Refactor pre-getopts command line argument handling
Rebased version of #111658. I've also fixed the Windows CI failure (although I don't have access to Windows to test it myself).
Remove `feed_local_def_id`
best reviewed commit by commit
Basically I returned `TyCtxtFeed` from `create_def` and then preserved that in the local caches
based on https://github.com/rust-lang/rust/pull/121084
r? ````@petrochenkov````
Rework `untranslatable_diagnostic` lint
Currently it only checks calls to functions marked with `#[rustc_lint_diagnostics]`. This PR changes it to check calls to any function with an `impl Into<{D,Subd}iagnosticMessage>` parameter. This greatly improves its coverage and doesn't rely on people remembering to add `#[rustc_lint_diagnostics]`. It also lets us add `#[rustc_lint_diagnostics]` to a number of functions that don't have an `impl Into<{D,Subd}iagnosticMessage>`, such as `Diag::span`.
r? ``@davidtwco``
Currently it only checks calls to functions marked with
`#[rustc_lint_diagnostics]`. This commit changes it to check calls to
any function with an `impl Into<{D,Subd}iagMessage>` parameter. This
greatly improves its coverage and doesn't rely on people remembering to
add `#[rustc_lint_diagnostics]`.
The commit also adds `#[allow(rustc::untranslatable_diagnostic)`]
attributes to places that need it that are caught by the improved lint.
These places that might be easy to convert to translatable diagnostics.
Finally, it also:
- Expands and corrects some comments.
- Does some minor formatting improvements.
- Adds missing `DecorateLint` cases to
`tests/ui-fulldeps/internal-lints/diagnostics.rs`.
Hint user to update nightly on ICEs produced from outdated nightly
This is a conservative best-effort approach to detect a potentially outdated nightly; it will fallback to the regular ICE-reporting if any of the following cases are true:
- Channel is not nightly
- Version information is not available
- Version date is not parseable as a YYYY-MM-DD or is missing
- System time is at least 36 hours ahead of the user's nightly release datetime.
- Any internal features are used.
Note that I'm not sure how to make a test for this: I tested this manually by `CFG_VER_DATE="2020-02-02" ./x build library --stage 1`, and also changing the channel detection in `rustc_driver_impl` from `Some("nightly")` to `Some("nightly" | "dev")`, and then running `rustc +stage1 test.rs -Ztreat-err-as-bug=1` with a non-existent `test.rs`.
<img width="1145" alt="Screenshot 2024-02-27 at 01 12 28" src="https://github.com/rust-lang/rust/assets/39484203/eff6af2e-4b19-4a70-af57-cd739ecf0e84">
Closes#118832.
Existing names for values of this type are `sess`, `parse_sess`,
`parse_session`, and `ps`. `sess` is particularly annoying because
that's also used for `Session` values, which are often co-located, and
it can be difficult to know which type a value named `sess` refers to.
(That annoyance is the main motivation for this change.) `psess` is nice
and short, which is good for a name used this much.
The commit also renames some `parse_sess_created` values as
`psess_created`.
Invert diagnostic lints.
That is, change `diagnostic_outside_of_impl` and `untranslatable_diagnostic` from `allow` to `deny`, because more than half of the compiler has been converted to use translated diagnostics.
This commit removes more `deny` attributes than it adds `allow` attributes, which proves that this change is warranted.
r? ````@davidtwco````
When `catch_fatal_errors` catches a `FatalErrorMarker`, it returns an
`ErrorGuaranteed` that is conjured out of thin air with
`unchecked_claim_error_was_emitted`. But that `ErrorGuaranteed` is never
used.
This commit changes it to instead conjure a `FatalError` out of thin
air. (A non-deprecated action!) This makes more sense because
`FatalError` and `FatalErrorMarker` are a natural pairing -- a
`FatalErrorMarker` is created by calling `FatalError::raise`, so this is
effectively getting back the original `FatalError`.
This requires a tiny change in `catch_with_exit_code`. The old result of
the `catch_fatal_errors` call there was
`Result<Result<(), ErrorGuaranteed>, ErrorGuaranteed>` which could be
`flatten`ed into `Result<(), ErrorGuaranteed>`. The new result of the
`catch_fatal_errors` calls is
`Result<Result<(), ErrorGuaranteed>, FatalError>`, which can't be
`flatten`ed but is still easily matched for the success case.
That is, change `diagnostic_outside_of_impl` and
`untranslatable_diagnostic` from `allow` to `deny`, because more than
half of the compiler has be converted to use translated diagnostics.
This commit removes more `deny` attributes than it adds `allow`
attributes, which proves that this change is warranted.
Error codes are integers, but `String` is used everywhere to represent
them. Gross!
This commit introduces `ErrCode`, an integral newtype for error codes,
replacing `String`. It also introduces a constant for every error code,
e.g. `E0123`, and removes the `error_code!` macro. The constants are
imported wherever used with `use rustc_errors::codes::*`.
With the old code, we have three different ways to specify an error code
at a use point:
```
error_code!(E0123) // macro call
struct_span_code_err!(dcx, span, E0123, "msg"); // bare ident arg to macro call
\#[diag(name, code = "E0123")] // string
struct Diag;
```
With the new code, they all use the `E0123` constant.
```
E0123 // constant
struct_span_code_err!(dcx, span, E0123, "msg"); // constant
\#[diag(name, code = E0123)] // constant
struct Diag;
```
The commit also changes the structure of the error code definitions:
- `rustc_error_codes` now just defines a higher-order macro listing the
used error codes and nothing else.
- Because that's now the only thing in the `rustc_error_codes` crate, I
moved it into the `lib.rs` file and removed the `error_codes.rs` file.
- `rustc_errors` uses that macro to define everything, e.g. the error
code constants and the `DIAGNOSTIC_TABLES`. This is in its new
`codes.rs` file.
This works for most of its call sites. This is nice, because `emit` very
much makes sense as a consuming operation -- indeed,
`DiagnosticBuilderState` exists to ensure no diagnostic is emitted
twice, but it uses runtime checks.
For the small number of call sites where a consuming emit doesn't work,
the commit adds `DiagnosticBuilder::emit_without_consuming`. (This will
be removed in subsequent commits.)
Likewise, `emit_unless` becomes consuming. And `delay_as_bug` becomes
consuming, while `delay_as_bug_without_consuming` is added (which will
also be removed in subsequent commits.)
All this requires significant changes to `DiagnosticBuilder`'s chaining
methods. Currently `DiagnosticBuilder` method chaining uses a
non-consuming `&mut self -> &mut Self` style, which allows chaining to
be used when the chain ends in `emit()`, like so:
```
struct_err(msg).span(span).emit();
```
But it doesn't work when producing a `DiagnosticBuilder` value,
requiring this:
```
let mut err = self.struct_err(msg);
err.span(span);
err
```
This style of chaining won't work with consuming `emit` though. For
that, we need to use to a `self -> Self` style. That also would allow
`DiagnosticBuilder` production to be chained, e.g.:
```
self.struct_err(msg).span(span)
```
However, removing the `&mut self -> &mut Self` style would require that
individual modifications of a `DiagnosticBuilder` go from this:
```
err.span(span);
```
to this:
```
err = err.span(span);
```
There are *many* such places. I have a high tolerance for tedious
refactorings, but even I gave up after a long time trying to convert
them all.
Instead, this commit has it both ways: the existing `&mut self -> Self`
chaining methods are kept, and new `self -> Self` chaining methods are
added, all of which have a `_mv` suffix (short for "move"). Changes to
the existing `forward!` macro lets this happen with very little
additional boilerplate code. I chose to add the suffix to the new
chaining methods rather than the existing ones, because the number of
changes required is much smaller that way.
This doubled chainging is a bit clumsy, but I think it is worthwhile
because it allows a *lot* of good things to subsequently happen. In this
commit, there are many `mut` qualifiers removed in places where
diagnostics are emitted without being modified. In subsequent commits:
- chaining can be used more, making the code more concise;
- more use of chaining also permits the removal of redundant diagnostic
APIs like `struct_err_with_code`, which can be replaced easily with
`struct_err` + `code_mv`;
- `emit_without_diagnostic` can be removed, which simplifies a lot of
machinery, removing the need for `DiagnosticBuilderState`.
rework `-Zverbose`
implements the changes described in https://github.com/rust-lang/compiler-team/issues/706
the first commit is only a name change from `-Zverbose` to `-Zverbose-internals` and does not change behavior. the second commit changes diagnostics.
possible follow up work:
- `ty::pretty` could print more info with `--verbose` than it does currently. `-Z verbose-internals` shows too much info in a way that's not helpful to users. michael had ideas about this i didn't fully understand: https://rust-lang.zulipchat.com/#narrow/stream/233931-t-compiler.2Fmajor-changes/topic/uplift.20some.20-Zverbose.20calls.20and.20rename.20to.E2.80.A6.20compiler-team.23706/near/408984200
- `--verbose` should imply `-Z write-long-types-to-disk=no`. the code in `ty_string_with_limit` should take `--verbose` into account (apparently this affects `Ty::sort_string`, i'm not familiar with this code). writing a file to disk should suggest passing `--verbose`.
r? `@compiler-errors` cc `@estebank`
Instead of allowing `rustc::potential_query_instability` on the whole
crate we go over each lint and allow it individually if it is safe to
do. Turns out there were no instances of this lint in this crate.
They're not used in `rustc_session`, and `rustc_metadata` is a more
obvious location.
`MetadataLoader` was originally put into `rustc_session` in #41565 to
avoid a dependency on LLVM, but things have changed a lot since then and
that's no longer relevant, e.g. `rustc_codegen_llvm` depends on
`rustc_metadata`.
Currently we always do this:
```
use rustc_fluent_macro::fluent_messages;
...
fluent_messages! { "./example.ftl" }
```
But there is no need, we can just do this everywhere:
```
rustc_fluent_macro::fluent_messages! { "./example.ftl" }
```
which is shorter.
The `fluent_messages!` macro produces uses of
`crate::{D,Subd}iagnosticMessage`, which means that every crate using
the macro must have this import:
```
use rustc_errors::{DiagnosticMessage, SubdiagnosticMessage};
```
This commit changes the macro to instead use
`rustc_errors::{D,Subd}iagnosticMessage`, which avoids the need for the
imports.
Emit smir
This adds ability to `-Zunpretty=smir` and get smir output of a Rust file, this is obliviously pretty basic compared to `mir` output but I think we could iteratively improve it, and even at this state this is useful for us.
r? ``@celinval``
I find `Compilation::and_then` hard to read. This commit removes it,
simplifying the control flow in `run_compiler`, and reducing the number
of lines of code.
In particular, `list_metadata` and `process_try_link` (renamed `rlink`)
are now only called if the relevant condition is true, rather than that
condition being checked within the function.
Currently we have an inconsistency between the "input" and "no input"
cases:
- no input: `rustc --print=sysroot -Whelp` prints the lint help.
- input: `rustc --print=sysroot -Whelp a.rs` prints the sysroot.
It makes sense to print the lint help in both cases, because that's what
happens with `--help`/`-Zhelp`/`-Chelp`.
In fact, the `describe_lints` in the "input" case happens amazingly
late, after *parsing*. This is because, with plugins, lints used to be
registered much later, when the global context was created. But #117649
moved lint registration much earlier, during session construction.
So this commit moves the `describe_lints` call to a single spot for both
for both the "input" and "no input" cases, as early as possible. This is
still not as early as `--help`/`-Zhelp`/`-Chelp`, because `-Whelp` must
wait until the session is constructed.
`rustc_driver_impl::run_compiler` currently has two
`interface::run_compiler` calls: one for the "no input" case, and one
for the normal case.
This commit merges the former into the latter, which makes the control
flow easier to read and avoids some duplication.
It also makes it clearer that the "no input" case will describe lints
before printing crate info, while the normal case does it in the reverse
order. Possibly a bug?
Yes, its type is `EarlyErrorHandler`, but there is another value of that
type later on in the function called `handler` that is initialized with
`sopts.error_format`. So `default_handler` is a better name because it
clarifies that it is initialized with `ErrorOutputType::default()`.
This was made possible by the removal of plugin support, which
simplified lint store creation.
This simplifies the places in rustc and rustdoc that call
`describe_lints`, which are early on. The lint store is now built before
those places, so they don't have to create their own lint store for
temporary use, they can just use the main one.
rustc_log: provide a way to init logging based on the values, not names, of the env vars
Miri wants to affect how rustc does logging. So far this required setting environment variables before calling `rustc_driver::init_rustc_env_logger`. However, `set_var` is a function one should really [avoid calling](https://github.com/rust-lang/rust/issues/90308), so this adds the necessary APIs to rustc such that Miri can just pass it the *values* of all the log-relevant environment variables, rather than having to change the global environment.
The included measurements have varied over the years. At one point there
were quite a few more, but #49558 deleted a lot that were no longer
used. Today there's just four, and it's a motley collection that doesn't
seem particularly valuable.
I think it has been well and truly subsumed by self-profiling, which
collects way more data.
They've been deprecated for four years.
This commit includes the following changes.
- It eliminates the `rustc_plugin_impl` crate.
- It changes the language used for lints in
`compiler/rustc_driver_impl/src/lib.rs` and
`compiler/rustc_lint/src/context.rs`. External lints are now called
"loaded" lints, rather than "plugins" to avoid confusion with the old
plugins. This only has a tiny effect on the output of `-W help`.
- E0457 and E0498 are no longer used.
- E0463 is narrowed, now only relating to unfound crates, not plugins.
- The `plugin` feature was moved from "active" to "removed".
- It removes the entire plugins chapter from the unstable book.
- It removes quite a few tests, mostly all of those in
`tests/ui-fulldeps/plugin/`.
Closes#29597.
Most notably, this commit changes the `pub use crate::*;` in that file
to `use crate::*;`. This requires a lot of `use` items in other crates
to be adjusted, because everything defined within `rustc_span::*` was
also available via `rustc_span::source_map::*`, which is bizarre.
The commit also removes `SourceMap::span_to_relative_line_string`, which
is unused.
By storing the unparsed values in `Config` and then parsing them within
`run_compiler`, the parsing functions can use the main symbol interner,
and not create their own short-lived interners.
This change also eliminates the need for one `EarlyErrorHandler` in
rustdoc, because parsing errors can be reported by another, slightly
later `EarlyErrorHandler`.
- Sort dependencies and features sections.
- Add `tidy` markers to the sorted sections so they stay sorted.
- Remove empty `[lib`] sections.
- Remove "See more keys..." comments.
Excluded files:
- rustc_codegen_{cranelift,gcc}, because they're external.
- rustc_lexer, because it has external use.
- stable_mir, because it has external use.
`parse_cfgspecs` and `parse_check_cfg` run very early, before the main
interner is running. They each use a short-lived interner and convert
all interned symbols to strings in their output data structures. Once
the main interner starts up, these data structures get converted into
new data structures that are identical except with the strings converted
to symbols.
All is not obvious from the current code, which is a mess, particularly
with inconsistent naming that obscures the parallel string/symbol data
structures. This commit clean things up a lot.
- The existing `CheckCfg` type is generic, allowing both
`CheckCfg<String>` and `CheckCfg<Symbol>` forms. This is really
useful, but it defaults to `String`. The commit removes the default so
we have to use `CheckCfg<String>` and `CheckCfg<Symbol>` explicitly,
which makes things clearer.
- Introduces `Cfg`, which is generic over `String` and `Symbol`, similar
to `CheckCfg`.
- Renames some things.
- `parse_cfgspecs` -> `parse_cfg`
- `CfgSpecs` -> `Cfg<String>`, plus it's used in more places, rather
than the underlying `FxHashSet` type.
- `CrateConfig` -> `Cfg<Symbol>`.
- `CrateCheckConfig` -> `CheckCfg<Symbol>`
- Adds some comments explaining the string-to-symbol conversions.
- `to_crate_check_config`, which converts `CheckCfg<String>` to
`CheckCfg<Symbol>`, is inlined and removed and combined with the
overly-general `CheckCfg::map_data` to produce
`CheckCfg::<String>::intern`.
- `build_configuration` now does the `Cfg<String>`-to-`Cfg<Symbol>`
conversion, so callers don't need to, which removes the need for
`to_crate_config`.
The diff for two of the fields in `Config` is a good example of the
improved clarity:
```
- pub crate_cfg: FxHashSet<(String, Option<String>)>,
- pub crate_check_cfg: CheckCfg,
+ pub crate_cfg: Cfg<String>,
+ pub crate_check_cfg: CheckCfg<String>,
```
Compare that with the diff for the corresponding fields in `ParseSess`,
and the relationship to `Config` is much clearer than before:
```
- pub config: CrateConfig,
- pub check_config: CrateCheckConfig,
+ pub config: Cfg<Symbol>,
+ pub check_config: CheckCfg<Symbol>,
```
Remove `rustc_symbol_mangling/messages.ftl`.
It contains a single message that (a) doesn't contain any natural language, and (b) is only used in tests.
r? `@davidtwco`
Stop telling people to submit bugs for internal feature ICEs
This keeps track of usage of internal features, and changes the message to instead tell them that using internal features is not supported.
I thought about several ways to do this but now used the explicit threading of an `Arc<AtomicBool>` through `Session`. This is not exactly incremental-safe, but this is fine, as this is set during macro expansion, which is pre-incremental, and also only affects the output of ICEs, at which point incremental correctness doesn't matter much anyways.
See [MCP 620.](https://github.com/rust-lang/compiler-team/issues/596)
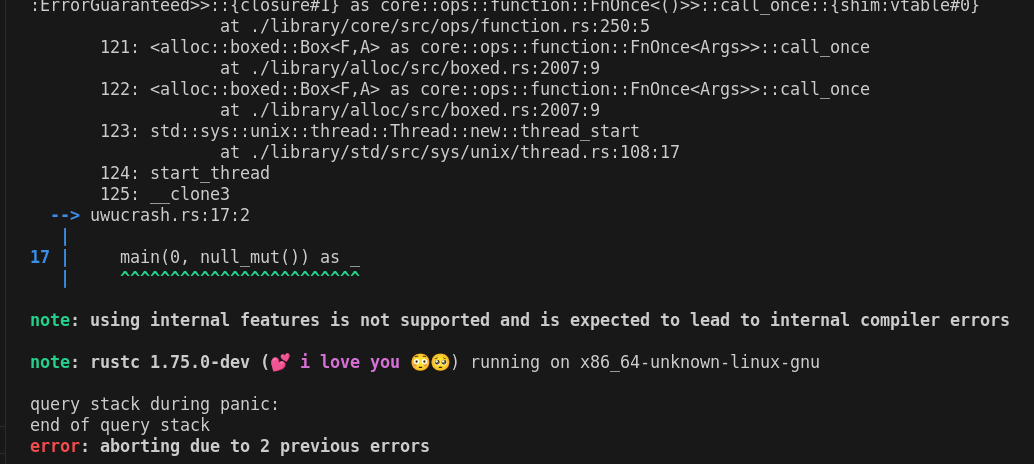
This keeps track of usage of internal features, and changes the message
to instead tell them that using internal features is not supported.
See MCP 620.
Use `YYYY-MM-DDTHH_MM_SS` as datetime format for ICE dump files
Windows paths do not support `:`, so use a datetime format in ICE dump paths that Windows will accept.
CC #116809, fix#115180.
Use tidy to enforce alphabetical dependency ordering
I get annoyed when dependencies in `Cargo.toml` files are not in alphabetical order. The [style guide](https://github.com/rust-lang/rust/blob/master/src/doc/style-guide/src/cargo.md) agrees with me.
There are ongoing efforts to provide linting/formatting of `Cargo.toml` files, e.g. https://github.com/rust-lang/rustfmt/pull/5240, https://crates.io/crates/cargo-toml-lint, and https://github.com/TimonPost/cargo-toml-format. But it's far from clear what's the right approach.
So this PR does something very simple: it uses the order checking already present in tidy. This allows incremental application of ordering, starting right now, and avoiding the need for any kind of all-at-once conversion.
If we do end up using some more comprehensive `Cargo.toml` linting/formatting solution in the future, the `tidy-alphabetical` lines will be easy to remove.
r? `@wesleywiser`
Add `Config::hash_untracked_state` callback
For context, I'm looking to use [late module passes](https://doc.rust-lang.org/nightly/nightly-rustc/rustc_lint/context/struct.LintStore.html#structfield.late_module_passes) in Clippy which unlike regular late passes run incrementally per module
However we have a config file which can change between runs, we need changes to that to invalidate the `lint_mod` query. This PR adds a side channel for us to hash some extra state into `Options` in order to do that
This does not make any changes to Clippy, I plan to do that in a PR to the Clippy repo along with some other required changes
An alternative implementation would be to add a new query to track this state and override the `lint_mod` query in Clippy to first call that
cc `@rust-lang/clippy`
`NoAnn` and `IdentifiedAnnotation` impl both `pprust_ast::PpAnn` and
`pprust_hir::PpAnn`, which is a bit confusing, because the optional
`tcx` is only needed for the HIR cases. (Currently the `tcx` is
unnecessarily provided in the `expanded` AST cases.)
This commit splits each one into `Ast` and `Hir` versions, which makes
things clear about where the `tcx` is needed. The commit also renames
all the traits so they consistently end with `Ann`.
`call_with_pp_support_ast` and `call_with_pp_support_hir` how each have
a single call site. This commit inlines and removes them, which also
removes the need for all the supporting traits: `Sess`,
`AstPrinterSupport`, and `HirPrinterSupport`. The `sess` member is also
removed from several structs.
The handling of the `PpMode` variants is currently spread across three
functions: `print_after_parsing`, `print_after_hir_lowering`, and
`print_with_analysis`. Each one handles some of the variants. This split
is primarily because `print_after_parsing` has slightly different
arguments to the other two.
This commit changes the structure. It merges the three functions into a
single `print` function, and encapsulates the different arguments in a
new enum `PrintExtra`.
Benefits:
- The code is a little shorter.
- All the `PpMode` variants are handled in a single `match`, with no
need for `unreachable!` arms.
- It enables the trait removal in the subsequent commit by reducing
the number of `call_with_pp_support_ast` call sites from two to one.
First, both `AstPrinterSupport` and `HirPrinterSupport` have a `sess`
method. This commit introduces a `Sess` trait and makes the support
traits be subtraits of `Sess`, to avoid some duplication.
Second, both support traits have a `pp_ann` method that isn't needed if
we enable `trait_upcasting`. This commit removes those methods.
(Both of these traits will be removed in a subsequent commit, as will
the `trait_upcasting` use.)
`phase_3_run_analysis_passes` no longer exists, and AFAICT this code has
been refactored so much since this comment was written that it no longer
has any useful meaning.
Use `std::path::PathBuf` rather than `String`; use `std::env::var_os`
rather than `std::env::var`. These changes avoid a number of error paths
which can arise in the presence of non-UTF-8 paths.
Don't modify libstd to dump rustc ICEs
Do a much simpler thing and just dump a `std::backtrace::Backtrace` to file.
r? `@estebank` `@oli-obk`
Fixes#115610
Make SIGSEGV handler emit nicer backtraces
This annotates the code heavily with comments to explain what is going on, for the benefit of other compiler contributors. The backtrace also emits appropriate comments to clarify, to a programmer who may not know why a bunch of file paths and hexadecimal blather was just dumped into stderr, what is going on. Finally, it detects cycles and uses their regularity to avoid repeating a bunch of text. The previous backtraces we were emitting was extremely unfriendly, potentially confusing, and often alarming, and this makes things almost "nice".
We can't necessarily make them much nicer than this, because a signal handler must use "signal-safe" functions. This precludes conveniences like dynamic allocations. Fortunately, Rust's stdlib has allocation-free formatting, but it may hinder integrating this error with our localization middleware, as I wasn't able to clearly ascertain, at a glance, whether there was a zero-alloc path through it.
r? `@Nilstrieb`
Always add LC_BUILD_VERSION for metadata object files
As of Xcode 15 Apple's linker has become a bit more strict about the warnings it produces. One of those new warnings requires all valid Mach-O object files in an archive to have a LC_BUILD_VERSION load command:
```
ld: warning: no platform load command found in 'ARCHIVE[arm64][2106](lib.rmeta)', assuming: iOS-simulator
```
This was already being done for Mac Catalyst so this change expands this logic to include it for all Apple platforms. I filed this behavior change as FB12546320 and was told it was the new intentional behavior.
As of Xcode 15 Apple's linker has become a bit more strict about the
warnings it produces. One of those new warnings requires all valid
Mach-O object files in an archive to have a LC_BUILD_VERSION load
command:
```
ld: warning: no platform load command found in 'ARCHIVE[arm64][2106](lib.rmeta)', assuming: iOS-simulator
```
This was already being done for Mac Catalyst so this change expands this
logic to include it for all Apple platforms. I filed this behavior
change as FB12546320 and was told it was the new intentional behavior.
Fix missing dependency file with `-Zunpretty`
This PR force the `output_filenames` to be run ~~in every early exits like~~ when using `-Zunpretty`, so to respect the `dep-info` flag.
Fixes https://github.com/rust-lang/rust/issues/112898
r? `@oli-obk`
Support `--print KIND=PATH` command line syntax
As is already done for `--emit KIND=PATH` and `-L KIND=PATH`.
In the discussion of #110785, it was pointed out that `--print KIND=PATH` is nicer than trying to apply the single global `-o` path to `--print`'s output, because in general there can be multiple print requests within a single rustc invocation, and anyway `-o` would already be used for a different meaning in the case of `link-args` and `native-static-libs`.
I am interested in using `--print cfg=PATH` in Buck2. Currently Buck2 works around the lack of support for `--print KIND=PATH` by [indirecting through a Python wrapper script](d43cf3a51a/prelude/rust/tools/get_rustc_cfg.py) to redirect rustc's stdout into the location dictated by the build system.
From skimming Cargo's usages of `--print`, it definitely seems like it would benefit from `--print KIND=PATH` too. Currently it is working around the lack of this by inserting `--crate-name=___ --print=crate-name` so that it can look for a line containing `___` as a delimiter between the 2 other `--print` informations it actually cares about. This is commented as a "HACK" and "abuse". 31eda6f7c3/src/cargo/core/compiler/build_context/target_info.rs (L242) (FYI `@weihanglo` as you dealt with this recently in https://github.com/rust-lang/cargo/pull/11633.)
Mentioning reviewers active in #110785: `@fee1-dead` `@jyn514` `@bjorn3`
Implement rust-lang/compiler-team#578.
When an ICE is encountered on nightly releases, the new rustc panic
handler will also write the contents of the backtrace to disk. If any
`delay_span_bug`s are encountered, their backtrace is also added to the
file. The platform and rustc version will also be collected.
First, we reuse the `MAX_FRAMES` constant.
Co-authored-by: erikdesjardins <erikdesjardins@users.noreply.github.com>
Then we choose an arbitrary recursion depth for
the other case. In this case, I used 2d20. Honest.
This cleans up the formatting of the backtrace considerably,
dodging the fact that a backtrace with recursion normally
emits hundreds of lines. Instead, simply write repeated symbols,
and add how many times the recursion occurred.
Also, it makes it look more like the "normal" backtrace.
Some fine details in the code are important for reducing
the amount of possible panic branches.
...to both compiler contributors and other rustc invokers.
The previous error messaging was extremely unfriendly,
and potentially both confusing and alarming.
The entire modules is extracted into a new file to help
ease of reading, and plenty of comments get layered in.
The design of the messaging is focused on preventing
the overall purpose of the output from being too opaque
in common cases, so users understand why it is there.
There's not an immediate need for it being actionable,
but some suggestions are offered anyways.
Previously, forgetting to call `interface::set_thread_safe_mode` would cause the following ICE:
```
thread 'rustc' panicked at 'uninitialized dyn_thread_safe mode!', /rustc/dfe0683138de0959b6ab6a039b54d9347f6a6355/compiler/rustc_data_structures/src/sync.rs:74:18
```
This calls `set_thread_safe_mode` in `interface::run_compiler` to avoid requiring it in the caller.
Fixes `tests/run-make-fulldeps/issue-19371` when parallel-compiler is enabled.
rustc installs a signal stack that assumes that
MINSIGSTKSZ is a constant, unchanging value.
Newer hardware undermines that assumption greatly,
with register files larger than MINSIGSTKZ.
Properly handle this so that it is correct on
all supported Linux versions with all CPUs.
Currently, the output of `rustc --explain foo` displays the raw markdown in a
pager. This is acceptable, but using actual formatting makes it easier to
understand.
This patch consists of three major components:
1. A markdown parser. This is an extremely simple non-backtracking recursive
implementation that requires normalization of the final token stream
2. A utility to write the token stream to an output buffer
3. Configuration within rustc_driver_impl to invoke this combination for
`--explain`. Like the current implementation, it first attempts to print to
a pager with a fallback colorized terminal, and standard print as a last
resort.
If color is disabled, or if the output does not support it, or if printing
with color fails, it will write the raw markdown (which matches current
behavior).
Pagers known to support color are: `less` (with `-r`), `bat` (aka `catbat`),
and `delta`.
The markdown parser does not support the entire markdown specification, but
should support the following with reasonable accuracy:
- Headings, including formatting
- Comments
- Code, inline and fenced block (no indented block)
- Strong, emphasis, and strikethrough formatted text
- Links, anchor, inline, and reference-style
- Horizontal rules
- Unordered and ordered list items, including formatting
This parser and writer should be reusable by other systems if ever needed.
Because `Lrc<Box<T>>` is silly. (Clippy warns about `Rc<Box<T>>` and
`Arc<Box<T>>`, and it would warn here if (a) we used Clippy with rustc,
and (b) Clippy knew about `Lrc`.)