The new implementation doesn't use weak lang items and instead changes
`#[alloc_error_handler]` to an attribute macro just like
`#[global_allocator]`.
The attribute will generate the `__rg_oom` function which is called by
the compiler-generated `__rust_alloc_error_handler`. If no `__rg_oom`
function is defined in any crate then the compiler shim will call
`__rdl_oom` in the alloc crate which will simply panic.
This also fixes link errors with `-C link-dead-code` with
`default_alloc_error_handler`: `__rg_oom` was previously defined in the
alloc crate and would attempt to reference the `oom` lang item, even if
it didn't exist. This worked as long as `__rg_oom` was excluded from
linking since it was not called.
This is a prerequisite for the stabilization of
`default_alloc_error_handler` (#102318).
Remove redundant lifetime bound from `impl Borrow for Cow`
The lifetime bound `B::Owned: 'a` is redundant and doesn't make a difference,
because `Cow<'a, B>` comes with an implicit `B: 'a`, and associated types
will outlive lifetimes outlived by the `Self` type (and all the trait's
generic parameters, of which there are none in this case), so the implicit `B: 'a`
implies `B::Owned: 'a` anyway.
The explicit lifetime bound here does however [end up in documentation](https://doc.rust-lang.org/std/borrow/enum.Cow.html#impl-Borrow%3CB%3E),
and that's confusing in my opinion, so let's remove it ^^
_(Documentation right now, compare to `AsRef`, too:)_
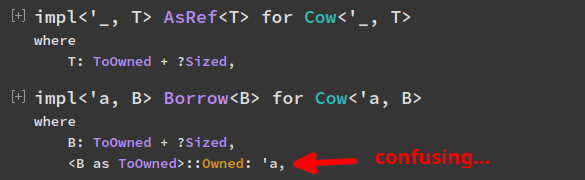
Adjust argument type for mutable with_metadata_of (#75091)
The method takes two pointer arguments: one `self` supplying the pointer value, and a second pointer supplying the metadata.
The new parameter type more clearly reflects the actual requirements. The provenance of the metadata parameter is disregarded completely. Using a mutable pointer in the call site can be coerced to a const pointer while the reverse is not true.
In some cases, the current parameter type can thus lead to a very slightly confusing additional cast. [Example](cad93775eb).
```rust
// Manually taking an unsized object from a `ManuallyDrop` into another allocation.
let val: &core::mem::ManuallyDrop<T> = …;
let ptr = val as *const _ as *mut T;
let ptr = uninit.as_ptr().with_metadata_of(ptr);
```
This could then instead be simplified to:
```rust
// Manually taking an unsized object from a `ManuallyDrop` into another allocation.
let val: &core::mem::ManuallyDrop<T> = …;
let ptr = uninit.as_ptr().with_metadata_of(&**val);
```
Tracking issue: https://github.com/rust-lang/rust/issues/75091
``@dtolnay`` you're reviewed #95249, would you mind chiming in?
Remove incorrect comment in `Vec::drain`
r? ``@scottmcm``
Turns out this comment wasn't correct for 6 years, since #34951, which switched from using `slice::IterMut` into using `slice::Iter`.
Add `Box<[T; N]>: TryFrom<Vec<T>>`
We have `[T; N]: TryFrom<Vec<T>>` (#76310) and `Box<[T; N]>: TryFrom<Box<[T]>>`, but not this combination.
`vec.into_boxed_slice().try_into()` isn't quite a replacement for this, as that'll reallocate unnecessarily in the error case.
**Insta-stable, so needs an FCP**
(I tried to make this work with `, A`, but that's disallowed because of `#[fundamental]` https://github.com/rust-lang/rust/issues/29635#issuecomment-1247598385)
Detect and reject out-of-range integers in format string literals
Until now out-of-range integers in format string literals were silently ignored. They wrapped around to zero at usize::MAX, producing unexpected results.
When using debug builds of rustc, such integers in format string literals even cause an 'attempt to add with overflow' panic in rustc.
Fix this by producing an error diagnostic for integers in format string literals which do not fit into usize.
Fixes#102528
add Vec::push_within_capacity - fallible, does not allocate
This method can serve several purposes. It
* is fallible
* guarantees that items in Vec aren't moved
* allows loops that do `reserve` and `push` separately to avoid pulling in the allocation machinery a second time in the `push` part which should make things easier on the optimizer
* eases the path towards `ArrayVec` a bit since - compared to `push()` - there are fewer questions around how it should be implemented
I haven't named it `try_push` because that should probably occupy a middle ground that will still try to reserve and only return an error in the unlikely OOM case.
resolves#84649
Currently pretty much all of the btree_map and btree_set ones fail, as
well as linked_list::DrainFilter.
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:38:5
|
38 | / require_send_sync(async {
39 | | let _v = None::<alloc::collections::btree_map::Iter<'_, &u32, &u32>>;
40 | | async {}.await;
41 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:56:5
|
56 | / require_send_sync(async {
57 | | let _v = None::<
58 | | alloc::collections::btree_map::DrainFilter<
59 | | '_,
... |
65 | | async {}.await;
66 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:68:5
|
68 | / require_send_sync(async {
69 | | let _v = None::<alloc::collections::btree_map::Entry<'_, &u32, &u32>>;
70 | | async {}.await;
71 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:88:5
|
88 | / require_send_sync(async {
89 | | let _v = None::<alloc::collections::btree_map::Iter<'_, &u32, &u32>>;
90 | | async {}.await;
91 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:93:5
|
93 | / require_send_sync(async {
94 | | let _v = None::<alloc::collections::btree_map::IterMut<'_, &u32, &u32>>;
95 | | async {}.await;
96 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:98:5
|
98 | / require_send_sync(async {
99 | | let _v = None::<alloc::collections::btree_map::Keys<'_, &u32, &u32>>;
100 | | async {}.await;
101 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:103:5
|
103 | / require_send_sync(async {
104 | | let _v = None::<alloc::collections::btree_map::OccupiedEntry<'_, &u32, &u32>>;
105 | | async {}.await;
106 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:108:5
|
108 | / require_send_sync(async {
109 | | let _v = None::<alloc::collections::btree_map::OccupiedError<'_, &u32, &u32>>;
110 | | async {}.await;
111 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:113:5
|
113 | / require_send_sync(async {
114 | | let _v = None::<alloc::collections::btree_map::Range<'_, &u32, &u32>>;
115 | | async {}.await;
116 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:118:5
|
118 | / require_send_sync(async {
119 | | let _v = None::<alloc::collections::btree_map::RangeMut<'_, &u32, &u32>>;
120 | | async {}.await;
121 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:123:5
|
123 | / require_send_sync(async {
124 | | let _v = None::<alloc::collections::btree_map::VacantEntry<'_, &u32, &u32>>;
125 | | async {}.await;
126 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:128:5
|
128 | / require_send_sync(async {
129 | | let _v = None::<alloc::collections::btree_map::Values<'_, &u32, &u32>>;
130 | | async {}.await;
131 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:133:5
|
133 | / require_send_sync(async {
134 | | let _v = None::<alloc::collections::btree_map::ValuesMut<'_, &u32, &u32>>;
135 | | async {}.await;
136 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:146:5
|
146 | / require_send_sync(async {
147 | | let _v = None::<alloc::collections::btree_set::Difference<'_, &u32>>;
148 | | async {}.await;
149 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: implementation of `Send` is not general enough
--> library/alloc/tests/autotraits.rs:151:5
|
151 | / require_send_sync(async {
152 | | let _v = None::<alloc::collections::btree_set::DrainFilter<'_, &u32, fn(&&u32) -> bool>>;
153 | | async {}.await;
154 | | });
| |______^ implementation of `Send` is not general enough
|
= note: `Send` would have to be implemented for the type `&'0 u32`, for any lifetime `'0`...
= note: ...but `Send` is actually implemented for the type `&'1 u32`, for some specific lifetime `'1`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:156:5
|
156 | / require_send_sync(async {
157 | | let _v = None::<alloc::collections::btree_set::Intersection<'_, &u32>>;
158 | | async {}.await;
159 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:166:5
|
166 | / require_send_sync(async {
167 | | let _v = None::<alloc::collections::btree_set::Iter<'_, &u32>>;
168 | | async {}.await;
169 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:171:5
|
171 | / require_send_sync(async {
172 | | let _v = None::<alloc::collections::btree_set::Range<'_, &u32>>;
173 | | async {}.await;
174 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:176:5
|
176 | / require_send_sync(async {
177 | | let _v = None::<alloc::collections::btree_set::SymmetricDifference<'_, &u32>>;
178 | | async {}.await;
179 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: higher-ranked lifetime error
--> library/alloc/tests/autotraits.rs:181:5
|
181 | / require_send_sync(async {
182 | | let _v = None::<alloc::collections::btree_set::Union<'_, &u32>>;
183 | | async {}.await;
184 | | });
| |______^
|
= note: could not prove `impl Future<Output = ()>: Send`
error: future cannot be sent between threads safely
--> library/alloc/tests/autotraits.rs:243:23
|
243 | require_send_sync(async {
| _______________________^
244 | | let _v =
245 | | None::<alloc::collections::linked_list::DrainFilter<'_, &u32, fn(&mut &u32) -> bool>>;
246 | | async {}.await;
247 | | });
| |_____^ future created by async block is not `Send`
|
= help: within `impl Future<Output = ()>`, the trait `Send` is not implemented for `NonNull<std::collections::linked_list::Node<&u32>>`
note: future is not `Send` as this value is used across an await
--> library/alloc/tests/autotraits.rs:246:17
|
244 | let _v =
| -- has type `Option<std::collections::linked_list::DrainFilter<'_, &u32, for<'a, 'b> fn(&'a mut &'b u32) -> bool>>` which is not `Send`
245 | None::<alloc::collections::linked_list::DrainFilter<'_, &u32, fn(&mut &u32) -> bool>>;
246 | async {}.await;
| ^^^^^^ await occurs here, with `_v` maybe used later
247 | });
| - `_v` is later dropped here
note: required by a bound in `require_send_sync`
--> library/alloc/tests/autotraits.rs:3:25
|
3 | fn require_send_sync<T: Send + Sync>(_: T) {}
| ^^^^ required by this bound in `require_send_sync`
error: future cannot be shared between threads safely
--> library/alloc/tests/autotraits.rs:243:23
|
243 | require_send_sync(async {
| _______________________^
244 | | let _v =
245 | | None::<alloc::collections::linked_list::DrainFilter<'_, &u32, fn(&mut &u32) -> bool>>;
246 | | async {}.await;
247 | | });
| |_____^ future created by async block is not `Sync`
|
= help: within `impl Future<Output = ()>`, the trait `Sync` is not implemented for `NonNull<std::collections::linked_list::Node<&u32>>`
note: future is not `Sync` as this value is used across an await
--> library/alloc/tests/autotraits.rs:246:17
|
244 | let _v =
| -- has type `Option<std::collections::linked_list::DrainFilter<'_, &u32, for<'a, 'b> fn(&'a mut &'b u32) -> bool>>` which is not `Sync`
245 | None::<alloc::collections::linked_list::DrainFilter<'_, &u32, fn(&mut &u32) -> bool>>;
246 | async {}.await;
| ^^^^^^ await occurs here, with `_v` maybe used later
247 | });
| - `_v` is later dropped here
note: required by a bound in `require_send_sync`
--> library/alloc/tests/autotraits.rs:3:32
|
3 | fn require_send_sync<T: Send + Sync>(_: T) {}
| ^^^^ required by this bound in `require_send_sync`
Fix in-place collection leak when remaining element destructor panic
Fixes#101628
cc `@the8472`
I went for the drop guard route, placing it immediately before the `forget_allocation_drop_remaining` call and after the comment, as to signal they are closely related.
I also updated the test to check for the leak, though the only change really needed was removing the leak clean up for miri since now that's no longer leaked.
docs: be less harsh in wording for Vec::from_raw_parts
In particular, be clear that it is sound to specify memory not
originating from a previous `Vec` allocation. That is already suggested
in other parts of the documentation about zero-alloc conversions to Box<[T]>.
Incorporate a constraint from `slice::from_raw_parts` that was missing
but needs to be fulfilled, since a `Vec` can be converted into a slice.
Fixes https://github.com/rust-lang/rust/issues/98780.
Document the conditional existence of `alloc::sync` and `alloc::task`.
`alloc` declares
```rust
#[cfg(target_has_atomic = "ptr")]
pub mod sync;
```
but there is no public documentation of this condition. This PR fixes that, so that users of `alloc` can understand how to make their code compile everywhere `alloc` does, if they are writing a library with impls for `Arc`.
The wording is copied from `std::sync::atomic::AtomicPtr`, with additional advice on how to `#[cfg]` for it.
I feel quite uncertain about whether the paragraph I added to `Arc`'s documentation should actually be there, as it is a distraction for anyone using `std`. On the other hand, maybe more reminders that no_std exists would benefit the ecosystem.
Note: `target_has_atomic` is [stabilized](https://github.com/rust-lang/rust/issues/32976) but [not yet documented in the reference](https://github.com/rust-lang/reference/pull/1171).
Make `feature(const_btree_len)` implied by `feature(const_btree_new)`
...this should fix code that used the old feature that was changed in #102197
cc ```@davidtwco``` it seems like tidy doesn't check `implied_by`, should it?
Until now out-of-range integers in format string literals
were silently ignored. They wrapped around to zero at
usize::MAX, producing unexpected results.
When using debug builds of rustc, such integers in format string
literals even cause an 'attempt to add with overflow' panic in
rustc.
Fix this by producing an error diagnostic for integers in format
string literals which do not fit into usize.
Fixes#102528
Stabilize bench_black_box
This PR stabilize `feature(bench_black_box)`.
```rust
pub fn black_box<T>(dummy: T) -> T;
```
The FCP was completed in https://github.com/rust-lang/rust/issues/64102.
`@rustbot` label +T-libs-api -T-libs
Stabilize const `BTree{Map,Set}::new`
The FCP was completed in #71835.
Since `len` and `is_empty` are not const stable yet, this also creates a new feature for them since they previously used the same `const_btree_new` feature.
Make ZST checks in core/alloc more readable
There's a bunch of these checks because of special handing for ZSTs in various unsafe implementations of stuff.
This lets them be `T::IS_ZST` instead of `mem::size_of::<T>() == 0` every time, making them both more readable and more terse.
*Not* proposed for stabilization. Would be `pub(crate)` except `alloc` wants to use it too.
(And while it doesn't matter now, if we ever get something like #85836 making it a const can help codegen be simpler.)
Since `len` and `is_empty` are not const stable yet, this also
creates a new feature for them since they previously used the same
`const_btree_new` feature.
There's a bunch of these checks because of special handing for ZSTs in various unsafe implementations of stuff.
This lets them be `T::IS_ZST` instead of `mem::size_of::<T>() == 0` every time, making them both more readable and more terse.
*Not* proposed for stabilization at this time. Would be `pub(crate)` except `alloc` wants to use it too.
(And while it doesn't matter now, if we ever get something like 85836 making it a const can help codegen be simpler.)
Make `from_waker`, `waker` and `from_raw` unstably `const`
Make
- `Context::from_waker`
- `Context::waker`
- `Waker::from_raw`
`const`.
Also added a small test.
We have `[T; N]: TryFrom<Vec<T>>` and `Box<[T; N]>: TryFrom<Box<[T]>>`, but not the combination.
`vec.into_boxed_slice().try_into()` isn't quite a replacement for this, as that'll reallocate unnecessarily in the error case.
**Insta-stable, so needs an FCP**