mirror of
https://github.com/embassy-rs/embassy.git
synced 2024-11-21 22:32:29 +00:00
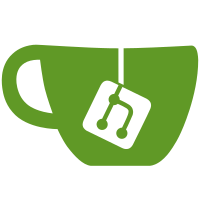
Spin polls the raw executor and never sleeps. It is useful for disabling any power features associated with wfi/wfe-like instructions. When implementing support for the CH32V30x MCU, the wfi instruction had issues interacting with the USB OTG peripheral and appeared to be non-spec-compliant. 1. When sending a USB Data-in packet, the USB peripheral appears to be unable to read the system main memory while in WFI. This manifests in the USB peripheral sending all or partially zeroed DATA packets. Disabling WFI works around this issue. 2. The WFI instruction does not wake up the processor when MIE is disabled. The MCU provides a WFITOWFE bit to emulate the WFE instruction on arm, which, when enabled, ignores the MIE and allows the processor to wake up. This works around the non-compliant WFI implementation. Co-authored-by: Codetector <codetector@codetector.org> Co-authored-by: Dummyc0m <y@types.moe>
189 lines
5.1 KiB
TOML
189 lines
5.1 KiB
TOML
[package]
|
||
name = "embassy-executor"
|
||
version = "0.6.0"
|
||
edition = "2021"
|
||
license = "MIT OR Apache-2.0"
|
||
description = "async/await executor designed for embedded usage"
|
||
repository = "https://github.com/embassy-rs/embassy"
|
||
documentation = "https://docs.embassy.dev/embassy-executor"
|
||
categories = [
|
||
"embedded",
|
||
"no-std",
|
||
"asynchronous",
|
||
]
|
||
|
||
[package.metadata.embassy_docs]
|
||
src_base = "https://github.com/embassy-rs/embassy/blob/embassy-executor-v$VERSION/embassy-executor/src/"
|
||
src_base_git = "https://github.com/embassy-rs/embassy/blob/$COMMIT/embassy-executor/src/"
|
||
features = ["defmt"]
|
||
flavors = [
|
||
{ name = "std", target = "x86_64-unknown-linux-gnu", features = ["arch-std", "executor-thread"] },
|
||
{ name = "wasm", target = "wasm32-unknown-unknown", features = ["arch-wasm", "executor-thread"] },
|
||
{ name = "cortex-m", target = "thumbv7em-none-eabi", features = ["arch-cortex-m", "executor-thread", "executor-interrupt"] },
|
||
{ name = "riscv32", target = "riscv32imac-unknown-none-elf", features = ["arch-riscv32", "executor-thread"] },
|
||
]
|
||
|
||
[package.metadata.docs.rs]
|
||
default-target = "thumbv7em-none-eabi"
|
||
targets = ["thumbv7em-none-eabi"]
|
||
features = ["defmt", "arch-cortex-m", "executor-thread", "executor-interrupt"]
|
||
|
||
[dependencies]
|
||
defmt = { version = "0.3", optional = true }
|
||
log = { version = "0.4.14", optional = true }
|
||
rtos-trace = { version = "0.1.2", optional = true }
|
||
|
||
embassy-executor-macros = { version = "0.5.0", path = "../embassy-executor-macros" }
|
||
embassy-time-driver = { version = "0.1.0", path = "../embassy-time-driver", optional = true }
|
||
embassy-time-queue-driver = { version = "0.1.0", path = "../embassy-time-queue-driver", optional = true }
|
||
critical-section = "1.1"
|
||
|
||
document-features = "0.2.7"
|
||
|
||
# needed for AVR
|
||
portable-atomic = { version = "1.5", optional = true }
|
||
|
||
# arch-cortex-m dependencies
|
||
cortex-m = { version = "0.7.6", optional = true }
|
||
|
||
# arch-wasm dependencies
|
||
wasm-bindgen = { version = "0.2.82", optional = true }
|
||
js-sys = { version = "0.3", optional = true }
|
||
|
||
# arch-avr dependencies
|
||
avr-device = { version = "0.5.3", optional = true }
|
||
|
||
[dev-dependencies]
|
||
critical-section = { version = "1.1", features = ["std"] }
|
||
|
||
|
||
[features]
|
||
|
||
## Enable nightly-only features
|
||
nightly = ["embassy-executor-macros/nightly"]
|
||
|
||
# Enables turbo wakers, which requires patching core. Not surfaced in the docs by default due to
|
||
# being an complicated advanced and undocumented feature.
|
||
# See: https://github.com/embassy-rs/embassy/pull/1263
|
||
turbowakers = []
|
||
|
||
## Use the executor-integrated `embassy-time` timer queue.
|
||
integrated-timers = ["dep:embassy-time-driver", "dep:embassy-time-queue-driver"]
|
||
|
||
#! ### Architecture
|
||
_arch = [] # some arch was picked
|
||
## std
|
||
arch-std = ["_arch", "critical-section/std"]
|
||
## Cortex-M
|
||
arch-cortex-m = ["_arch", "dep:cortex-m"]
|
||
## RISC-V 32
|
||
arch-riscv32 = ["_arch"]
|
||
## WASM
|
||
arch-wasm = ["_arch", "dep:wasm-bindgen", "dep:js-sys", "critical-section/std"]
|
||
## AVR
|
||
arch-avr = ["_arch", "dep:portable-atomic", "dep:avr-device"]
|
||
## spin (architecture agnostic; never sleeps)
|
||
arch-spin = ["_arch"]
|
||
|
||
#! ### Executor
|
||
|
||
## Enable the thread-mode executor (using WFE/SEV in Cortex-M, WFI in other embedded archs)
|
||
executor-thread = []
|
||
## Enable the interrupt-mode executor (available in Cortex-M only)
|
||
executor-interrupt = []
|
||
|
||
#! ### Task Arena Size
|
||
#! Sets the [task arena](#task-arena) size. Necessary if you’re not using `nightly`.
|
||
#!
|
||
#! <details>
|
||
#! <summary>Preconfigured Task Arena Sizes:</summary>
|
||
#! <!-- rustdoc requires the following blank line for the feature list to render correctly! -->
|
||
#!
|
||
|
||
# BEGIN AUTOGENERATED CONFIG FEATURES
|
||
# Generated by gen_config.py. DO NOT EDIT.
|
||
## 64
|
||
task-arena-size-64 = []
|
||
## 128
|
||
task-arena-size-128 = []
|
||
## 192
|
||
task-arena-size-192 = []
|
||
## 256
|
||
task-arena-size-256 = []
|
||
## 320
|
||
task-arena-size-320 = []
|
||
## 384
|
||
task-arena-size-384 = []
|
||
## 512
|
||
task-arena-size-512 = []
|
||
## 640
|
||
task-arena-size-640 = []
|
||
## 768
|
||
task-arena-size-768 = []
|
||
## 1024
|
||
task-arena-size-1024 = []
|
||
## 1280
|
||
task-arena-size-1280 = []
|
||
## 1536
|
||
task-arena-size-1536 = []
|
||
## 2048
|
||
task-arena-size-2048 = []
|
||
## 2560
|
||
task-arena-size-2560 = []
|
||
## 3072
|
||
task-arena-size-3072 = []
|
||
## 4096 (default)
|
||
task-arena-size-4096 = [] # Default
|
||
## 5120
|
||
task-arena-size-5120 = []
|
||
## 6144
|
||
task-arena-size-6144 = []
|
||
## 8192
|
||
task-arena-size-8192 = []
|
||
## 10240
|
||
task-arena-size-10240 = []
|
||
## 12288
|
||
task-arena-size-12288 = []
|
||
## 16384
|
||
task-arena-size-16384 = []
|
||
## 20480
|
||
task-arena-size-20480 = []
|
||
## 24576
|
||
task-arena-size-24576 = []
|
||
## 32768
|
||
task-arena-size-32768 = []
|
||
## 40960
|
||
task-arena-size-40960 = []
|
||
## 49152
|
||
task-arena-size-49152 = []
|
||
## 65536
|
||
task-arena-size-65536 = []
|
||
## 81920
|
||
task-arena-size-81920 = []
|
||
## 98304
|
||
task-arena-size-98304 = []
|
||
## 131072
|
||
task-arena-size-131072 = []
|
||
## 163840
|
||
task-arena-size-163840 = []
|
||
## 196608
|
||
task-arena-size-196608 = []
|
||
## 262144
|
||
task-arena-size-262144 = []
|
||
## 327680
|
||
task-arena-size-327680 = []
|
||
## 393216
|
||
task-arena-size-393216 = []
|
||
## 524288
|
||
task-arena-size-524288 = []
|
||
## 655360
|
||
task-arena-size-655360 = []
|
||
## 786432
|
||
task-arena-size-786432 = []
|
||
## 1048576
|
||
task-arena-size-1048576 = []
|
||
|
||
# END AUTOGENERATED CONFIG FEATURES
|
||
|
||
#! </details>
|