mirror of
https://github.com/Lokathor/bytemuck.git
synced 2024-11-21 22:32:23 +00:00
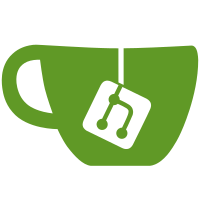
- new feature `nightly_docs` enables `doc_cfg` unstable feature that allows showing explicit dependencies on features. - new cargo alias `nightly_docs` for a more convenient local documentation build `cargo +nightly nightly_docs --open`. - annotate exported items and implementations depending on some feature with the corresponding `doc(cfg())` attribute. - add the `nightly_docs` feature to the `[package.metadata.docs.rs]` section.
36 lines
1.3 KiB
Rust
36 lines
1.3 KiB
Rust
use super::*;
|
|
|
|
// Note(Lokathor): This is the neat part!!
|
|
unsafe impl<T: ZeroableInOption> Zeroable for Option<T> {}
|
|
|
|
/// Trait for types which are [Zeroable](Zeroable) when wrapped in
|
|
/// [Option](core::option::Option).
|
|
///
|
|
/// ## Safety
|
|
///
|
|
/// * `Option<YourType>` must uphold the same invariants as
|
|
/// [Zeroable](Zeroable).
|
|
pub unsafe trait ZeroableInOption: Sized {}
|
|
|
|
unsafe impl ZeroableInOption for NonZeroI8 {}
|
|
unsafe impl ZeroableInOption for NonZeroI16 {}
|
|
unsafe impl ZeroableInOption for NonZeroI32 {}
|
|
unsafe impl ZeroableInOption for NonZeroI64 {}
|
|
unsafe impl ZeroableInOption for NonZeroI128 {}
|
|
unsafe impl ZeroableInOption for NonZeroIsize {}
|
|
unsafe impl ZeroableInOption for NonZeroU8 {}
|
|
unsafe impl ZeroableInOption for NonZeroU16 {}
|
|
unsafe impl ZeroableInOption for NonZeroU32 {}
|
|
unsafe impl ZeroableInOption for NonZeroU64 {}
|
|
unsafe impl ZeroableInOption for NonZeroU128 {}
|
|
unsafe impl ZeroableInOption for NonZeroUsize {}
|
|
|
|
// Note: this does not create NULL vtable because we get `None` anyway.
|
|
unsafe impl<T: ?Sized> ZeroableInOption for NonNull<T> {}
|
|
unsafe impl<T: ?Sized> ZeroableInOption for &'_ T {}
|
|
unsafe impl<T: ?Sized> ZeroableInOption for &'_ mut T {}
|
|
|
|
#[cfg(feature = "extern_crate_alloc")]
|
|
#[cfg_attr(feature = "nightly_docs", doc(cfg(feature = "extern_crate_alloc")))]
|
|
unsafe impl<T: ?Sized> ZeroableInOption for alloc::boxed::Box<T> {}
|