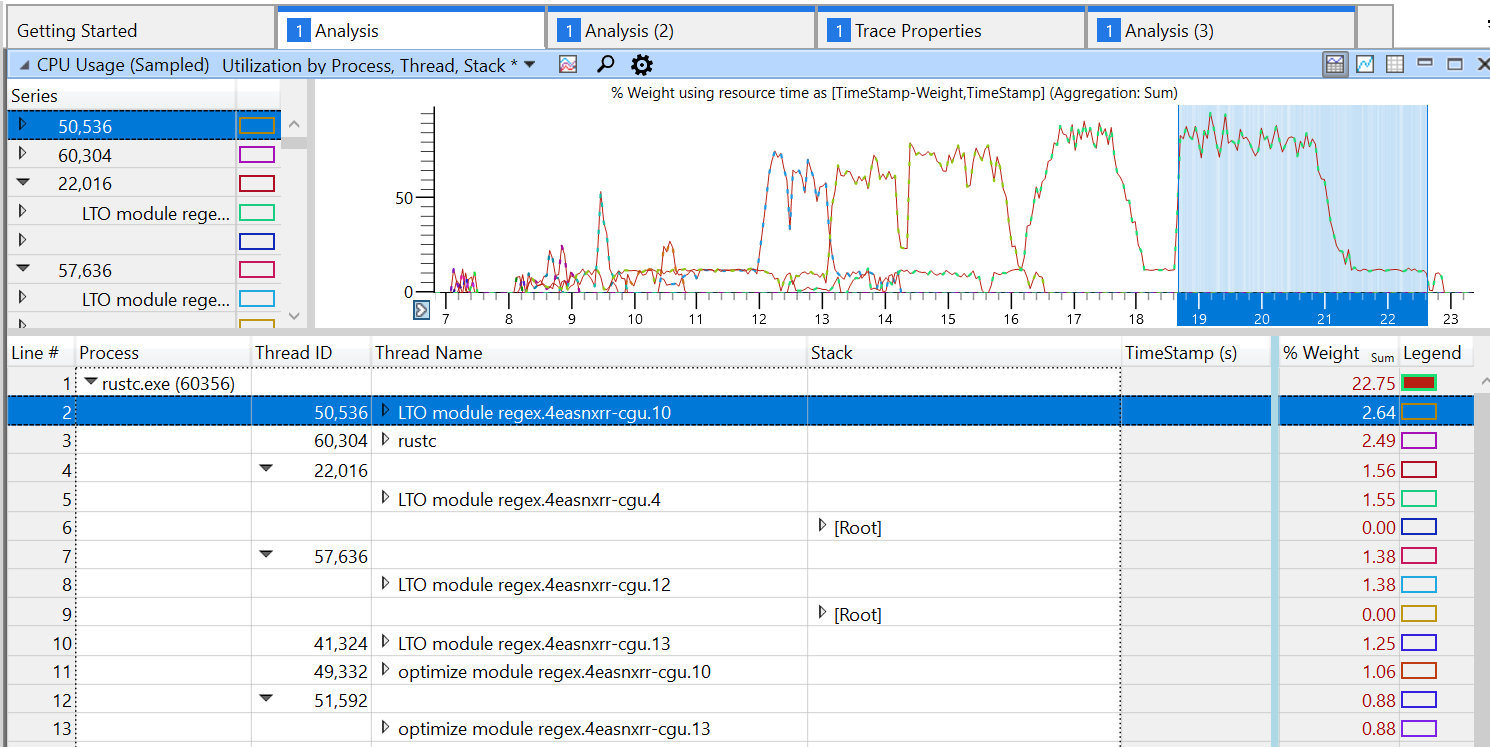
Consider inexpensive inlining criteria first
Refactor inlining decisions so that inexpensive criteria are considered first:
1. Based on code generation attributes.
2. Based on MIR availability (examines call graph).
3. Based on MIR body.
Reword labels on E0308 involving async fn return type
Fix for #80658.
When someone writes code like this:
```rust
fn foo() -> u8 {
async fn async_fn() -> () {}
async_fn()
}
```
And they try to compile it, they will see an error that looks like this:
```bash
error[E0308]: mismatched types
--> test.rs:4:5
|
1 | fn foo() -> u8 {
| -- expected `u8` because of return type
2 | async fn async_fn() -> () {}
| -- checked the `Output` of this `async fn`, found opaque type
3 |
4 | async_fn()
| ^^^^^^^^^^ expected `u8`, found opaque type
|
= note: while checking the return type of this `async fn`
= note: expected type `u8`
found opaque type `impl Future`
```
[librustdoc] Only split lang string on `,`, ` `, and `\t`
Split markdown lang strings into tokens on `,`.
The previous behavior was to split lang strings into tokens on any
character that wasn't a `_`, `_`, or alphanumeric.
This is a potentially breaking change, so please scrutinize! See discussion in #78344.
I noticed some test cases that made me wonder if there might have been some reason for the original behavior:
```
t("{.no_run .example}", false, true, Ignore::None, true, false, false, false, v(), None);
t("{.sh .should_panic}", true, false, Ignore::None, false, false, false, false, v(), None);
t("{.example .rust}", false, false, Ignore::None, true, false, false, false, v(), None);
t("{.test_harness .rust}", false, false, Ignore::None, true, true, false, false, v(), None);
```
It seemed pretty peculiar to specifically test lang strings in braces, with all the tokens prefixed by `.`.
I did some digging, and it looks like the test cases were added way back in [this commit from 2014](https://github.com/rust-lang/rust/commit/3fef7a74ca9a) by `@skade.`
It looks like they were added just to make sure that the splitting was permissive, and aren't testing that those strings in particular are accepted.
Closes https://github.com/rust-lang/rust/issues/78344.
Move pick_by_value_method docs above function header
- Currently style triggers #81183 so we can't add `#[instrument]` to
this function.
- Having docs above the header is more consistent with the rest of the
code base.
Cleanup `PpMode` and friends
This PR:
- Separates `PpSourceMode` and `PpHirMode` to remove invalid states
- Renames the variant to remove the redundant `Ppm` prefix
- Adds basic documentation for the different pretty-print modes
- Cleanups some code to make it more idiomatic
Not sure if this is actually useful, but it looks cleaner to me.
Add #[rustc_legacy_const_generics]
This is the first step towards removing `#[rustc_args_required_const]`: a new attribute is added which rewrites function calls of the form `func(a, b, c)` to `func::<{b}>(a, c)`. This allows previously stabilized functions in `stdarch` which use `rustc_args_required_const` to use const generics instead.
This new attribute is not intended to ever be stabilized, it is only intended for use in `stdarch` as a replacement for `#[rustc_args_required_const]`.
```rust
#[rustc_legacy_const_generics(1)]
pub fn foo<const Y: usize>(x: usize, z: usize) -> [usize; 3] {
[x, Y, z]
}
fn main() {
assert_eq!(foo(0 + 0, 1 + 1, 2 + 2), [0, 2, 4]);
assert_eq!(foo::<{1 + 1}>(0 + 0, 2 + 2), [0, 2, 4]);
}
```
r? `@oli-obk`
Improve error msgs when found type is deref of expected
This improves help messages in two cases:
- When expected type is `T` and found type is `&T`, we now look through blocks
and suggest dereferencing the expression of the block, rather than the whole
block.
- In the above case, if the expression is an `&`, we not suggest removing the
`&` instead of adding `*`.
Both of these are demonstrated in the regression test. Before this patch the
first error in the test would be:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:8:9
|
5 | / if true {
6 | | a
| | - expected because of this
7 | | } else {
8 | | b
| | ^ expected `usize`, found `&usize`
9 | | };
| |_____- `if` and `else` have incompatible types
|
help: consider dereferencing the borrow
|
7 | } else *{
8 | b
9 | };
|
Now:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:8:9
|
5 | / if true {
6 | | a
| | - expected because of this
7 | | } else {
8 | | b
| | ^
| | |
| | expected `usize`, found `&usize`
| | help: consider dereferencing the borrow: `*b`
9 | | };
| |_____- `if` and `else` have incompatible types
The second error:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:14:9
|
11 | / if true {
12 | | 1
| | - expected because of this
13 | | } else {
14 | | &1
| | ^^ expected integer, found `&{integer}`
15 | | };
| |_____- `if` and `else` have incompatible types
|
help: consider dereferencing the borrow
|
13 | } else *{
14 | &1
15 | };
|
now:
error[E0308]: `if` and `else` have incompatible types
--> test.rs:14:9
|
11 | / if true {
12 | | 1
| | - expected because of this
13 | | } else {
14 | | &1
| | ^-
| | ||
| | |help: consider removing the `&`: `1`
| | expected integer, found `&{integer}`
15 | | };
| |_____- `if` and `else` have incompatible types
Fixes#82361
---
r? ````@estebank````
fix the false 'defined here' messages
Closes#80853.
Take this code:
```rust
struct S;
fn repro_ref(thing: S) {
thing();
}
```
Previously, the error message would be this:
```
error[E0618]: expected function, found `S`
--> src/lib.rs:4:5
|
3 | fn repro_ref(thing: S) {
| ----- `S` defined here
4 | thing();
| ^^^^^--
| |
| call expression requires function
error: aborting due to previous error
```
This is incorrect as `S` is not defined in the function arguments, `thing` is defined there. With this change, the following is emitted:
```
error[E0618]: expected function, found `S`
--> $DIR/80853.rs:4:5
|
LL | fn repro_ref(thing: S) {
| ----- is of type `S`
LL | thing();
| ^^^^^--
| |
| call expression requires function
|
= note: local variable `S` is not a function
error: aborting due to previous error
```
As you can see, this error message points out that `thing` is of type `S` and later in a note, that `S` is not a function. This change does seem like a downside for some error messages. Take this example:
```
LL | struct Empty2;
| -------------- is of type `Empty2`
```
As you can see, the error message shows that the definition of `Empty2` is of type `Empty2`. Although this isn't wrong, it would be more helpful if it would say something like this (which was there previously):
```
LL | struct Empty2;
| -------------- `Empty2` defined here
```
If there is a better way of doing this, where the `Empty2` example would stay the same as without this change, please inform me.
**Update: This is now fixed**
CC `@camelid`
Fix ICE caused by suggestion with no code substitutions
Change suggestion logic to filter and checking _before_ creating
specific resolution suggestion.
Assert earlier that suggestions contain code substitions to make it
easier in the future to debug invalid uses. If we find this becomes too
noisy in the wild, we can always make the emitter resilient to these
cases and remove the assertions.
Fix#78651.