mirror of
https://github.com/NixOS/nixpkgs.git
synced 2024-12-03 20:33:21 +00:00
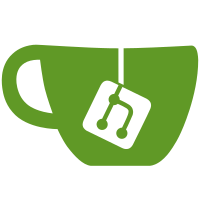
The flag iterates through the lockfile entries, rewrites `resolved` URLs to those that will be in the cache (like `fixup_yarn_lock` from yarn2nix), removes `integrity` for git deps whose hash won't match the reproducible repacking that the fetcher does, writes the amended lockfile, and exits.
75 lines
1.7 KiB
JavaScript
Executable File
75 lines
1.7 KiB
JavaScript
Executable File
#!/usr/bin/env node
|
|
'use strict'
|
|
|
|
const fs = require('fs')
|
|
const process = require('process')
|
|
const lockfile = require('./yarnpkg-lockfile.js')
|
|
const { urlToName } = require('./common.js')
|
|
|
|
const fixupYarnLock = async (lockContents, verbose) => {
|
|
const lockData = lockfile.parse(lockContents)
|
|
|
|
const fixedData = Object.fromEntries(
|
|
Object.entries(lockData.object)
|
|
.map(([dep, pkg]) => {
|
|
const [ url, hash ] = pkg.resolved.split("#", 2)
|
|
|
|
if (hash || url.startsWith("https://codeload.github.com")) {
|
|
if (verbose) console.log(`Removing integrity for git dependency ${dep}`)
|
|
delete pkg.integrity
|
|
}
|
|
|
|
if (verbose) console.log(`Rewriting URL ${url} for dependency ${dep}`)
|
|
pkg.resolved = urlToName(url)
|
|
|
|
return [dep, pkg]
|
|
})
|
|
)
|
|
|
|
if (verbose) console.log('Done')
|
|
|
|
return fixedData
|
|
}
|
|
|
|
const showUsage = async () => {
|
|
process.stderr.write(`
|
|
syntax: fixup-yarn-lock [path to yarn.lock] [options]
|
|
|
|
Options:
|
|
-h --help Show this help
|
|
-v --verbose Verbose output
|
|
`)
|
|
process.exit(1)
|
|
}
|
|
|
|
const main = async () => {
|
|
const args = process.argv.slice(2)
|
|
let next, lockFile, verbose
|
|
while (next = args.shift()) {
|
|
if (next == '--verbose' || next == '-v') {
|
|
verbose = true
|
|
} else if (next == '--help' || next == '-h') {
|
|
showUsage()
|
|
} else if (!lockFile) {
|
|
lockFile = next
|
|
} else {
|
|
showUsage()
|
|
}
|
|
}
|
|
let lockContents
|
|
try {
|
|
lockContents = await fs.promises.readFile(lockFile || 'yarn.lock', 'utf-8')
|
|
} catch {
|
|
showUsage()
|
|
}
|
|
|
|
const fixedData = await fixupYarnLock(lockContents, verbose)
|
|
await fs.promises.writeFile(lockFile || 'yarn.lock', lockfile.stringify(fixedData))
|
|
}
|
|
|
|
main()
|
|
.catch(e => {
|
|
console.error(e)
|
|
process.exit(1)
|
|
})
|