mirror of
https://github.com/Lokathor/bytemuck.git
synced 2024-11-25 00:02:22 +00:00
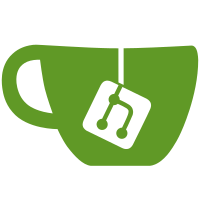
* Implement `ByteEq` and `ByteHash` derives This adds the derives `ByteEq` and `ByteHash` that can be used as an alternative to the `Eq` / `PartialEq` and `Hash` derives from the standard library. The difference is that these variants use `bytemuck` to convert their values to byte slices before comparing / hashing them. This allows the comparisons to turn into a simple `memcmp` / `bcmp` (or completely inlined as a few vector instructions) and allows hashers to process all bytes at once, possibly allowing for some vector operations as well. Here's a quick comparison of the generated assembly:  * Address review comments
26 lines
441 B
Rust
26 lines
441 B
Rust
#![cfg(feature = "derive")]
|
|
#![allow(dead_code)]
|
|
|
|
use bytemuck::{ByteEq, ByteHash, Pod, TransparentWrapper, Zeroable};
|
|
|
|
#[derive(Copy, Clone, Pod, Zeroable, ByteEq, ByteHash)]
|
|
#[repr(C)]
|
|
struct Test {
|
|
a: u16,
|
|
b: u16,
|
|
}
|
|
|
|
#[derive(TransparentWrapper)]
|
|
#[repr(transparent)]
|
|
struct TransparentSingle {
|
|
a: u16,
|
|
}
|
|
|
|
#[derive(TransparentWrapper)]
|
|
#[repr(transparent)]
|
|
#[transparent(u16)]
|
|
struct TransparentWithZeroSized {
|
|
a: u16,
|
|
b: (),
|
|
}
|