mirror of
https://github.com/Lokathor/bytemuck.git
synced 2024-11-21 22:32:23 +00:00
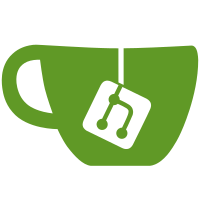
* update rustfmt.toml replace `merge_imports = true` (deprecated) with `imports_granularity = "Crate"` * run `cargo +nightly fmt` * rewrite docs and rename `Wrapped` to `Inner` rewriting some docs for conciseness rename `Wrapped` to `Inner`, because it's hard to visually differentiate between `Wrapper` and `Wrapped` * impl missing `TransparentWrapper::wrap_{}` fns Implement 3 new wrapping functions on `TransparentWrapper` providing new conversions. - `TransparentWrapper::wrap(s: Inner) -> Self` - `TransparentWrapper::wrap_slice(s: &[Inner]) -> &[Self]` - `TransparentWrapper::wrap_slice_mut(s: &mut [Inner]) -> &mut [Self]` * impl `TransparentWrapper::unwrap_{}` fns Implement counterparts to `TransparentWrapper::wrap_{}` functions providing reverse conversions. - `TransparentWrapper::unwrap(self) -> Inner` - `TransparentWrapper::unwrap_ref(&self) -> &Inner` - `TransparentWrapper::unwrap_mut(&mut self) -> &mut Inner` - `TransparentWrapper::unwrap_slice(s: &[Self]) -> &[Inner]` - `TransparentWrapper::unwrap_slice_mut(s: &mut [Self]) -> &mut [Inner]` * add `TransparentWrapper` UB test This test is only for MIRI to check all trait functions on `TransparentWrapper` if they cause any UB. The output of the functions is not checked. * small `TransparentWrapper` doc adjustments * change fn signature on `TransparentWrapper` Methods on `TransparentWrapper` trait are now associated functions. They now take `Self` instead of `self` as argument) - `TransparentWrapper::unwrap(s: Self)` - `TransparentWrapper::unwrap_ref(s: &Self)` - `TransparentWrapper::unwrap_mut(s: &mut Self)`
65 lines
2.1 KiB
Rust
65 lines
2.1 KiB
Rust
// Currently this test doesn't actually check the output of the functions.
|
|
// It's only here for miri to check for any potential undefined behaviour.
|
|
// TODO: check function results
|
|
|
|
#[test]
|
|
fn test_transparent_wrapper() {
|
|
// An external type defined in a different crate.
|
|
#[derive(Copy, Clone, Default)]
|
|
struct Foreign(u8);
|
|
|
|
use bytemuck::TransparentWrapper;
|
|
|
|
#[derive(Copy, Clone)]
|
|
#[repr(transparent)]
|
|
struct Wrapper(Foreign);
|
|
|
|
unsafe impl TransparentWrapper<Foreign> for Wrapper {}
|
|
|
|
// Traits can be implemented on crate-local wrapper.
|
|
unsafe impl bytemuck::Zeroable for Wrapper {}
|
|
unsafe impl bytemuck::Pod for Wrapper {}
|
|
|
|
let _: u8 = bytemuck::cast(Wrapper::wrap(Foreign::default()));
|
|
let _: Foreign = Wrapper::unwrap(bytemuck::cast(u8::default()));
|
|
|
|
let _: &u8 = bytemuck::cast_ref(Wrapper::wrap_ref(&Foreign::default()));
|
|
let _: &Foreign = Wrapper::unwrap_ref(bytemuck::cast_ref(&u8::default()));
|
|
|
|
let _: &mut u8 =
|
|
bytemuck::cast_mut(Wrapper::wrap_mut(&mut Foreign::default()));
|
|
let _: &mut Foreign =
|
|
Wrapper::unwrap_mut(bytemuck::cast_mut(&mut u8::default()));
|
|
|
|
let _: &[u8] =
|
|
bytemuck::cast_slice(Wrapper::wrap_slice(&[Foreign::default()]));
|
|
let _: &[Foreign] =
|
|
Wrapper::unwrap_slice(bytemuck::cast_slice(&[u8::default()]));
|
|
|
|
let _: &mut [u8] =
|
|
bytemuck::cast_slice_mut(Wrapper::wrap_slice_mut(
|
|
&mut [Foreign::default()],
|
|
));
|
|
let _: &mut [Foreign] =
|
|
Wrapper::unwrap_slice_mut(bytemuck::cast_slice_mut(&mut [u8::default()]));
|
|
|
|
let _: &[u8] = bytemuck::bytes_of(Wrapper::wrap_ref(&Foreign::default()));
|
|
let _: &Foreign = Wrapper::unwrap_ref(bytemuck::from_bytes(&[u8::default()]));
|
|
|
|
let _: &mut [u8] =
|
|
bytemuck::bytes_of_mut(Wrapper::wrap_mut(&mut Foreign::default()));
|
|
let _: &mut Foreign =
|
|
Wrapper::unwrap_mut(bytemuck::from_bytes_mut(&mut [u8::default()]));
|
|
|
|
// not sure if this is the right usage
|
|
let _ =
|
|
bytemuck::pod_align_to::<_, u8>(Wrapper::wrap_slice(&[Foreign::default()]));
|
|
// counterpart?
|
|
|
|
// not sure if this is the right usage
|
|
let _ = bytemuck::pod_align_to_mut::<_, u8>(Wrapper::wrap_slice_mut(&mut [
|
|
Foreign::default(),
|
|
]));
|
|
// counterpart?
|
|
}
|