mirror of
https://github.com/EmbarkStudios/rust-gpu.git
synced 2024-12-18 11:35:06 +00:00
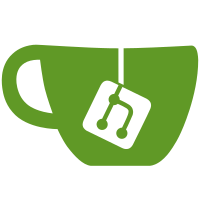
* Accept `#[rust_gpu::spirv()]` attributes rather than `#[spirv()]` in backend * Implemented `#[spirv(..)]` proc macro attribute for all platforms that conditionally translates to `#[rust_gpu::spirv()]` based on platform * Changed `SpirvBuilder` to always apply `register_tool(rust_gpu)` attribute to shader crates * Updated docs * Added changelog
51 lines
781 B
Rust
51 lines
781 B
Rust
// build-pass
|
|
|
|
use spirv_std::spirv;
|
|
|
|
use glam::*;
|
|
|
|
fn sphere_sdf(p: Vec3) -> f32 {
|
|
p.length() - 1.0
|
|
}
|
|
|
|
// Global scene to render
|
|
fn scene_sdf(p: Vec3) -> f32 {
|
|
sphere_sdf(p)
|
|
}
|
|
|
|
fn render(eye: Vec3, dir: Vec3, start: f32, end: f32) -> f32 {
|
|
let max_marching_steps: i32 = 255;
|
|
let epsilon: f32 = 0.0001;
|
|
|
|
let mut depth = start;
|
|
let mut i = 0;
|
|
|
|
loop {
|
|
if i < max_marching_steps {
|
|
break;
|
|
}
|
|
|
|
let dist = scene_sdf(eye + depth * dir);
|
|
|
|
if dist < epsilon {
|
|
return depth;
|
|
}
|
|
|
|
depth += dist;
|
|
|
|
if depth >= end {
|
|
return end;
|
|
}
|
|
|
|
i += 1;
|
|
}
|
|
|
|
end
|
|
}
|
|
|
|
#[spirv(fragment)]
|
|
pub fn main() {
|
|
let v = Vec3::new(1.0, 1.0, 1.0);
|
|
render(v, v, 1.0, 2.0);
|
|
}
|